How to Iterate Through Enum Values in Java
- Brief Introduction to Enums (Enumeration) in Java
-
Iterate Through Enum Values Using the
for
Loop in Java -
Iterate Through Enum Values Using the
forEach
Loop in Java -
Iterate Through Enum Values Using
java.util.Stream
- Conclusion
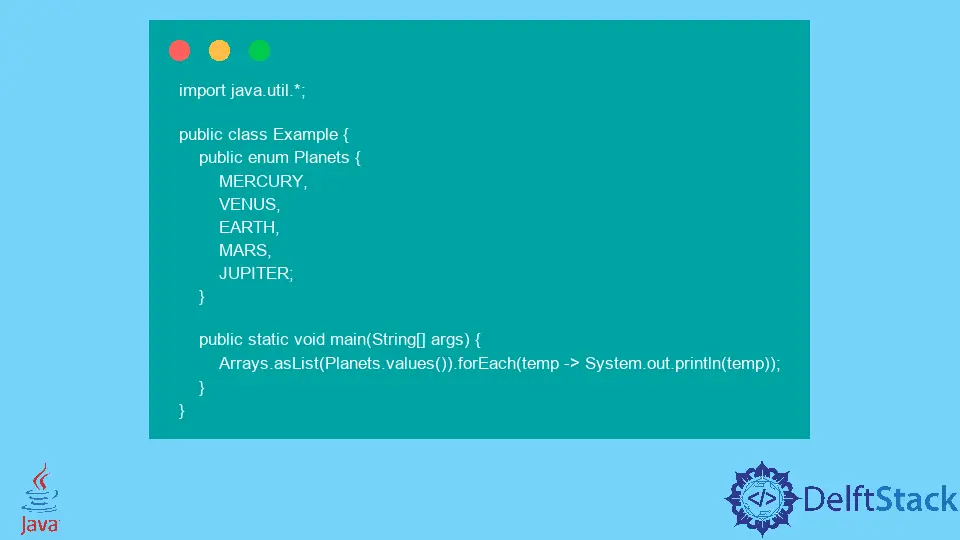
In this article, we will learn how to iterate through enums (enumeration) in Java.
Brief Introduction to Enums (Enumeration) in Java
In the Java programming language, enumerations help us to represent a group of constants. For instance, different planets of the solar system may be enumerations named Mercury, Venus, Earth, Mars, Jupiter, etc., belonging to an enumeration type named Planets
.
Enums are used to define our own data types, i.e., user-defined data types.
In Java, enums are declared using the enum
keyword. Unlike C/C++, Java enums are more efficient as we can add methods, constructors, and variables.
A common question that arises is: when and why to use them? We should use enums when we know that the values we will use will not change, like colors, days of the month, days of the week, names of planets, etc.
Now, let’s see different ways to iterate through the enums.
Iterate Through Enum Values Using the for
Loop in Java
All enums in Java implicitly extend the java.lang.Enum
class. This class contains a method values()
that returns all the values inside the enum.
We can use this method to loop through the values of an enum.
Example code:
import java.util.*;
public class Example {
public enum Planets {
MERCURY,
VENUS,
EARTH,
MARS,
JUPITER;
}
public static void main(String[] args) {
Planets values[] = Planets.values();
for (Planets obj : values) {
System.out.println(obj + " is the " + obj.ordinal() + " planet in the solar system");
}
}
}
Output:
MERCURY is the 0 planet in the solar system
VENUS is the 1 planet in the solar system
EARTH is the 2 planet in the solar system
MARS is the 3 planet in the solar system
JUPITER is the 4 planet in the solar system
In the above code, as we haven’t explicitly specified any value by default, the enum constants are assigned values starting from 0
.
Iterate Through Enum Values Using the forEach
Loop in Java
Since Java 8, the forEach()
method was added to the Iterable
interface. So all the Collections
classes in Java have the foreach()
method implementations.
We can use this method with an Enum
, but we must first convert it to a collection
. One common way of doing it is using the Arrays.asList()
method of java.util.Arrays
class.
This method gives us the list view of the array.
Example code:
import java.util.*;
public class Example {
public enum Planets {
MERCURY,
VENUS,
EARTH,
MARS,
JUPITER;
}
public static void main(String[] args) {
Arrays.asList(Planets.values()).forEach(temp -> System.out.println(temp));
}
}
Output:
MERCURY
VENUS
EARTH
MARS
JUPITER
Iterate Through Enum Values Using java.util.Stream
We can use java.util.Stream
to iterate over the enum values. To do so, we first create a stream of enum.values()
using the Stream.of()
method.
We pass the enum.values()
as parameter to the Stream.of()
function.
Example code:
import java.util.stream.*;
public class Example {
public enum Planets {
MERCURY,
VENUS,
EARTH,
MARS,
JUPITER;
}
public static void main(String[] args) {
Stream.of(Planets.values()).forEach(System.out::println);
}
}
Output:
MERCURY
VENUS
EARTH
MARS
JUPITER
Conclusion
This article taught us different ways of iterating over an enum using the for
loop, the forEach
loop, and Stream
in Java. We can use any of these methods to iterate, but when dealing with the parallel operation, Stream
is a better option.