How to Convert Enum to Int in Java
-
Introduction to Converting
Enum
toInt
in Java -
Convert
Enum
toInt
in Java -
Best Practices for
Enum
toInt
Conversion in Java - Conclusion
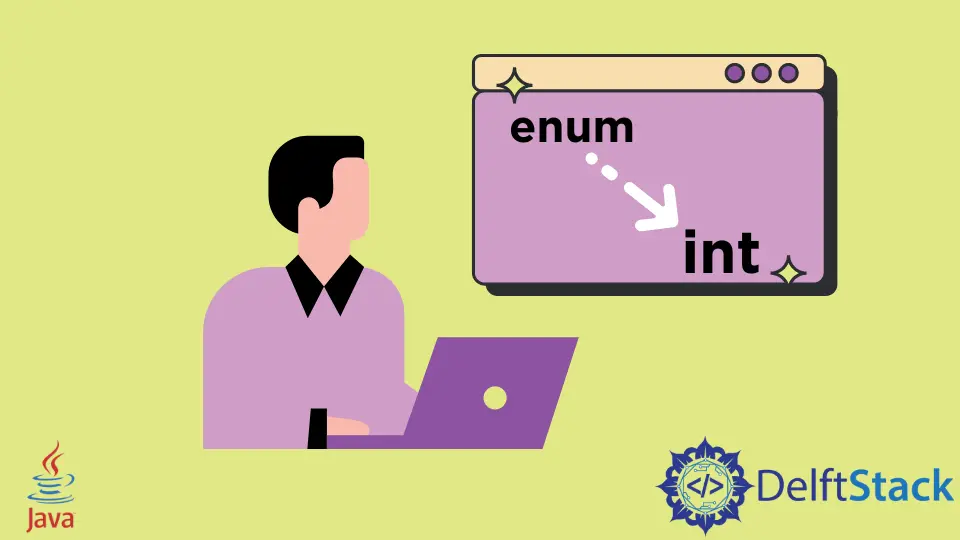
In this comprehensive article, we delve into the significance of converting enums
to integers in Java, exploring the various methods available for this task, including ordinal()
, custom fields, maps, and the valueOf()
method. Additionally, we will discuss best practices, providing valuable insights to guide developers in making informed decisions when performing enum-to-int
conversions.
Introduction to Converting Enum
to Int
in Java
Converting enums
to integers in Java is important for several reasons.
Firstly, integer representations of enums
facilitate efficient storage and manipulation in scenarios where numerical values are more suitable than symbolic names. Additionally, integer representations are often required when interacting with external systems or APIs that expect numerical inputs.
This conversion enables seamless integration with databases or protocols that rely on numeric codes. Furthermore, numerical representations can be advantageous in switch statements, mathematical computations, or array indexing.
Overall, converting enums
to ints
enhances flexibility, interoperability, and the practical utility of enum
constants in various programming contexts.
Convert Enum
to Int
in Java
Using the ordinal()
Method in Java
The ordinal()
method is important for converting enums
to int
in Java due to its simplicity and direct association with the order of enum
constants. It provides a quick and convenient way to obtain a numeric representation, especially when the sequence of constants is meaningful.
While it offers simplicity, it’s crucial to consider potential fragility if the order of enum
constants changes, making it essential to use ordinal()
judiciously based on the specific requirements of the application.
We’ll demonstrate how to convert an enum
constant to an integer using the ordinal()
method.
public class EnumToIntExample {
// Enum representing days of the week
public enum DaysOfWeek {
SUNDAY,
MONDAY,
TUESDAY,
WEDNESDAY,
THURSDAY,
FRIDAY,
SATURDAY;
}
public static void main(String[] args) {
// Converting an enum constant to int using ordinal()
int dayIndex = DaysOfWeek.WEDNESDAY.ordinal();
// Displaying the result
System.out.println("Index of WEDNESDAY: " + dayIndex);
}
}
In our example, we have an enum
called DaysOfWeek
that represents the days of the week. Enum
constants in Java are automatically assigned ordinal values based on their order of declaration, starting from 0.
In the EnumToIntExample
class’s main method, we showcase the conversion of an enum
constant to an integer using the ordinal()
method. For this illustration, we selected the WEDNESDAY
constant.
Following the conversion, we display the result by printing the obtained integer value to the console. This simple example highlights the straightforward process of utilizing ordinal()
for converting enums
to integers in Java.
When you run the program, it will output:
The output confirms that the ordinal()
method returns the index of the WEDNESDAY
enum
constant, which is 3. It’s important to note that ordinal()
is zero-based, so the index starts from 0.
Using Custom Fields in Java
The custom field method is important for converting enums
to int
in Java as it offers explicit control over the numeric representation of enum
constants. By introducing a dedicated field, it enhances code readability and provides resilience to changes in the order of enum
constants.
This method is particularly valuable when a stable and explicit mapping is essential, contributing to improved maintainability and reducing potential fragility compared to methods relying on implicit ordinal values.
Let’s delve into a practical example to illustrate the conversion of an enum
to an int
using custom fields.
public class EnumToIntCustomFieldExample {
// Enum representing days of the week
public enum DaysOfWeek {
SUNDAY(0),
MONDAY(1),
TUESDAY(2),
WEDNESDAY(3),
THURSDAY(4),
FRIDAY(5),
SATURDAY(6);
private final int intValue;
// Constructor to initialize the custom field
DaysOfWeek(int intValue) {
this.intValue = intValue;
}
// Getter for the custom field
public int getIntValue() {
return intValue;
}
}
public static void main(String[] args) {
// Converting an enum constant to int using custom field
int dayIndex = DaysOfWeek.WEDNESDAY.getIntValue();
// Displaying the result
System.out.println("Index of WEDNESDAY: " + dayIndex);
}
}
In the presented example, the DaysOfWeek
enum incorporates a custom field called intValue
. This dedicated field serves the purpose of explicitly linking each enum constant with a distinct integer value, providing a clear and controlled mapping.
Within the main method of the EnumToIntCustomFieldExample
class, we showcase the conversion of an enum constant to an integer. This is achieved by accessing the custom field associated with the chosen constant, in this instance, the WEDNESDAY
constant.
Following the conversion process, the program proceeds to display the result by printing the obtained integer value to the console. This concise demonstration illustrates the effectiveness of using a custom field for enum-to-int
conversions, promoting clarity and explicitness in managing the numeric representation of enum
constants.
When you run the program, it will output:
Using custom fields provides a clear and explicit way to manage the numeric representation of enum
constants, enhancing code readability and maintainability.
Using Map
in Java
The map method is crucial for converting enums
to int
in Java as it establishes a clear, customizable association between enum
constants and their integer values. Offering flexibility and avoiding reliance on ordinal positions, it ensures a robust mapping that remains unaffected by changes in enum
order.
The use of a map enhances code maintainability and readability, making it particularly valuable when explicit and adaptable mappings are desired in enum-to-int
conversions.
We’ll demonstrate how to employ a Map
for seamless conversion of enum
constants to integers.
import java.util.HashMap;
import java.util.Map;
public class EnumToIntMapExample {
// Enum representing days of the week
public enum DaysOfWeek {
SUNDAY,
MONDAY,
TUESDAY,
WEDNESDAY,
THURSDAY,
FRIDAY,
SATURDAY;
}
// Map for enum to int conversion
private static final Map<DaysOfWeek, Integer> enumToIntMap = new HashMap<>();
static {
enumToIntMap.put(DaysOfWeek.SUNDAY, 0);
enumToIntMap.put(DaysOfWeek.MONDAY, 1);
enumToIntMap.put(DaysOfWeek.TUESDAY, 2);
enumToIntMap.put(DaysOfWeek.WEDNESDAY, 3);
enumToIntMap.put(DaysOfWeek.THURSDAY, 4);
enumToIntMap.put(DaysOfWeek.FRIDAY, 5);
enumToIntMap.put(DaysOfWeek.SATURDAY, 6);
}
public static void main(String[] args) {
// Converting an enum constant to int using the map
int dayIndex = enumToIntMap.get(DaysOfWeek.WEDNESDAY);
// Displaying the result
System.out.println("Index of WEDNESDAY: " + dayIndex);
}
}
In the presented example, the DaysOfWeek enum
defines the days of the week without explicitly assigning ordinal values, allowing for flexibility in the order of enum
constants.
To facilitate the conversion of enum
constants to integers, a Map
named enumToIntMap
is introduced. This map establishes a clear association between each enum
constant and its corresponding integer value, with the static initializer block populating the map with the desired associations.
In the main method of the EnumToIntMapExample
class, we illustrate the conversion of an enum
constant to an integer using the map approach. Specifically, we’ve chosen the WEDNESDAY
constant for this example.
Following the conversion, the program proceeds to display the result by printing the obtained integer value to the console. This concise explanation emphasizes the effectiveness of using a map for enum-to-int
conversions, providing a clear and maintainable mechanism for managing these mappings.
When you run the program, it will output:
Utilizing a map for enum-to-int
conversions provides a robust and clear mechanism for managing such mappings, ensuring code readability and maintainability.
Using valueOf()
Method in Java
The valueOf
method is vital for converting enums
to int
in Java as it allows direct retrieval of an enum
constant by name, providing clarity and flexibility. This method eliminates dependencies on enum
order or additional fields, offering a concise and readable approach.
It excels in scenarios where explicit naming and a straightforward conversion process are paramount, contributing to code simplicity and ease of maintenance compared to other methods.
We’ll demonstrate how to utilize the valueOf()
method to convert an enum
constant to an integer.
public class EnumToIntValueOfExample {
// Enum representing days of the week
public enum DaysOfWeek {
SUNDAY,
MONDAY,
TUESDAY,
WEDNESDAY,
THURSDAY,
FRIDAY,
SATURDAY;
}
public static void main(String[] args) {
// Converting an enum constant to int using valueOf()
int dayIndex = convertEnumToInt(DaysOfWeek.WEDNESDAY);
// Displaying the result
System.out.println("Index of WEDNESDAY: " + dayIndex);
}
// Method to convert enum to int using valueOf()
private static int convertEnumToInt(DaysOfWeek day) {
return DaysOfWeek.valueOf(day.name()).ordinal();
}
}
In our example, the DaysOfWeek enum
succinctly represents the days of the week without explicit ordinal assignments, allowing for a straightforward focus on the conversion process.
Within the main method of the EnumToIntValueOfExample
class, we showcase the conversion of an enum
constant to an integer using the valueOf()
method. Specifically, we highlight the example of converting the WEDNESDAY
constant.
The conversion logic is encapsulated in a dedicated method named convertEnumToInt
. This method efficiently utilizes the valueOf()
method to obtain the enum
constant by its name and subsequently retrieves its ordinal value.
This approach provides a concise and effective means of converting enums
to integers, leveraging the inherent capabilities of the valueOf()
method. Upon execution, the program output confirms the successful conversion, displaying the ordinal value of the WEDNESDAY
constant as 3.
When you run the program, it will output:
This method provides a concise and straightforward approach, leveraging the inherent capabilities of enums
for efficient conversion.
Best Practices for Enum
to Int
Conversion in Java
Use Custom Fields for Integer Values
When converting enums
to integers in Java, consider adding a custom field to your enum
class to explicitly store integer values. This enhances code readability and avoids reliance on the ordinal values, which may change with enum
modifications.
Utilize a Map
for Mapping Enum
Constants to Integers
Consider using a Map
to establish a clear association between enum
constants and their corresponding integer values. This approach provides flexibility and avoids implicit dependencies on the order of enum
constants.
Prioritize Clarity and Maintenance
Opt for methods that enhance code clarity and maintenance. While the ordinal()
method is concise, it may be fragile if enum
constants are reordered. Choose approaches that explicitly convey the intent and minimize potential future issues.
Document Enum
Conversions
Regardless of the chosen method, document enum-to-int
conversions, especially if using ordinal values or custom fields. Clear documentation helps developers understand the mapping logic and prevents unintentional errors during maintenance or modifications.
Conclusion
This article explored diverse methods—ordinal()
, custom fields, maps, and valueOf()
—for converting enums
to integers in Java. Each method caters to specific needs, providing developers with versatile options.
Additionally, we discussed best practices, emphasizing the importance of clarity, flexibility, and resilience in the conversion process. By understanding and applying these methods judiciously, developers can navigate enum-to-int conversions effectively, ensuring code readability and maintainability in their Java projects.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook