How to Fix Java.Net.UnknownHostException
-
Causes of the
java.net.UnknownHostException
in Java -
Prevent the
java.net.UnknownHostException
in Java
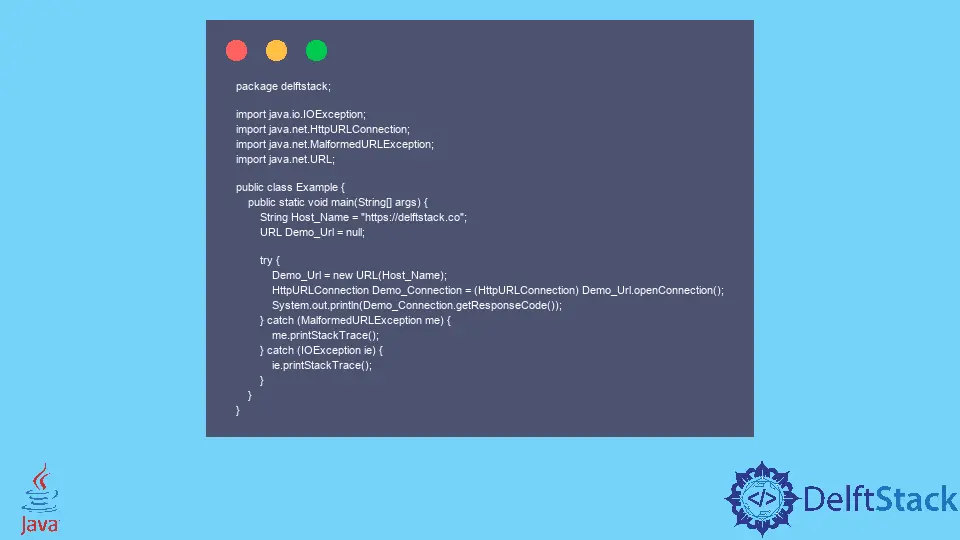
This tutorial demonstrates the java.net.UnknownHostException
error in Java.
Causes of the java.net.UnknownHostException
in Java
The java.net.UnknownHostException
means the IP address of the hostname cannot be found. Usually, this happens when there is a typing mistake in the hostname.
Another reason for this exception is DNS misconfiguration or DNS propagation. It might take 48 hours for a new DNS for all over internet propagation.
Here is an example of UnknownHostException
in Java:
package delftstack;
import java.io.IOException;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
public class Example {
public static void main(String[] args) {
String Host_Name = "https://delftstack.co";
URL Demo_Url = null;
try {
Demo_Url = new URL(Host_Name);
HttpURLConnection Demo_Connection = (HttpURLConnection) Demo_Url.openConnection();
System.out.println(Demo_Connection.getResponseCode());
} catch (MalformedURLException me) {
me.printStackTrace();
} catch (IOException ie) {
ie.printStackTrace();
}
}
}
The above tries to connect to a host, but the hostname has a typing mistake. It will throw the UnknownHostException
.
See output:
java.net.UnknownHostException: delftstack.co
at java.base/sun.nio.ch.NioSocketImpl.connect(NioSocketImpl.java:567)
at java.base/java.net.SocksSocketImpl.connect(SocksSocketImpl.java:327)
at java.base/java.net.Socket.connect(Socket.java:633)
at java.base/sun.security.ssl.SSLSocketImpl.connect(SSLSocketImpl.java:299)
at java.base/sun.security.ssl.BaseSSLSocketImpl.connect(BaseSSLSocketImpl.java:174)
at java.base/sun.net.NetworkClient.doConnect(NetworkClient.java:183)
at java.base/sun.net.www.http.HttpClient.openServer(HttpClient.java:498)
at java.base/sun.net.www.http.HttpClient.openServer(HttpClient.java:603)
at java.base/sun.net.www.protocol.https.HttpsClient.<init>(HttpsClient.java:266)
at java.base/sun.net.www.protocol.https.HttpsClient.New(HttpsClient.java:380)
at java.base/sun.net.www.protocol.https.AbstractDelegateHttpsURLConnection.getNewHttpClient(AbstractDelegateHttpsURLConnection.java:189)
at java.base/sun.net.www.protocol.http.HttpURLConnection.plainConnect0(HttpURLConnection.java:1242)
at java.base/sun.net.www.protocol.http.HttpURLConnection.plainConnect(HttpURLConnection.java:1128)
at java.base/sun.net.www.protocol.https.AbstractDelegateHttpsURLConnection.connect(AbstractDelegateHttpsURLConnection.java:175)
at java.base/sun.net.www.protocol.http.HttpURLConnection.getInputStream0(HttpURLConnection.java:1665)
at java.base/sun.net.www.protocol.http.HttpURLConnection.getInputStream(HttpURLConnection.java:1589)
at java.base/java.net.HttpURLConnection.getResponseCode(HttpURLConnection.java:529)
at java.base/sun.net.www.protocol.https.HttpsURLConnectionImpl.getResponseCode(HttpsURLConnectionImpl.java:308)
at delftstack.Example.main(Example.java:16)
The java.net.UnknownHostException
is a checked exception and can be handled using the try-catch
blocks. See below how to handle the UnknownHostException
in Java:
package delftstack;
import java.io.IOException;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
import java.net.UnknownHostException;
public class Example {
public static void main(String[] args) {
String Host_Name = "https://delftstack.co";
URL Demo_Url = null;
try {
Demo_Url = new URL(Host_Name);
HttpURLConnection Demo_Connection = (HttpURLConnection) Demo_Url.openConnection();
System.out.println(Demo_Connection.getResponseCode());
} catch (UnknownHostException ue) {
ue.printStackTrace();
} catch (MalformedURLException me) {
me.printStackTrace();
} catch (IOException ie) {
ie.printStackTrace();
}
}
}
Prevent the java.net.UnknownHostException
in Java
To prevent the java.net.UnknownHostException
in Java, we should consider the following two points:
- First, ensure the hostname is correct and doesn’t have any typing mistakes - double-check for any typing mistakes or white spaces.
- Check the DNS setting to ensure that the server is reachable. The new hostname will take time to catch up on the DNS server.
Let’s try the same example with the correct hostname. See example:
package delftstack;
import java.io.IOException;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
import java.net.UnknownHostException;
public class Example {
public static void main(String[] args) {
String Host_Name = "http://localhost/";
URL Demo_Url = null;
try {
Demo_Url = new URL(Host_Name);
HttpURLConnection Demo_Connection = (HttpURLConnection) Demo_Url.openConnection();
System.out.println(Demo_Connection.getResponseCode());
} catch (UnknownHostException uhe) {
uhe.printStackTrace();
} catch (MalformedURLException me) {
me.printStackTrace();
} catch (IOException ie) {
ie.printStackTrace();
}
}
}
The code above will work correctly and print the response code. See output:
200
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn FacebookRelated Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack