Java switch Statement on Strings
- Understanding the Switch Statement in Java
- Syntax of the Switch Statement
- Example of Using Switch Statement with Strings
- Benefits of Using Switch Statements with Strings
- Common Mistakes to Avoid
- Conclusion
- FAQ
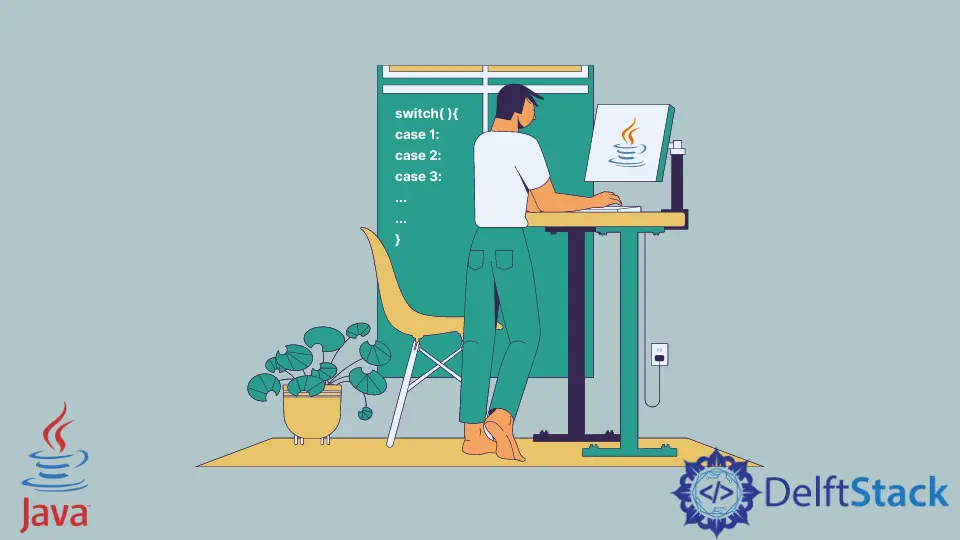
In the world of programming, control flow is essential for making decisions based on different conditions. One of the most useful tools in Java for this purpose is the switch statement. While many developers are familiar with using switch statements for integers and enums, using them with strings can be equally powerful and efficient.
In this tutorial, we will explore how to utilize the switch statement with strings in Java. We will cover the syntax, provide clear examples, and explain the nuances of this feature. By the end, you will have a solid understanding of how to implement switch statements with strings in your Java applications.
Understanding the Switch Statement in Java
Before diving into string-specific usage, let’s briefly revisit what a switch statement is. A switch statement allows you to execute different parts of code based on the value of a variable. This can be particularly useful when you have multiple conditions to evaluate, making your code cleaner and more readable compared to a series of if-else statements.
In Java, the switch statement can evaluate variables of types such as int
, char
, enum
, and, as of Java 7, String
. This enhancement allows for more flexibility and enhances code clarity when dealing with string comparisons.
Syntax of the Switch Statement
The basic syntax of a switch statement in Java is straightforward:
switch (expression) {
case value1:
// code block
break;
case value2:
// code block
break;
default:
// default code block
}
Here, the expression
is evaluated, and the program control jumps to the case that matches the expression’s value. The break
statement is crucial as it prevents the code from falling through to subsequent cases. If no case matches, the default
block is executed.
Example of Using Switch Statement with Strings
Now, let’s see a practical example of how to use a switch statement with strings in Java. In this example, we will create a simple program that takes a string input representing a day of the week and outputs whether it is a weekday or weekend.
import java.util.Scanner;
public class DayChecker {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter a day of the week: ");
String day = scanner.nextLine();
switch (day.toLowerCase()) {
case "monday":
case "tuesday":
case "wednesday":
case "thursday":
case "friday":
System.out.println(day + " is a weekday.");
break;
case "saturday":
case "sunday":
System.out.println(day + " is a weekend.");
break;
default:
System.out.println("Invalid day entered.");
}
scanner.close();
}
}
Output:
Enter a day of the week: Wednesday
Wednesday is a weekday.
This code snippet starts by importing the necessary Scanner class for user input. The user is prompted to enter a day of the week. The input is then converted to lowercase to ensure that the switch statement is case-insensitive. The switch statement evaluates the input and checks it against various cases. If the input matches a weekday, it outputs that it is a weekday; if it matches a weekend, it outputs that it is a weekend. If the input does not match any case, it informs the user that they entered an invalid day.
Benefits of Using Switch Statements with Strings
Using switch statements with strings offers several advantages. First, it enhances code readability. Instead of cluttering your code with multiple if-else statements, a switch statement allows for a cleaner and more organized approach. This can be particularly beneficial in larger applications where clarity is paramount.
Second, switch statements can be more efficient than multiple if-else checks, especially when dealing with numerous conditions. The switch statement jumps directly to the matching case, which can lead to better performance in certain scenarios.
Lastly, the ability to use strings in switch statements allows developers to create more intuitive and user-friendly applications. For instance, in our day-checker example, users can input days in any case format, making the application more flexible and accessible.
Common Mistakes to Avoid
While using switch statements with strings can simplify your code, there are common pitfalls to be aware of. One frequent mistake is forgetting to include the break
statement. Omitting this can lead to unexpected behavior, as the code will continue executing into the subsequent cases until it encounters a break or reaches the end of the switch.
Another mistake is not considering the case sensitivity of string comparisons. Using toLowerCase()
or toUpperCase()
on the input string can help mitigate this issue, ensuring that the switch statement behaves as expected regardless of how the user inputs the string.
Conclusion
In summary, the switch statement on strings in Java provides a powerful way to manage control flow in your applications. With its straightforward syntax and enhanced readability, it allows developers to handle multiple conditions efficiently. By using the examples and explanations provided in this tutorial, you can confidently implement switch statements in your Java projects, creating cleaner and more maintainable code. As you continue to explore Java, remember that mastering control flow is key to becoming a proficient programmer.
FAQ
-
can I use switch statements with other data types in Java?
yes, switch statements can also be used with integers, characters, and enums. -
what happens if I forget to include a break statement?
if you omit the break statement, the code will continue executing into the subsequent cases, which may lead to unexpected results. -
is the switch statement case-sensitive?
yes, string comparisons in switch statements are case-sensitive unless you convert the input to a consistent case using methods like toLowerCase(). -
can I use a switch statement for string comparisons in Java 6?
no, string comparisons in switch statements were introduced in Java 7. -
what should I do if none of the cases match?
you can use the default case to handle situations where no matches are found, providing a fallback option for unexpected input.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook