How to Use Multiple Values for One Switch Case Statement in Java
-
Use Two or More Values for One
switch-case
Statement -
Use the Arrow Syntax to Use Multiple Values for One
switch-case
Statement - Conclusion
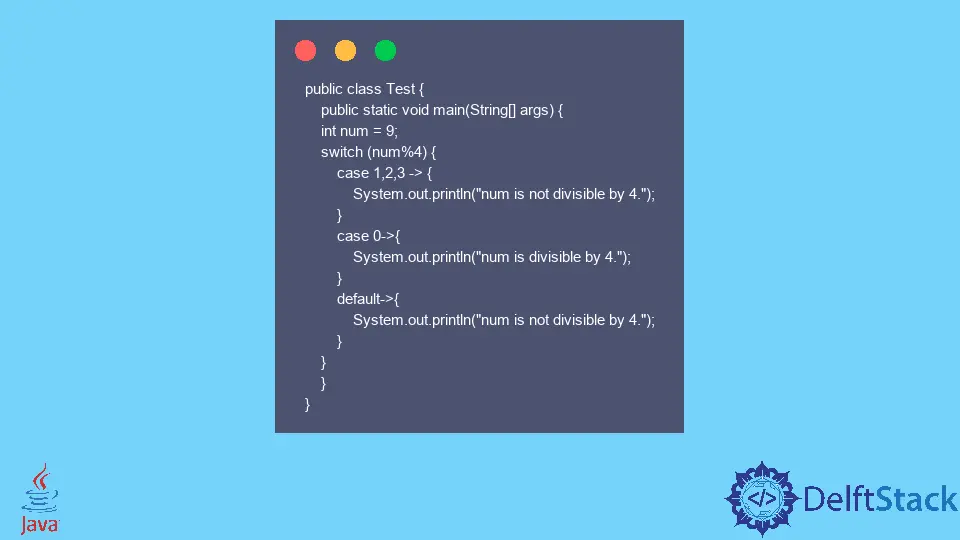
In Java programming, the switch
statement is a versatile tool for managing multiple conditions. However, traditional implementations often involve redundancy when handling the same code for multiple values.
This article explores two methods to address this challenge. First, the Multiple Case Labels
method streamlines decision-making by grouping values without separate code blocks.
Then, the Arrow Syntax
method, introduced in Java 14, offers a concise alternative. These techniques enhance code clarity and efficiency, particularly when dealing with scenarios like categorizing days of the week. Let’s delve into these methods to simplify code and improve readability.
Use Two or More Values for One switch-case
Statement
The multiple case labels method provides an elegant solution to this challenge. This section will explore how this method enhances code clarity and efficiency by handling multiple values within a switch
statement.
Imagine a scenario where you want to categorize days of the week based on their position. Let’s use this method to achieve this without duplicating code blocks.
public class DayOfWeekClassifier {
public static void main(String[] args) {
int day = 4; // Assume 1 represents Monday, 7 represents Sunday
switch (day) {
case 1:
case 2:
case 3:
case 4:
case 5:
System.out.println("Weekday");
break;
case 6:
case 7:
System.out.println("Weekend");
break;
default:
System.out.println("Invalid day");
}
}
}
We initialize an int
variable named day
with a value of 4
. The subsequent switch
statement evaluates the value of day
.
Cases 1
, 2
, 3
, 4
, and 5
are listed sequentially without individual code blocks. When the variable day
matches any of these cases, the code block immediately following the cases is executed.
Notice that there is no need for explicit break
statements between these cases, allowing the control to flow through to the common code block.
The common code block, following the weekday cases, contains the logic to be executed when day
represents a weekday. The absence of a break
statement facilitates the flow through to this common code block, minimizing redundancy and improving code organization.
Subsequently, cases 6
and 7
are listed together, each with its own code block, specifying the behavior for when day
represents a weekend.
The optional default
case acts as a catch-all, executing its code block if none of the specified cases match the value of the variable.
Output:
Weekday
Use the Arrow Syntax to Use Multiple Values for One switch-case
Statement
Java 14 introduces a new syntax for the switch-case
statement. Users can add multiple values for a single case
by separating the comma, and users have to put the executable code in the curly braces.
In this section, we’ll explore the significance of arrow syntax and demonstrate how it simplifies code, making it more readable and efficient.
Consider a situation where you want to categorize days of the week based on their position. The arrow syntax method allows you to achieve this in a concise manner.
public class DayOfWeekClassifier {
public static void main(String[] args) {
int day = 4; // Assume 1 represents Monday, 7 represents Sunday
switch (day) {
case 1, 2, 3, 4, 5 -> System.out.println("Weekday");
case 6, 7 -> System.out.println("Weekend");
default -> System.out.println("Invalid day");
}
}
}
We initialize an int
variable named day
with a value of 4
. The subsequent switch
statement evaluates the value of day
.
The first case
line uses arrow syntax to group values 1
, 2
, 3
, 4
, and 5
together. This eliminates the need for redundant code blocks, and the arrow (->
) succinctly associates this group of values with the subsequent code block.
Similarly, the second case
line groups values 6
and 7
together using arrow syntax, providing a clean and expressive way to handle multiple values within a single line.
The default
case serves as a catch-all, executing its code block if none of the specified cases match the value of the variable.
Output:
Weekday
Conclusion
Handling multiple values within a switch
statement has evolved. The Multiple Case Labels
and Arrow Syntax
methods provide elegant solutions, reducing redundancy and enhancing code readability.
The first method groups cases without separate blocks, allowing for seamless control flow. On the other hand, the arrow syntax in the second method condenses multiple values into a single line, offering a clean and expressive alternative.
Whether streamlining decision-making or embracing the latest syntax, these methods contribute to cleaner, more efficient code. As you navigate the intricacies of Java, consider incorporating these techniques for a more streamlined development experience.