How to Get Character in String by Index in Java
-
Get
String
Character UsingcharAt()
Method in Java -
Convert Char to String by Using the
String.valueOf()
Method - Getting Char Array From a String in Java
- Fetching Characters of the Unicode Supplementary Multilingual Plane (SMP)
- Summary
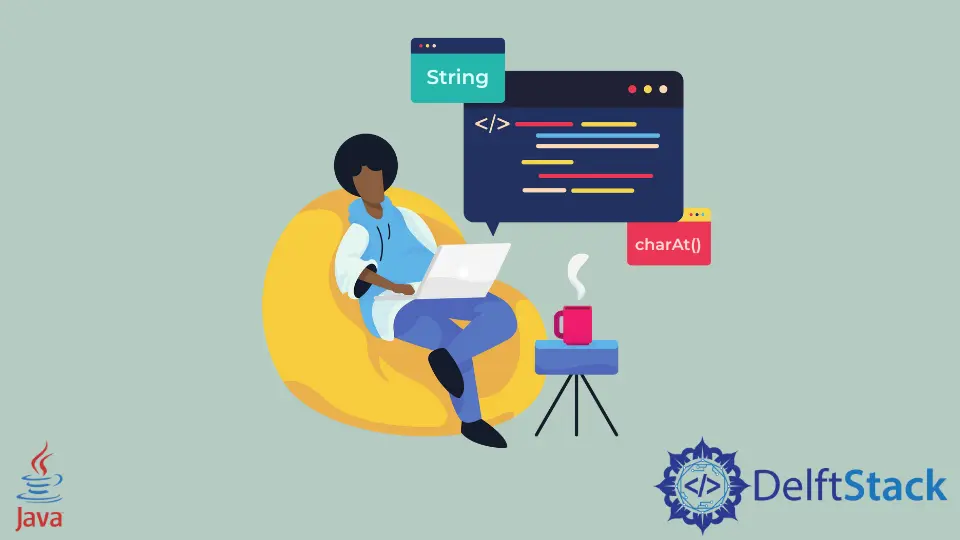
This tutorial introduces how to get String
characters by index in Java and also lists some example codes to understand the topic.
Strings are used to store a sequence of characters. Just like arrays, strings also follow zero-based indexing. This means that the first character of the string is assigned an index value of zero. We can fetch individual characters from a string by using their indices. In this tutorial, we will learn how to do this.
Get String
Character Using charAt()
Method in Java
The charAt()
method takes an index value as a parameter and returns a character present at that index in the string. It is the simplest way to fetch characters from a string and is a String
class method.
public class Demo {
public static void main(String[] args) {
String s = "test string";
char characterAtIdx1 = s.charAt(1); // character e
char characterAtIdx4 = s.charAt(4); // whitespace
char characterAtIdx5 = s.charAt(5); // character s
System.out.println("The string is: " + s);
System.out.println("Character at Index 1: " + characterAtIdx1);
System.out.println("Character at Index 4: " + characterAtIdx4);
System.out.println("Character at Index 5: " + characterAtIdx5);
}
}
Output:
The string is: test string
Character at Index 1: e
Character at Index 4:
Character at Index 5: s
As we saw in the code above, the return type of this method is char. We can convert this char type to a string by using the toString()
method of the Character
class and even get the length of this string using the length()
method.
public class Demo {
public static void main(String[] args) {
String s = "test string";
char characterAtIdx6 = s.charAt(6); // character t
String stringAtIdx6 = Character.toString(characterAtIdx6); // char to string
System.out.println("Length: " + stringAtIdx6.length());
System.out.println("String: " + stringAtIdx6);
}
}
Output:
Length: 1
String: t
Convert Char to String by Using the String.valueOf()
Method
After getting a char from a string, we can use the String.valueOf()
method to convert a char to a String
if required.
public class Demo {
public static void main(String[] args) {
String s = "test string";
char characterAtIdx6 = s.charAt(6); // character t
String stringAtIdx6 = String.valueOf(characterAtIdx6); // char to string
System.out.println("Length: " + stringAtIdx6.length());
System.out.println("String: " + stringAtIdx6);
}
}
Output:
Length: 1
String: t
We can also concatenate an empty string to the character to convert it to a string. Quotes with nothing in between represent an empty string. This is one of the simplest and implicit way to get a String
object in Java.
public class Demo {
public static void main(String[] args) {
String s = "test string";
char characterAtIdx6 = s.charAt(6); // character t
String stringAtIdx6 = "" + characterAtIdx6; // char to string
System.out.println("Length: " + stringAtIdx6.length());
System.out.println("String: " + stringAtIdx6);
}
}
Output:
Length: 1
String: t
Getting Char Array From a String in Java
We can get an array element by its index with the help of square brackets. If we convert our string to a char type array, we can fetch any character from it.
Java provides a convenient toCharArray()
method that returns a char array. We can use this method on a string and get a char array for it.
public class Demo {
public static void main(String[] args) {
String s = "test string";
char[] charArr = s.toCharArray();
System.out.println("The string is: " + s);
System.out.println("Character at Index 1: " + charArr[1]);
System.out.println("Character at Index 4: " + charArr[4]);
System.out.println("Character at Index 5: " + charArr[5]);
}
}
Output:
The string is: test string
Character at Index 1: e
Character at Index 4:
Character at Index 5: s
We can use the methods described in the previous section to convert the char to a string.
public class Demo {
public static void main(String[] args) {
String s = "test string";
char[] charArr = s.toCharArray();
char characterAtIdx6 = charArr[6]; // character t
String str1 = Character.toString(characterAtIdx6); // char to string
String str2 = String.valueOf(characterAtIdx6); // char to string
String str3 = "" + characterAtIdx6; // char to string
System.out.println("Using toString()");
System.out.println("Length: " + str1.length() + " String: " + str1);
System.out.println("\nUsing valueOf()");
System.out.println("Length: " + str2.length() + " String: " + str2);
System.out.println("\nUsing empty string concatenation");
System.out.println("Length: " + str3.length() + " String: " + str3);
}
}
Output:
Using toString()
Length: 1 String: t
Using valueOf()
Length: 1 String: t
Using empty string concatenation
Length: 1 String: t
Fetching Characters of the Unicode Supplementary Multilingual Plane (SMP)
In some rare cases, our string may contain characters not present in the Basic Unicode Multilingual Plane. An example could be a string of emojis or emoticons. These characters are part of the Unicode Supplementary Multilingual Plane(SMP).
The above approaches do not work for these characters. We can use the codePointAt()
method to fetch such characters from the string. This method returns an integer representing the Unicode value of the character at that index. Make sure you have the appropriate font installed to view these characters.
public class Demo {
public static void main(String[] args) {
String s = "😀 the grinning emoji";
for (int i = 0; i < s.length();) {
int codePoint = s.codePointAt(i);
char[] charArr = Character.toChars(
codePoint); // More than one characters may be required to represent an SMP char
System.out.print(new String(charArr) + " ");
i = i
+ Character.charCount(
codePoint); // Increase i according to the number of chars required for that SMP char
}
}
}
Output:
😀 t h e g r i n n i n g e m o j i
If we try to print the same string using the charAt()
method, we get the following output.
public class Demo
{
public static void main(String args[])
{
String s = "😀 the grinning emoji";
for(int i=0; i<s.length(); i++)
{
char c = s.charAt(i);
System.out.print(c + " ");
}
}
}
Output:
? ? t h e g r i n n i n g e m o j i
Summary
Strings, just like arrays, follow zero-based indexing. We can get individual characters from the string by using the charAt()
method. We can also convert the string to an array of characters and then fetch individual chars using their indices. If you want the single character as a string and not as a char, then use the Character.toString()
or the String.valueOf()
method to convert it. Sometimes our string may contain characters outside the Basic Multilingual Plane. In such cases, we can use the codePointAt()
method instead of charAt()
method.