Java.Net.UnknownHostException
-
Ursachen der
java.net.UnknownHostException
in Java -
Verhindern Sie die
java.net.UnknownHostException
in Java
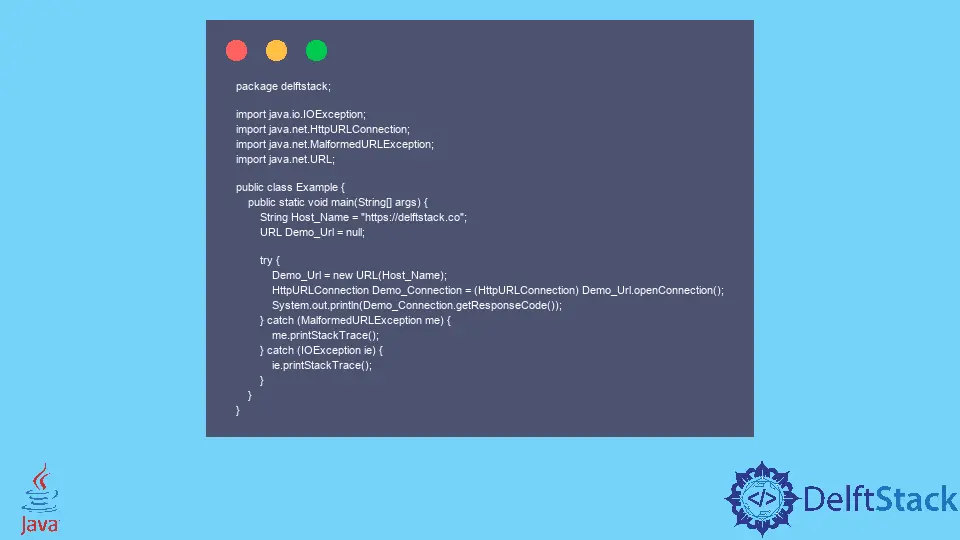
Dieses Tutorial demonstriert den Fehler java.net.UnknownHostException
in Java.
Ursachen der java.net.UnknownHostException
in Java
Die java.net.UnknownHostException
bedeutet, dass die IP-Adresse des Hostnamens nicht gefunden werden kann. Normalerweise passiert dies, wenn ein Tippfehler im Hostnamen vorliegt.
Ein weiterer Grund für diese Ausnahme ist eine DNS-Fehlkonfiguration oder DNS-Propagation. Es kann 48 Stunden dauern, bis ein neues DNS für die gesamte Internetverbreitung erstellt wird.
Hier ist ein Beispiel für UnknownHostException
in Java:
package delftstack;
import java.io.IOException;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
public class Example {
public static void main(String[] args) {
String Host_Name = "https://delftstack.co";
URL Demo_Url = null;
try {
Demo_Url = new URL(Host_Name);
HttpURLConnection Demo_Connection = (HttpURLConnection) Demo_Url.openConnection();
System.out.println(Demo_Connection.getResponseCode());
} catch (MalformedURLException me) {
me.printStackTrace();
} catch (IOException ie) {
ie.printStackTrace();
}
}
}
Das obige versucht, eine Verbindung zu einem Host herzustellen, aber der Hostname hat einen Tippfehler. Es wird die UnknownHostException
auslösen.
Siehe Ausgabe:
java.net.UnknownHostException: delftstack.co
at java.base/sun.nio.ch.NioSocketImpl.connect(NioSocketImpl.java:567)
at java.base/java.net.SocksSocketImpl.connect(SocksSocketImpl.java:327)
at java.base/java.net.Socket.connect(Socket.java:633)
at java.base/sun.security.ssl.SSLSocketImpl.connect(SSLSocketImpl.java:299)
at java.base/sun.security.ssl.BaseSSLSocketImpl.connect(BaseSSLSocketImpl.java:174)
at java.base/sun.net.NetworkClient.doConnect(NetworkClient.java:183)
at java.base/sun.net.www.http.HttpClient.openServer(HttpClient.java:498)
at java.base/sun.net.www.http.HttpClient.openServer(HttpClient.java:603)
at java.base/sun.net.www.protocol.https.HttpsClient.<init>(HttpsClient.java:266)
at java.base/sun.net.www.protocol.https.HttpsClient.New(HttpsClient.java:380)
at java.base/sun.net.www.protocol.https.AbstractDelegateHttpsURLConnection.getNewHttpClient(AbstractDelegateHttpsURLConnection.java:189)
at java.base/sun.net.www.protocol.http.HttpURLConnection.plainConnect0(HttpURLConnection.java:1242)
at java.base/sun.net.www.protocol.http.HttpURLConnection.plainConnect(HttpURLConnection.java:1128)
at java.base/sun.net.www.protocol.https.AbstractDelegateHttpsURLConnection.connect(AbstractDelegateHttpsURLConnection.java:175)
at java.base/sun.net.www.protocol.http.HttpURLConnection.getInputStream0(HttpURLConnection.java:1665)
at java.base/sun.net.www.protocol.http.HttpURLConnection.getInputStream(HttpURLConnection.java:1589)
at java.base/java.net.HttpURLConnection.getResponseCode(HttpURLConnection.java:529)
at java.base/sun.net.www.protocol.https.HttpsURLConnectionImpl.getResponseCode(HttpsURLConnectionImpl.java:308)
at delftstack.Example.main(Example.java:16)
Die java.net.UnknownHostException
ist eine geprüfte Ausnahme und kann mit den try-catch
-Blöcken behandelt werden. Sehen Sie unten, wie Sie mit der UnknownHostException
in Java umgehen:
package delftstack;
import java.io.IOException;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
import java.net.UnknownHostException;
public class Example {
public static void main(String[] args) {
String Host_Name = "https://delftstack.co";
URL Demo_Url = null;
try {
Demo_Url = new URL(Host_Name);
HttpURLConnection Demo_Connection = (HttpURLConnection) Demo_Url.openConnection();
System.out.println(Demo_Connection.getResponseCode());
} catch (UnknownHostException ue) {
ue.printStackTrace();
} catch (MalformedURLException me) {
me.printStackTrace();
} catch (IOException ie) {
ie.printStackTrace();
}
}
}
Verhindern Sie die java.net.UnknownHostException
in Java
Um die java.net.UnknownHostException
in Java zu verhindern, sollten wir folgende zwei Punkte beachten:
- Stellen Sie zunächst sicher, dass der Hostname korrekt ist und keine Tippfehler enthält – überprüfen Sie ihn nochmals auf Tippfehler oder Leerzeichen.
- Überprüfen Sie die DNS-Einstellung, um sicherzustellen, dass der Server erreichbar ist. Der neue Hostname wird einige Zeit brauchen, um den DNS-Server einzuholen.
Versuchen wir dasselbe Beispiel mit dem richtigen Hostnamen. Siehe Beispiel:
package delftstack;
import java.io.IOException;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
import java.net.UnknownHostException;
public class Example {
public static void main(String[] args) {
String Host_Name = "http://localhost/";
URL Demo_Url = null;
try {
Demo_Url = new URL(Host_Name);
HttpURLConnection Demo_Connection = (HttpURLConnection) Demo_Url.openConnection();
System.out.println(Demo_Connection.getResponseCode());
} catch (UnknownHostException uhe) {
uhe.printStackTrace();
} catch (MalformedURLException me) {
me.printStackTrace();
} catch (IOException ie) {
ie.printStackTrace();
}
}
}
Der obige Code wird korrekt funktionieren und den Antwortcode drucken. Siehe Ausgabe:
200
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn FacebookVerwandter Artikel - Java Error
- Adresse wird bereits verwendet JVM_Bind-Fehler in Java
- Android Java.Lang.IllegalStateException behoben: Methode der Aktivität konnte nicht ausgeführt werden
- Ausnahme im Hauptthread Java.Lang.ClassNotFoundException in IntelliJ IDEA
- Ausnahme im Hauptthread Java.Lang.NoClassDefFoundError
- Beheben Sie das Problem, dass Java nicht installiert werden kann. Es gibt Fehler in den folgenden Schaltern
- Beheben Sie den Fehler `Es wurde keine Java Virtual Machine gefunden` in Eclipse