Java 多行字串
- Java 多行字串的各種方法
-
在 Java 中對多行字串使用
Text Blocks
-
在 Java 中使用
+
表示多行字串 -
在 Java 中對多行字串使用
getProperty()
和concat()
函式 -
在 Java 中使用
String
類的format()
方法處理多行字串 -
在 Java 中使用
String
類的join()
方法處理多行字串 -
使用
StringBuilder
類的append()
方法用於 Java 中的多行字串
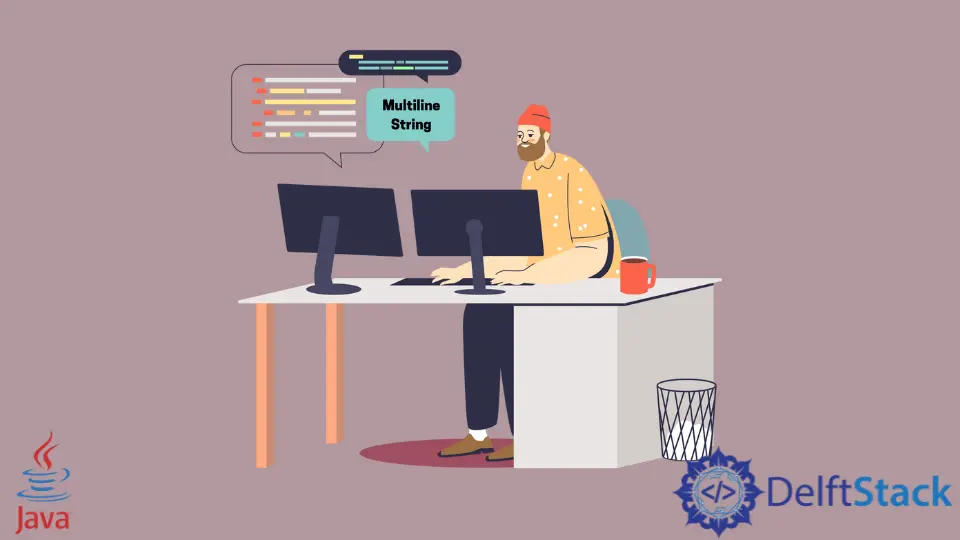
我們旨在探索適用於 Java 多行字串的不同方法。我們還將看到哪種方法適合字串陣列。
Java 多行字串的各種方法
我們有不同的方法來實現在 java 中編寫多行字串的目標。所有這些都在下面列出,你可以根據你的專案需求使用它們中的任何一個。
- 使用文字塊(三個雙引號
"""
)。 - 使用
+
號。 - 使用
getProperty()
和concat()
方法。 - 使用 String 類的
format()
方法。 - 使用 String 類的
join()
方法。 - 對字串陣列使用
StringBuilder()
。
在 Java 中對多行字串使用 Text Blocks
示例程式碼:
public class MainClass {
public static void main(String args[]) {
String str =
"" "
This is line one.This is line two.This is line three.This is line four.This is line
five."" ";
System.out.println(str);
}
}
輸出:
This is line one.
This is line two.
This is line three.
This is line four.
This is line five.
Java 15 帶來了一種使用 Text Blocks
編寫多行字串的新方法,如果啟用了預覽功能,我們也可以在 Java 13 和 14 中使用它。使用 Text Blocks
的主要優點是我們不需要指定轉義序列和連線運算子。
它還讀取空格並將它們新增到輸出中。你可能會發現在插入程式碼塊時使用 Text Blocks
很有用,因為它通過讀取空格來縮排標籤。
示例程式碼:
public class MainClass {
public static void main(String args[]) {
String str = "" "
< html > <head><title> Learning Java Multiline Strings</ title></ head><body>
<h1> Java Multiline String</ h1></ body> < / html > "" ";
System.out.println(str);
}
}
輸出:
<html>
<head>
<title> Learning Java Multiline Strings </title>
</head>
<body>
<h1> Java Multiline String </h1>
</body>
</html>
在 Java 中使用 +
表示多行字串
示例程式碼:
public class MainClass {
public static void main(String args[]) {
String str = "This is line one. \n"
+ "This is line two. \n"
+ "This is line three. \n"
+ "This is line four. \n"
+ "This is line five. \n";
System.out.println(str);
}
}
輸出:
This is line one.
This is line two.
This is line three.
This is line four.
This is line five.
我們編寫多個字串(每行一個字串)並用 +
符號連線它們。在關閉每個字串之前,我們還使用 \n
指定一個新行。
如果我們在多行上鍵入字串但忘記寫\n
(用於新行),輸出將如下所示。
輸出:
This is line one.This is line two.This is line three.This is line four.This is line five.
在 Java 中對多行字串使用 getProperty()
和 concat()
函式
示例程式碼:
public class MainClass {
public static void main(String args[]) {
String newLine = System.getProperty("line.separator");
String str = "This is line one.".concat(newLine)
.concat("This is line two.")
.concat(newLine)
.concat("This is line three.")
.concat(newLine)
.concat("This is line four.")
.concat(newLine)
.concat("This is line five.");
System.out.println(str);
}
}
輸出:
This is line one.
This is line two.
This is line three.
This is line four.
This is line five.
我們可以使用 java.lang.System
類的方法 getProperty()
來獲取 Java 程式設計中的行分隔符。getProperty()
方法獲取屬性的鍵並返回系統屬性,由給定鍵表示(作為引數傳遞)。
此外,我們使用 concat()
方法將一個字串附加到另一個字串的末尾。在這裡,它將新行與第一個字串連線起來,然後將第二個字串與新行連線起來,依此類推。
在 Java 中使用 String
類的 format()
方法處理多行字串
示例程式碼:
public class MainClass {
public static void main(String args[]) {
String str = String.format("%s\n%s\n%s\n%s\n%s\n", "This is line one.", "This is line two.",
"This is line three.", "This is line four.", "This is line five.");
System.out.println(str);
}
}
輸出:
This is line one.
This is line two.
This is line three.
This is line four.
This is line five.
在這種情況下,我們使用 format()
方法來格式化指定的字串。雖然它工作得很好,但我們不推薦這個功能,因為它很難管理和保持%s
和\n
的計數。
在 Java 中使用 String
類的 join()
方法處理多行字串
示例程式碼:
public class MainClass {
public static void main(String args[]) {
String str = String.join("\n", "This is line one.", "This is line two.", "This is line three.",
"This is line four.", "This is line five.");
System.out.println(str);
}
}
輸出:
This is line one.
This is line two.
This is line three.
This is line four.
This is line five.
join()
方法似乎比 format()
方法更乾淨。join()
方法使用給定的分隔符連線給定的字串。
我們使用 \n
作為本教程的分隔符。你可以使用逗號、句號或其他任何你想要的東西。
使用 StringBuilder
類的 append()
方法用於 Java 中的多行字串
示例程式碼:
public class MainClass {
public static void main(String args[]) {
String newLine = System.getProperty("line.separator");
StringBuilder string = new StringBuilder();
String array[] = {"This is line one.", "This is line two.", "This is line three.",
"This is line four.", "This is line five."};
for (int i = 0; i < array.length; i++) {
string.append(array[i]);
string.append(newLine);
}
System.out.println(string.toString());
}
}
輸出:
This is line one.
This is line two.
This is line three.
This is line four.
This is line five.
如果我們使用字串陣列,StringBuilder
類非常有用。它用於可修改(可變)的字串。
然後,我們使用 append()
方法來追加駐留在陣列中的字串。最後,我們使用將任何物件轉換為字串的 toString()
方法在螢幕上列印字串。