How to Switch on Enum in Java
- Switch on Enum Using Traditional Switch and Case in Java
- Switch on Enum Using the Enhanced Switch and Case in Java 12
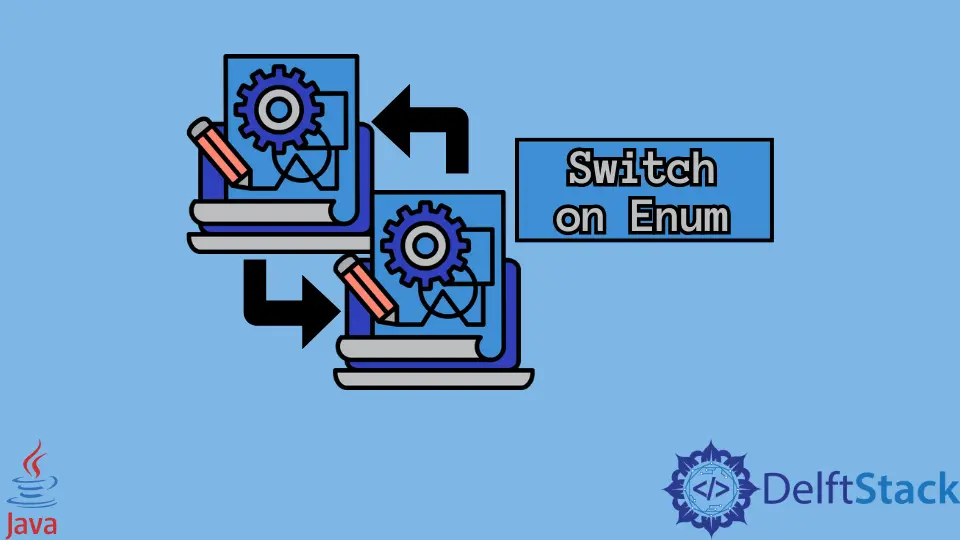
This article explains how to use switch
on enum in Java. We will go through two ways to use the switch
statement with enum.
Switch on Enum Using Traditional Switch and Case in Java
In the example, we create an enum inside the SwitchEnum
class and name it Days
. It holds seven constants that are the days of a week. We use the switch and case method to show a different message for each day.
We get the value from the enum using the constant’s name like Days.MONDAY
will fetch the constant MONDAY
, and it will be stored in the enum object day
. We can use it to switch between cases. switch()
takes in the value to switch, that is day
. At last, we specify each case and the output that it should produce.
We have to break every case in the switch so that it breaks the execution once it is finished.
public class SwitchEnum {
enum Days { SUNDAY, MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY }
public static void main(String[] args) {
Days day = Days.MONDAY;
switch (day) {
case SUNDAY:
System.out.println("Sundays are wonderful");
break;
case MONDAY:
System.out.println("Mondays are boring");
break;
case TUESDAY:
System.out.println("Tuesdays are OK");
break;
case WEDNESDAY:
System.out.println("Wednesdays are tiring");
break;
case THURSDAY:
System.out.println("Thursdays are even more boring");
break;
case FRIDAY:
System.out.println("Fridays means work work and work");
break;
case SATURDAY:
System.out.println("Saturdays makes everybody happy");
break;
}
}
}
Output:
Mondays are boring
Switch on Enum Using the Enhanced Switch and Case in Java 12
In Java 12, the enhanced switch and case were introduced to overcome the disadvantages of the traditional switch and case. The biggest downfall of the traditional switch statements is that we have to specify the break keyword in every case.
Now with the enhanced switch and case, we can use the enum with shorter codes. We use arrows instead of colons in the new switch and case. As we want to print only one statement, we do not have to use any curly braces.
public class SwitchEnum {
enum Days { SUNDAY, MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY }
public static void main(String[] args) {
Days day = Days.SATURDAY;
switch (day) {
case SUNDAY -> System.out.println("Sundays are wonderful");
case MONDAY -> System.out.println("Mondays are boring");
case TUESDAY -> System.out.println("Tuesdays are OK");
case WEDNESDAY -> System.out.println("Wednesdays are tiring");
case THURSDAY -> System.out.println("Thursdays are even more boring");
case FRIDAY -> System.out.println("Fridays means work work and work");
case SATURDAY -> System.out.println("Saturdays makes everybody happy");
}
}
}
Output:
Saturdays makes everybody happy
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedInRelated Article - Java Switch
- How to Use Multiple Values for One Switch Case Statement in Java
- How to Switch Multiple Case in Java
- Java switch Statement on Strings