Java Multiline Strings
- Various Methods for Java Multiline String
-
Use
Text Blocks
for Multiline String in Java -
Use
+
for Multiline String in Java -
Use the
getProperty()
andconcat()
Function for Multiline String in Java -
Use the
format()
Method ofString
Class for Multiline String in Java -
Use the
join()
Method ofString
Class for Multiline String in Java -
Use the
append()
Method ofStringBuilder
Class for Multiline String in Java
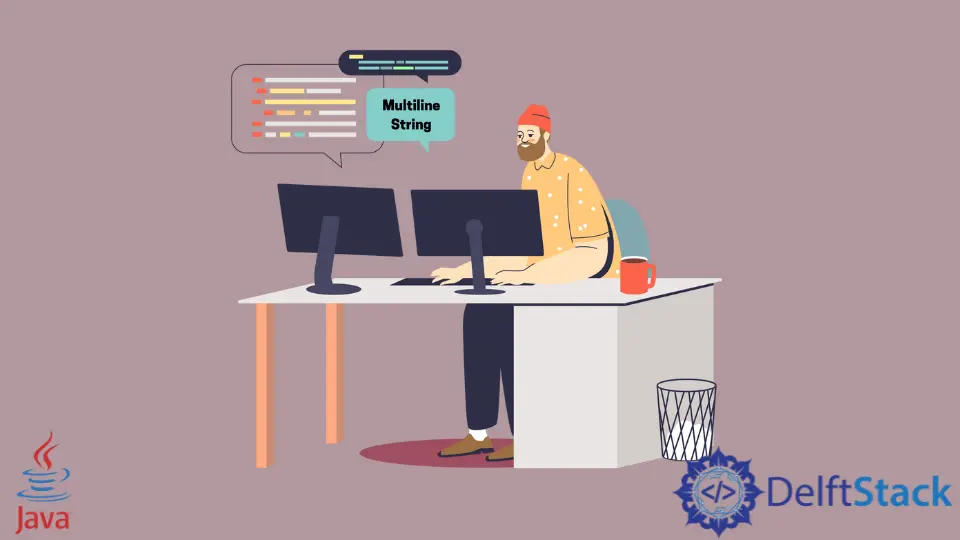
We aim to explore different approaches that are good for Java multiline strings. We’ll also see which method is suitable for an array of strings.
Various Methods for Java Multiline String
We have different ways to meet the goal of writing multiline strings in java. All of them are listed below, and you use any of them considering your project needs.
- Use
Text Blocks
(the three double quotes"""
). - Use the
+
sign. - Use
getProperty()
andconcat()
method. - Use the String class’s
format()
method. - Use the String class’s
join()
method. - Use
StringBuilder()
with an array of strings.
Use Text Blocks
for Multiline String in Java
Example Code:
public class MainClass {
public static void main(String args[]) {
String str =
"" "
This is line one.This is line two.This is line three.This is line four.This is line
five."" ";
System.out.println(str);
}
}
Output:
This is line one.
This is line two.
This is line three.
This is line four.
This is line five.
Java 15 brings a new way to write multiline strings using Text Blocks
, and we can also use it in Java 13 & 14 if the preview feature is enabled. The major advantage of using Text Blocks
is that we don’t need to specify escape sequences and concatenation operators.
It also reads white spaces and adds them to the output. You may find using Text Blocks
useful while inserting code blocks because it indents the tags by reading white spaces.
Example Code:
public class MainClass {
public static void main(String args[]) {
String str = "" "
< html > <head><title> Learning Java Multiline Strings</ title></ head><body>
<h1> Java Multiline String</ h1></ body></ html> "" ";
System.out.println(str);
}
}
Output:
<html>
<head>
<title> Learning Java Multiline Strings </title>
</head>
<body>
<h1> Java Multiline String </h1>
</body>
</html>
Use +
for Multiline String in Java
Example Code:
public class MainClass {
public static void main(String args[]) {
String str = "This is line one. \n"
+ "This is line two. \n"
+ "This is line three. \n"
+ "This is line four. \n"
+ "This is line five. \n";
System.out.println(str);
}
}
Output:
This is line one.
This is line two.
This is line three.
This is line four.
This is line five.
We write multiple strings (one string per line) and concatenate them with the +
symbol. We also specify a new line using \n
before closing each string.
If we type the string on multiple lines but forget to write \n
(used for the new line), the output will look as follows.
Output:
This is line one.This is line two.This is line three.This is line four.This is line five.
Use the getProperty()
and concat()
Function for Multiline String in Java
Example Code:
public class MainClass {
public static void main(String args[]) {
String newLine = System.getProperty("line.separator");
String str = "This is line one.".concat(newLine)
.concat("This is line two.")
.concat(newLine)
.concat("This is line three.")
.concat(newLine)
.concat("This is line four.")
.concat(newLine)
.concat("This is line five.");
System.out.println(str);
}
}
Output:
This is line one.
This is line two.
This is line three.
This is line four.
This is line five.
We can use a method getProperty()
of java.lang.System
Class to get a line separator in Java programming. The getProperty()
method takes the property’s key and returns the system property, denoted by the given key (passed as the argument).
Further, we use the concat()
method that appends one string to the end of the other string. Here, it concatenates the new line with the first string, and then the second string is concatenated with the new line and so on.
Use the format()
Method of String
Class for Multiline String in Java
Example Code:
public class MainClass {
public static void main(String args[]) {
String str = String.format("%s\n%s\n%s\n%s\n%s\n", "This is line one.", "This is line two.",
"This is line three.", "This is line four.", "This is line five.");
System.out.println(str);
}
}
Output:
This is line one.
This is line two.
This is line three.
This is line four.
This is line five.
In this instance, we use the format()
method that formats the string as specified. Although it works fine, we don’t recommend this function because it is hard to manage and keep the count of %s
and \n
.
Use the join()
Method of String
Class for Multiline String in Java
Example Code:
public class MainClass {
public static void main(String args[]) {
String str = String.join("\n", "This is line one.", "This is line two.", "This is line three.",
"This is line four.", "This is line five.");
System.out.println(str);
}
}
Output:
This is line one.
This is line two.
This is line three.
This is line four.
This is line five.
The join()
method seems cleaner than the format()
method. The join()
method uses the given delimiter to join the given string(s).
We are using \n
as a delimiter for this tutorial. You may use a comma, period, or anything else you want.
Use the append()
Method of StringBuilder
Class for Multiline String in Java
Example Code:
public class MainClass {
public static void main(String args[]) {
String newLine = System.getProperty("line.separator");
StringBuilder string = new StringBuilder();
String array[] = {"This is line one.", "This is line two.", "This is line three.",
"This is line four.", "This is line five."};
for (int i = 0; i < array.length; i++) {
string.append(array[i]);
string.append(newLine);
}
System.out.println(string.toString());
}
}
Output:
This is line one.
This is line two.
This is line three.
This is line four.
This is line five.
The StringBuilder
class is very useful if we are using an array of strings. It is used to modifiable (mutable) strings.
Then, we use the append()
method to append strings that reside in the array. Finally, we print the string on the screen using the toString()
method that converts any object to the string.