Obtenir un caractère dans une chaîne par index en Java
-
Obtenir le caractère
String
en utilisant la méthodecharAt()
en Java -
Convertir Char en String en utilisant la méthode
String.valueOf()
- Obtenir un tableau de caractères à partir d’une chaîne en Java
- Extraction des caractères du plan multilingue supplémentaire (SMP) Unicode
- Sommaire
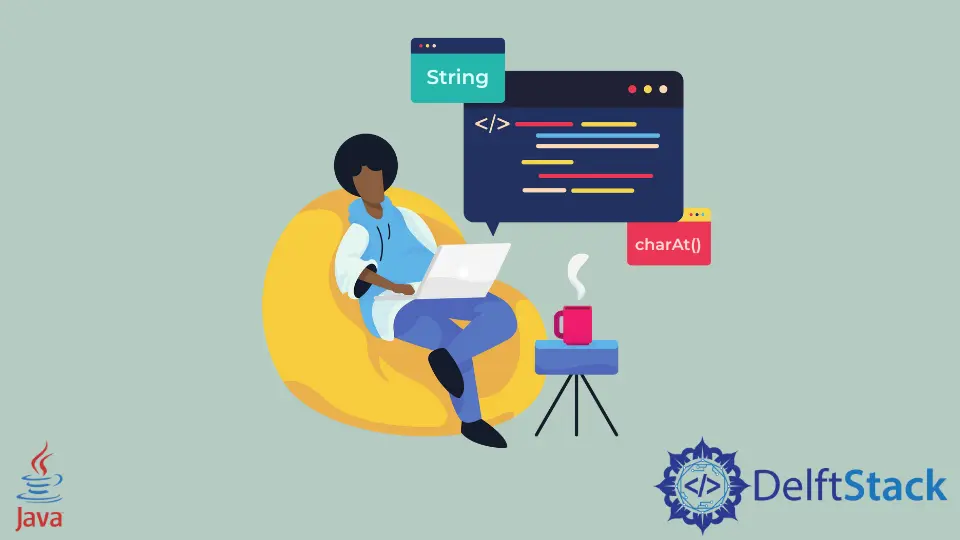
Ce tutoriel présente comment obtenir des caractères String
par index en Java et répertorie également quelques exemples de codes pour comprendre le sujet.
Les chaînes sont utilisées pour stocker une séquence de caractères. Tout comme les tableaux, les chaînes suivent également une indexation de base zéro. Cela signifie que le premier caractère de la chaîne se voit attribuer une valeur d’index de zéro. Nous pouvons récupérer des caractères individuels à partir d’une chaîne en utilisant leurs indices. Dans ce tutoriel, nous allons apprendre comment faire cela.
Obtenir le caractère String
en utilisant la méthode charAt()
en Java
La méthode charAt()
prend une valeur d’index en paramètre et renvoie un caractère présent à cet index dans la chaîne. C’est le moyen le plus simple d’extraire des caractères d’une chaîne et c’est une méthode de classe String
.
public class Demo {
public static void main(String[] args) {
String s = "test string";
char characterAtIdx1 = s.charAt(1); // character e
char characterAtIdx4 = s.charAt(4); // whitespace
char characterAtIdx5 = s.charAt(5); // character s
System.out.println("The string is: " + s);
System.out.println("Character at Index 1: " + characterAtIdx1);
System.out.println("Character at Index 4: " + characterAtIdx4);
System.out.println("Character at Index 5: " + characterAtIdx5);
}
}
Production:
The string is: test string
Character at Index 1: e
Character at Index 4:
Character at Index 5: s
Comme nous l’avons vu dans le code ci-dessus, le type de retour de cette méthode est char. On peut convertir ce type char en chaîne en utilisant la méthode toString()
de la classe Character
et même obtenir la longueur de cette chaîne en utilisant la méthode length()
.
public class Demo {
public static void main(String[] args) {
String s = "test string";
char characterAtIdx6 = s.charAt(6); // character t
String stringAtIdx6 = Character.toString(characterAtIdx6); // char to string
System.out.println("Length: " + stringAtIdx6.length());
System.out.println("String: " + stringAtIdx6);
}
}
Production:
Length: 1
String: t
Convertir Char en String en utilisant la méthode String.valueOf()
Après avoir obtenu un caractère à partir d’une chaîne, nous pouvons utiliser la méthode String.valueOf()
pour convertir un caractère en String
si nécessaire.
public class Demo {
public static void main(String[] args) {
String s = "test string";
char characterAtIdx6 = s.charAt(6); // character t
String stringAtIdx6 = String.valueOf(characterAtIdx6); // char to string
System.out.println("Length: " + stringAtIdx6.length());
System.out.println("String: " + stringAtIdx6);
}
}
Production:
Length : 1 String : t
Nous pouvons également concaténer une chaîne vide au caractère pour le convertir en chaîne. Les guillemets sans rien entre les deux représentent une chaîne vide. C’est l’un des moyens les plus simples et implicites d’obtenir un objet String
en Java.
public class Demo {
public static void main(String[] args) {
String s = "test string";
char characterAtIdx6 = s.charAt(6); // character t
String stringAtIdx6 = "" + characterAtIdx6; // char to string
System.out.println("Length: " + stringAtIdx6.length());
System.out.println("String: " + stringAtIdx6);
}
}
Production:
Length: 1
String: t
Obtenir un tableau de caractères à partir d’une chaîne en Java
Nous pouvons obtenir un élément de tableau par son indice à l’aide de crochets. Si nous convertissons notre chaîne en un tableau de type char, nous pouvons en extraire n’importe quel caractère.
Java fournit une méthode toCharArray()
pratique qui renvoie un tableau de caractères. Nous pouvons utiliser cette méthode sur une chaîne et obtenir un tableau de caractères pour celle-ci.
public class Demo {
public static void main(String[] args) {
String s = "test string";
char[] charArr = s.toCharArray();
System.out.println("The string is: " + s);
System.out.println("Character at Index 1: " + charArr[1]);
System.out.println("Character at Index 4: " + charArr[4]);
System.out.println("Character at Index 5: " + charArr[5]);
}
}
Production:
The string is: test string
Character at Index 1: e
Character at Index 4:
Character at Index 5: s
Nous pouvons utiliser les méthodes décrites dans la section précédente pour convertir le caractère en chaîne.
public class Demo {
public static void main(String[] args) {
String s = "test string";
char[] charArr = s.toCharArray();
char characterAtIdx6 = charArr[6]; // character t
String str1 = Character.toString(characterAtIdx6); // char to string
String str2 = String.valueOf(characterAtIdx6); // char to string
String str3 = "" + characterAtIdx6; // char to string
System.out.println("Using toString()");
System.out.println("Length: " + str1.length() + " String: " + str1);
System.out.println("\nUsing valueOf()");
System.out.println("Length: " + str2.length() + " String: " + str2);
System.out.println("\nUsing empty string concatenation");
System.out.println("Length: " + str3.length() + " String: " + str3);
}
}
Production:
Using toString()
Length: 1 String: t
Using valueOf()
Length: 1 String: t
Using empty string concatenation
Length: 1 String: t
Extraction des caractères du plan multilingue supplémentaire (SMP) Unicode
Dans de rares cas, notre chaîne peut contenir des caractères non présents dans le plan multilingue Unicode de base. Un exemple pourrait être une chaîne d’emojis ou d’émoticônes. Ces caractères font partie du Plan Multilingue Supplémentaire Unicode (SMP).
Les approches ci-dessus ne fonctionnent pas pour ces personnages. Nous pouvons utiliser la méthode codePointAt()
pour récupérer ces caractères dans la chaîne. Cette méthode renvoie un entier représentant la valeur Unicode du caractère à cet index. Assurez-vous que la police appropriée est installée pour afficher ces caractères.
public class Demo
{
public static void main(String[] args)
{
String s = "😀 the grinning emoji";
for(int i=0; i<s.length();)
{
int codePoint = s.codePointAt(i);
char[] charArr = Character.toChars(codePoint);//More than one characters may be required to represent an SMP char
System.out.print(new String(charArr) + " ");
i = i + Character.charCount(codePoint);//Increase i according to the number of chars required for that SMP char
}
}
}
Production:
😀 t h e g r i n n i n g e m o j i
Si nous essayons d’imprimer la même chaîne en utilisant la méthode charAt()
, nous obtenons la sortie suivante.
public class Demo
{
public static void main(String args[])
{
String s = "😀 the grinning emoji";
for(int i=0; i<s.length(); i++)
{
char c = s.charAt(i);
System.out.print(c + " ");
}
}
}
Production:
? ? t h e g r i n n i n g e m o j i
Sommaire
Les chaînes, tout comme les tableaux, suivent une indexation de base zéro. Nous pouvons obtenir des caractères individuels de la chaîne en utilisant la méthode charAt()
. Nous pouvons également convertir la chaîne en un tableau de caractères, puis extraire des caractères individuels à l’aide de leurs indices. Si vous voulez que le caractère unique soit une chaîne et non un caractère, utilisez la méthode Character.toString()
ou la méthode String.valueOf()
pour le convertir. Parfois, notre chaîne peut contenir des caractères en dehors du plan multilingue de base. Dans de tels cas, nous pouvons utiliser la méthode codePointAt()
au lieu de la méthode charAt()
.