How to Declare Enum in Java
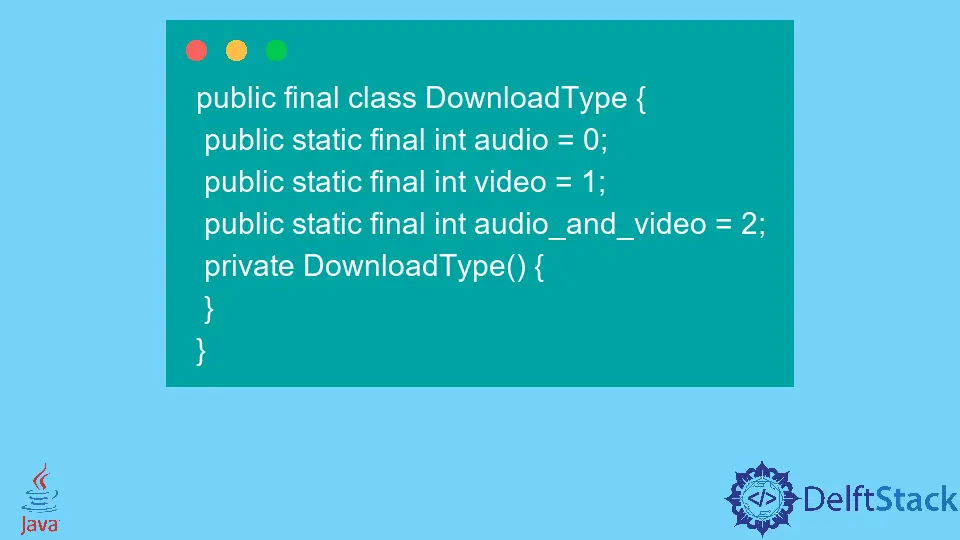
Declaring an enum
for a DownloadType
in Java will be the topic of discussion in this article. Let’s start by looking at the purpose of enums
.
Purpose of Enums
in Java
- Enumerations are used so that a collection of objects may be maintained as constants inside a program without the need to depend on an external value, such as an
integer
. - In Java,
enums
may be used as input onswitch
statements, and they can be compared securely using the==
or equality operator, among other comparison methods.
Because enums
are essentially objects of their type and not primitives, you can’t get around having to call a method or use a .value
to extract the value from an enum
if you need to do so.
To begin, we have to ask ourselves whether or not an int
is required. Then there will be two possible outcomes, which we will go through in the following section.
an int
Is Required
When you want an integer, you are willing to risk the loss of type safety and the possibility that users would send invalid information to your API. You can declare the below constants as integers as well.
Make the constructor private
when you only have static members and wish to simulate a static class in Java.
public final class DownloadType {
public static final int audio = 0;
public static final int video = 1;
public static final int audio_and_video = 2;
private DownloadType() {}
}
Since the .ordinal()
function is available regardless of whether the value field is present, the enum
might be defined as:
enum DownloadType { audio, video, audio_and_video }
To get the matching number for an enum
value, use the .ordinal()
function. Each enumerated value is automatically allocated a unique number beginning with zero and increasing by one for each successive value in the same sequence as declared.
DownloadType.audio_and_video.ordinal();
No int
Required
The integer value of an enum
isn’t always reliable. Use the value itself instead.
In cases when an associated integer is unnecessary, try this instead:
private enum DownloadType { audio, video, audio_and_video }
DownloadType dt = MyObj.getDownloadType();
if (dt == DownloadType.audio) {
}
You can write your logic in the switch
and if()
body to perform actions. Further, you can use the switch
statement instead of the if()
condition.
private enum DownloadType { audio, video, audio_and_video }
DownloadType dt = MyObj.getDownloadType();
switch (dt) {
case audio:
break;
case video:
break;
case audio_and_video:
break;
}
Good Practices
- You will compromise your program’s type-safety if you use integer constants instead of enumeration constants. Therefore try to avoid doing so.
- When you don’t necessarily have to, avoid combining an integer with an
enum
in your code.
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn