How to Remove Element From Array and Then Shift Other Elements in Java
-
Use the
for
Loop to Remove Element From Array and Shift in Java -
Use
System.arraycopy()
to Remove Element From Array and Shift in Java -
Use
ArrayList
to Remove Element From Array and Shift in Java - Conclusion
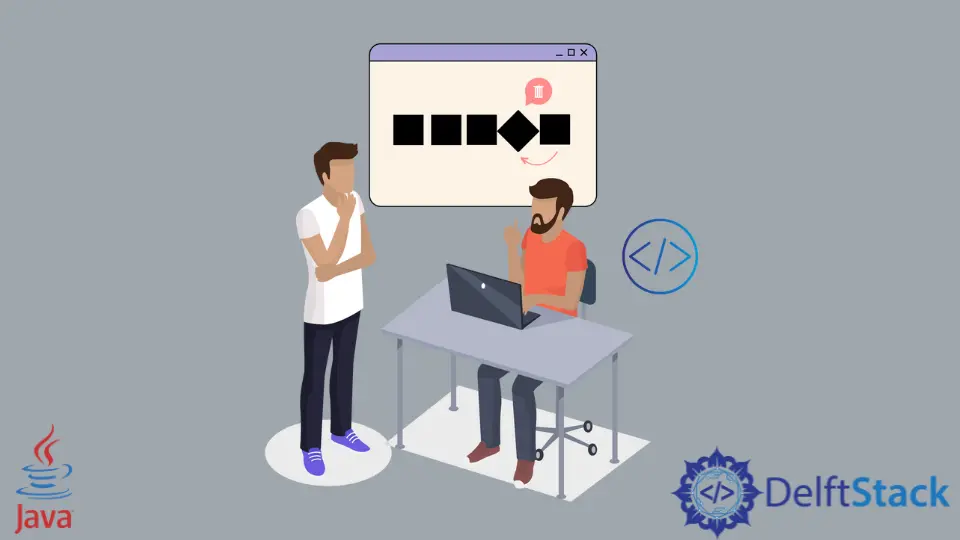
Arrays are a fundamental data structure in Java, offering a straightforward way to store collections of elements.
However, the rigid nature of arrays with a fixed size can pose challenges when it comes to removing elements while maintaining the array’s integrity.
In this article, we’ll explore multiple methods for removing an element from an array and shifting the remaining elements in Java. Throughout this discussion, we’ll cover methods employing for
loops, System.arraycopy()
, and ArrayList
.
Each method offers a unique approach to address this common issue, providing flexibility and versatility for array manipulation.
Use the for
Loop to Remove Element From Array and Shift in Java
One common approach to remove an element from an array and shift the remaining elements to close the gap is by using a for
loop. We will see how this is done through a demonstration below.
Consider an array with the following elements:
int[] array = {1, 2, 3, 4, 5};
Suppose we want to remove the element at a specific index (e.g., index 2
, which contains the value 3
) and shift the remaining elements to close the gap. After the operation, the array should look like this:
int[] array = {1, 2, 4, 5};
To achieve the desired result, we can use a for
loop to iterate through the array. Start by defining your array with the initial elements.
Then, decide on the index of the element you want to remove. For this example, let’s say you want to remove the element at index 2
(which contains the value 3
).
int indexToRemove = 2;
Verify that the index you want to remove is within the valid range of array indices. You should check if it’s greater than or equal to 0
and less than the array length to avoid index out-of-bounds errors.
if (indexToRemove >= 0 && indexToRemove < array.length) {
// Code to remove and shift
} else {
System.out.println("Index out of bounds");
}
Inside the if
statement, use a for
loop to iterate through the array, starting from the index to be removed. During the loop, copy the value from the next element to the current element to shift the array.
for (int i = indexToRemove; i < array.length - 1; i++) {
array[i] = array[i + 1];
}
In this loop, i
starts from the indexToRemove
, and in each iteration, it copies the value from the next element (array[i + 1]
) to the current element (array[i]
). This effectively shifts the elements in the array.
After the loop completes, the last element in the array (at array.length - 1
) will still contain the old value of the previous-to-last element. To close the gap, you can simply modify the array like this.
array = Arrays.copyOf(array, array.length - 1);
You can then print or use the modified array, which will have the desired element removed and the rest of the elements shifted accordingly.
Here’s the complete code:
import java.util.Arrays;
public class ArrayElementRemoval {
public static void main(String[] args) {
int[] array = {1, 2, 3, 4, 5};
int indexToRemove = 2;
if (indexToRemove >= 0 && indexToRemove < array.length) {
for (int i = indexToRemove; i < array.length - 1; i++) {
array[i] = array[i + 1];
}
array = Arrays.copyOf(array, array.length - 1);
System.out.println(Arrays.toString(array));
} else {
System.out.println("Index out of bounds");
}
}
}
In this code, the element at index 2
(which contains the value 3
) will be removed, and the remaining elements will be shifted to close the gap. The output of this code will be:
[1, 2, 4, 5]
After the operation, the array contains the elements [1, 2, 4, 5]
, which is the desired result where the element at index 2
has been removed, and the array elements have been shifted accordingly.
This approach follows a systematic process to remove an element from an array and shift the remaining elements to maintain the array’s integrity. Adjust the array and index as needed to fit your specific use case.
Remember to handle index out-of-bounds scenarios to ensure your code is robust and doesn’t result in runtime errors.
Use System.arraycopy()
to Remove Element From Array and Shift in Java
Removing an element from an array and shifting the remaining elements can be efficiently achieved using the System.arraycopy()
method.
Here’s the syntax of the System.arraycopy()
method:
System.arraycopy(Object src, int srcPos, Object dest, int destPos, int length);
Let’s break down each parameter:
src
: This is the source array or object from which elements will be copied.srcPos
: This is the starting position in the source array where the copying begins.dest
: This is the destination array or object where the elements will be copied to.destPos
: This is the starting position in the destination array where the copied elements will be placed.length
: This is the number of elements to copy from the source to the destination.
The System.arraycopy()
method is a low-level way to copy a range of elements from one array to another. It provides fine-grained control over the copying process.
Keep in mind that it requires the source and destination objects to be of the same type, and the source and destination ranges must not overlap.
Now, let’s start with an array containing the following elements:
int[] array = {2, 4, 6, 8, 10};
Suppose you want to remove an element at a specific index (e.g., index 2
, which contains the value 6
) and shift the remaining elements to close the gap. After this operation, the array should look like this:
int[] array = {2, 4, 8, 10};
In this scenario, we aim to remove the element at index 2
while ensuring there are no trailing zeros in the array.
Start by defining your array with the initial elements. Then, decide on the index of the element you want to remove.
In this example, let’s assume you want to remove the element at index 2
.
int indexToRemove = 2;
Verify that the index to be removed is within the valid range of array indices, ensuring it’s greater than or equal to 0
and less than the array length to prevent index out-of-bounds errors.
if (indexToRemove >= 0 && indexToRemove < array.length) {
// Code for removal and shifting
} else {
System.out.println("Index out of bounds");
}
Next, within the if
statement, apply System.arraycopy()
to remove the element and shift the remaining elements, ensuring there are no trailing zeros.
int elementsToShift = array.length - indexToRemove - 1;
System.arraycopy(array, indexToRemove + 1, array, indexToRemove, elementsToShift);
array = Arrays.copyOf(array, array.length - 1)
In this code, we calculate the number of elements to shift and then use System.arraycopy()
to move the elements. The source array is the original array, and the destination array is the same array with the same index (indexToRemove
) for both.
This effectively shifts the elements to the left while removing the specified element.
Finally, you can print or use the modified array, which will have the desired element removed and the remaining elements shifted without any trailing zeros.
Here’s the complete working code:
import java.util.Arrays;
public class ArrayElementRemoval {
public static void main(String[] args) {
int[] array = {2, 4, 6, 8, 10};
int indexToRemove = 2;
if (indexToRemove >= 0 && indexToRemove < array.length) {
int elementsToShift = array.length - indexToRemove - 1;
System.arraycopy(array, indexToRemove + 1, array, indexToRemove, elementsToShift);
array = Arrays.copyOf(array, array.length - 1);
System.out.println(Arrays.toString(array));
} else {
System.out.println("Index out of bounds");
}
}
}
The output will display the modified array:
[2, 4, 8, 10]
In this output, the element at the specified index has been removed, and the remaining elements have been shifted to preserve the array’s structure without any trailing zeros.
Use ArrayList
to Remove Element From Array and Shift in Java
ArrayList
is a dynamic array-like data structure in Java that allows you to add and remove elements without having to manage array resizing manually. To remove an element and shift the remaining elements in an array, we can convert the array to an ArrayList
, perform the removal, and then convert it back to an array.
Here’s a step-by-step guide on how to achieve this:
Start by defining your array with the initial elements. Consider the array with the following elements:
int[] array = {1, 2, 3, 4, 5};
Decide on the index of the element you want to remove. In this example, let’s assume you want to remove the element at index 2
.
int indexToRemove = 2;
Verify that the index to be removed is within the valid range of array indices, ensuring it’s greater than or equal to 0
and less than the array length to prevent index out-of-bounds errors.
if (indexToRemove >= 0 && indexToRemove < array.length) {
// Code for removal and shifting
} else {
System.out.println("Index out of bounds");
}
Inside the if
statement, convert the array to an ArrayList
to perform the removal and shifting efficiently.
ArrayList<Integer> arrayList = new ArrayList<>(Arrays.asList(array));
arrayList.remove(indexToRemove);
In this code, we create an ArrayList
from the array, allowing us to use the remove
method to eliminate the element at the specified index.
After removing the element, convert the ArrayList
back to an array.
array = arrayList.toArray(new Integer[0]);
You can then print or use the modified array, which will have the desired element removed and the remaining elements shifted.
Here’s the complete working Java code:
import java.util.ArrayList;
import java.util.Arrays;
public class ArrayElementRemoval {
public static void main(String[] args) {
int[] array = {1, 2, 3, 4, 5};
int indexToRemove = 2;
if (indexToRemove >= 0 && indexToRemove < array.length) {
ArrayList<Integer> arrayList =
new ArrayList<>(Arrays.asList(Arrays.stream(array).boxed().toArray(Integer[] ::new)));
arrayList.remove(indexToRemove);
array = arrayList.stream().mapToInt(Integer::intValue).toArray();
System.out.println(Arrays.toString(array));
} else {
System.out.println("Index out of bounds");
}
}
}
The output of this code will be:
[1, 2, 4, 5]
As you can see, the element at the specified index has been removed, and the remaining elements have been shifted, maintaining the array’s structure without trailing zeros.
In this output, the element at the specified index has been removed, and the other elements have been shifted to preserve the array’s structure without any trailing zeros.
Conclusion
In this article, we’ve delved into various methods for removing an element from an array and shifting the array in Java. Each method offers its unique advantages and can be chosen based on specific requirements and coding preferences.
Using a for
loop, we can iterate through the array and shift elements manually, resulting in a straightforward and hands-on approach. The System.arraycopy()
method provides a more efficient low-level approach, allowing us to copy and shift elements with fine-grained control.
Finally, leveraging an ArrayList
empowers us with dynamic capabilities, making it easy to remove and shift elements while maintaining array continuity.
By understanding and implementing these methods, Java developers can tackle the challenge of modifying arrays with confidence. The choice of method may vary based on factors like performance, code readability, and specific use cases, but having a toolkit of options ensures that you can select the most appropriate method for your unique coding scenario.
Ultimately, these methods are powerful tools that enhance your ability to work with arrays, one of the fundamental data structures in Java, and maintain the integrity of your data.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn