String Padding in Java
-
Use the
String.format()
Method to Pad a String in Java -
Use the
Apache Common Lang
Package to Pad a String in Java
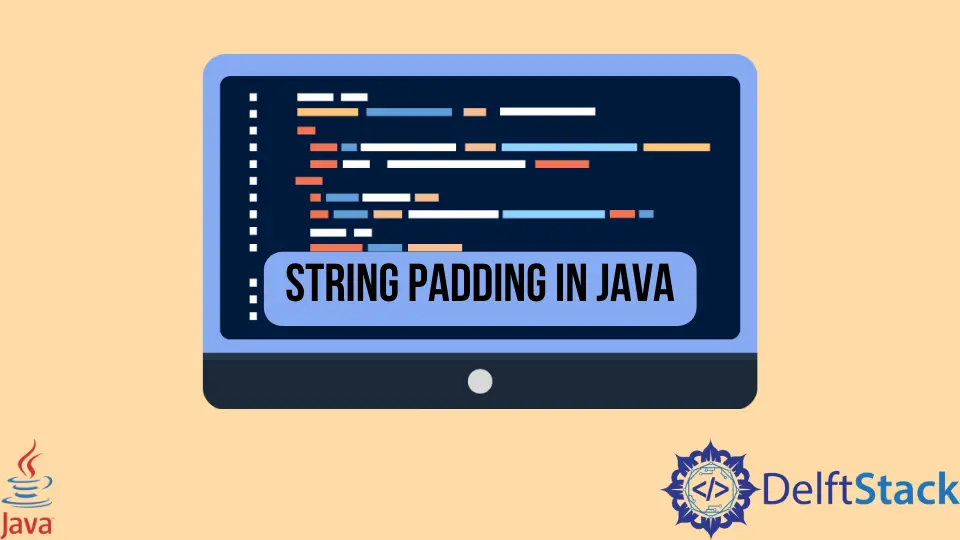
In this article, we’ll see how we can pad a string using two methods in Java.
Use the String.format()
Method to Pad a String in Java
We will use a string method in java called format()
. Remember that this method enables you to do the Left Padding
and the Right Padding
.
Code Example:
// Importing necessary packages.
import java.io.*;
import java.lang.*;
public class StringPadding {
// The function for left padding.
public static String StrLeft(String input, char ch, int Len) {
String result = String.format("%" + Len + "s", input).replace(' ', ch);
return result;
}
// The function right padding
public static String StrRight(String input, char ch, int Len) {
String result = String.format("%" + (-Len) + "s", input).replace(' ', ch);
return result;
}
public static void main(String[] args) {
String str = "DelftStack";
char ReplaceChar = '+';
int Len = 25;
System.out.println(StrLeft(str, ReplaceChar, Len));
System.out.println(StrRight(str, ReplaceChar, Len));
}
}
In our above code example, we illustrated the padding on a string. In both padding functions StrRight()
and StrLeft()
, we first right-pad the string according to the length provided by using the space.
Then we replace these spaces with the character provided.
Output:
+++++++++++++++DelftStack
DelftStack+++++++++++++++
Use the Apache Common Lang
Package to Pad a String in Java
This method is highly recommended for you if you want to pad a string in the center, but you also can pad a string left and right. The class we will use from the package is StringUtils
.
Before using this method, you must import the necessary package file for Apache Common Lang
.
Code Example:
// Importing necessary packages.
import java.io.*;
import java.lang.*;
import org.apache.commons.lang3.StringUtils;
public class StringPadding {
// Function for left padding
public static String StringLeft(String MainStr, char ReplaceChar, int Len) {
String result = StringUtils.leftPad(MainStr, Len, ReplaceChar);
return result;
}
// Function for center padding
public static String StringCenter(String MainStr, char ReplaceChar, int Len) {
String result = StringUtils.center(MainStr, Len, ReplaceChar);
return result;
}
// Function for right padding
public static String StringRight(String MainStr, char ReplaceChar, int Len) {
String result = StringUtils.rightPad(MainStr, Len, ReplaceChar);
return result;
}
// Main Driver Class
public static void main(String[] args) {
String MainStr = "DelftStack"; // Our main string that needs to be pad
char ReplaceChar = '-'; // Character that replace the " "
int Len = 20; // String length
System.out.println(StringLeft(MainStr, ReplaceChar, Len));
System.out.println(StringCenter(MainStr, ReplaceChar, Len));
System.out.println(StringRight(MainStr, ReplaceChar, Len));
}
}
In our above example, we illustrated a simple example of string padding and commanded the purpose of functions and lines.
Output:
----------DelftStack
-----DelftStack-----
DelftStack----------
Please note that the code examples shared here are written in Java, and you must install Java on your environment if your system doesn’t contain Java.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn