How to Switch Multiple Case in Java
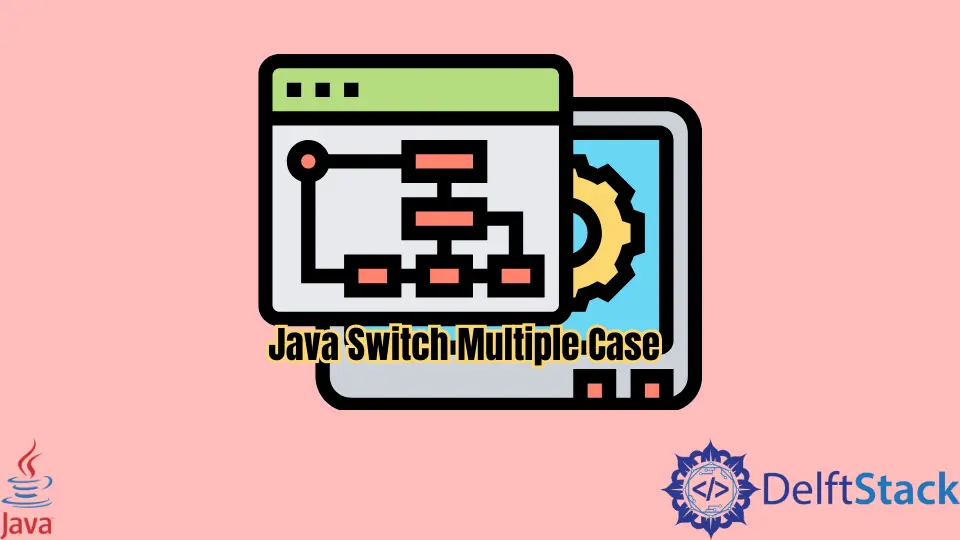
This tutorial demonstrates the multiple case switch
statement in Java.
Java Switch
Multiple Case
The switch
statement is a multi-way branch statement used instead of the if-elseif
scenario. The switch
statement will execute one statement for multiple conditions.
These conditions are assigned in cases
, and a switch
statement can have multiple cases. The expression for the switch
statement must be one of the byte
, short
, char
, and int
primitive types.
While working with switch
statements with multiple cases, we must consider some important points.
- The
switch
statement can have any number of cases, but duplicate cases will not be allowed. - The variables are not allowed when the value must be literal.
- The value for a case will be the same data type in the expression variable.
- The
break
statement can be used to terminate the statement, but it is optional, and if it is omitted, the execution will continue and move on to the next case. - The
default
statement is also optional in theswitch
statement, which is used for thedefault
case.
Let’s see the syntax for a switch
statement with multiple cases.
switch (Variable / Expression) {
case Case_Value1:
case Case_Value2:
case Case_Value3:
case Case_Value4:
// code inside the case
// optional break
break;
case Case_Value5:
case Case_Value6:
case Case_Value7:
case Case_Value8:
// code inside the case value
// optional
break;
default:
// code inside the default case.
}
Let’s try an example to demonstrate the switch
statement with multiple cases.
package delftstack;
import java.util.Scanner;
public class Example {
public static void main(String[] args) {
// Declaring a variable for switch expression
Scanner Demo_Input = new Scanner(System.in);
System.out.println("Please enter the name of a month to know how many days it contains: ");
String Month_Name = Demo_Input.nextLine();
switch (Month_Name) {
// Case statements
case "January":
case "March":
case "May":
case "July":
case "September":
case "November":
System.out.println("This month has 31 days");
break;
case "February":
System.out.println("This month has 28 days");
break;
case "April":
case "June":
case "August":
case "October":
case "December":
System.out.println("This month has 30 days");
break;
// Default case statement
default:
System.out.println("Please enter valid month");
}
}
}
The code above will use a switch
statement with multiple cases to check the number of days for the given month. See output:
Please enter the name of a month to know how many days it contains:
February
This month has 28 days
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook