How to Fix Java.Net.SocketException: Network Is Unreachable
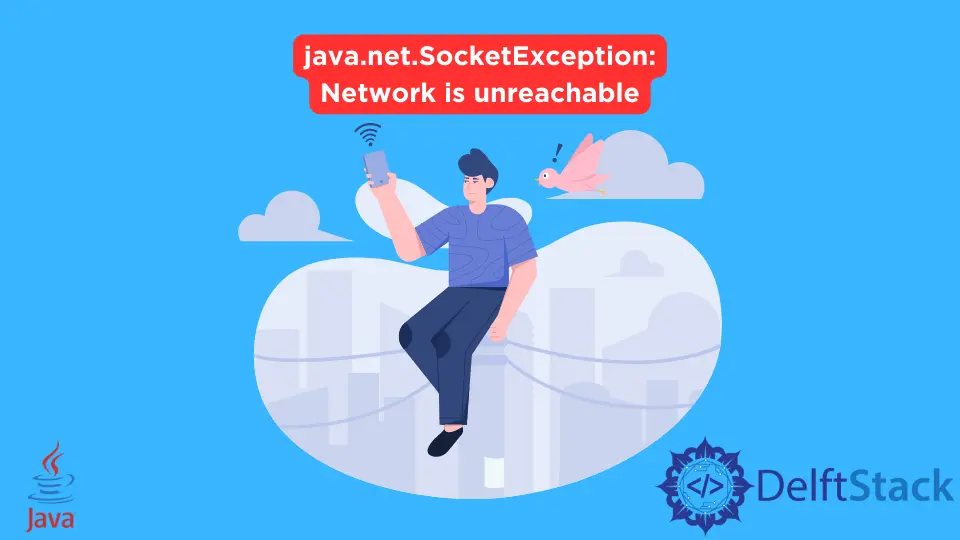
Today, we will discuss the possible reasons and solutions for the java.net.SocketException: Network is unreachable
exception while programming in Java.
Possible Reasons and Solution for java.net.SocketException: Network is unreachable
in Java
Example Code (Causing an Error):
// import required libraries
import java.io.*;
import java.net.URL;
// Main class
public class Main {
// download method
static void downloadXML(String webUrl, String file) throws IOException {
// create object
FileWriter xmlFileWriter;
xmlFileWriter = new FileWriter(file);
System.out.println("URL used for downloading the file is : " + webUrl);
// this statement throws an Exception
BufferedReader inputTextReader =
new BufferedReader(new BufferedReader(new InputStreamReader(new URL(webUrl).openStream())));
// create and initialize variables
String string;
String fileInString = "";
string = inputTextReader.readLine();
// read file
while (string != null) {
fileInString += (string + "\r\n");
string = inputTextReader.readLine();
}
// write file
xmlFileWriter.write(fileInString);
xmlFileWriter.flush();
xmlFileWriter.close();
System.out.println("The File is Downloaded");
} // end download() function
// main method
public static void main(String[] args) {
try {
downloadXML("https://www.cellml.org/Members/stevens/docs/sample.xml", "downloadXML.xml");
} catch (IOException exception) {
exception.printStackTrace();
}
} // end main
} // end Main class
In this code, we pass the URL
and the fileName
to the downloadXML()
method that reads the .xml
file from the specified URL
and writes it into the given fileName
, which is further saved on our local system.
Though this code example is syntactically and semantically correct but generates the java.net.SocketException: Network is unreachable
exception. The error is self-explanatory that tells us the network is not available at the current moment.
The reason causing this error is the connection breakdown. It can happen in Wi-Fi, 3G, or plain internet connection on the machine (computer/laptop).
Whenever we get this error, we must assume that the internet connection is not stable and may be lost from time to time while writing our application.
For instance, this happens with mobiles frequently when we are in the basements or tube, etc. It also happens while using apps on a PC/laptop, but it is less frequent.
The second reason can be incorrect Port
and/or HostName
. Make sure both are correct.
Additionally, you must remember two more things that can help in error identification.
-
First, you will get a
java.net.UnknownHostException
error if you are completely disconnected from the internet -
Usually, the
Network is unreachable
differs from theTimeout Error
. In theTimeout Error
, it can’t even find where it should go.For instance, there can be a difference between having our Wi-Fi card off and no Wi-Fi.
Firstly, perform the usual fiddling with the firewall to ensure that the required port is open. Then, have a look into the network issues that you might have.
Turn off the firewalls and eliminate the obstacles such as routers and complications to make it work in the simplest scenario possible since it is a network-related issue, not code related problem.