How to Get Length of Array in Java
- Using the Length Property
- Using a Loop to Count Elements
- Using Java Streams for Length
- Conclusion
- FAQ
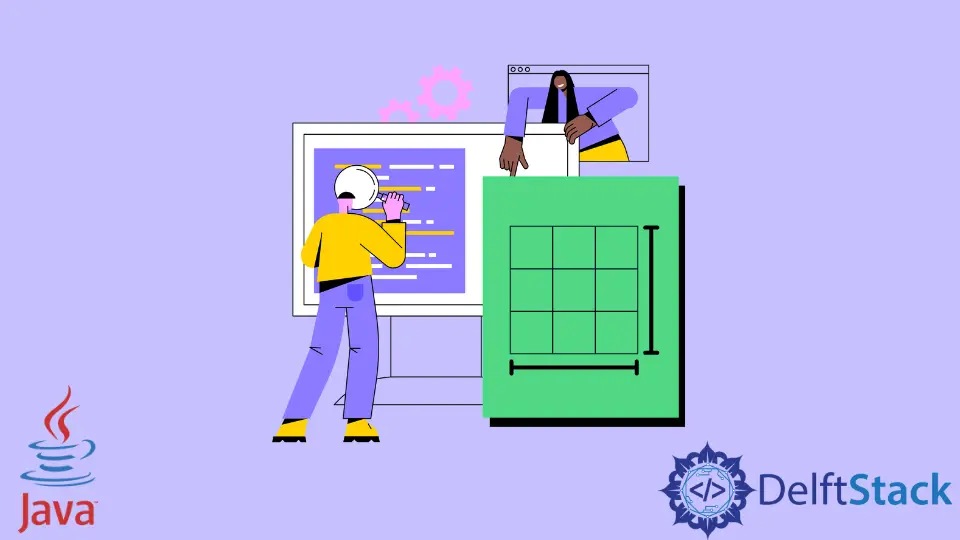
When working with arrays in Java, one common task you’ll encounter is determining the length of an array. Whether you’re processing data, manipulating collections, or simply trying to understand how many elements are in your array, knowing how to get its length is essential.
In this tutorial, we’ll explore various methods to retrieve the length of an array in Java. Understanding these techniques will not only enhance your coding skills but also improve your efficiency when working with data structures. So, let’s dive into the world of Java arrays and discover how to effectively get their lengths!
Using the Length Property
In Java, arrays have a built-in property called length
that allows you to easily obtain the number of elements contained within the array. This property is straightforward and does not require any additional methods or functions. Here’s how you can use it:
public class ArrayLengthExample {
public static void main(String[] args) {
int[] numbers = {1, 2, 3, 4, 5};
int length = numbers.length;
System.out.println("The length of the array is: " + length);
}
}
Output:
The length of the array is: 5
In this example, we declare an integer array named numbers
and initialize it with five elements. By accessing the length
property of the array, we store the value in the variable length
. Finally, we print out the length, which in this case is 5. This method is efficient and simple, making it the most commonly used way to get the length of an array in Java.
Using a Loop to Count Elements
While using the length
property is the most efficient way to get the length of an array, you might sometimes want to count the elements manually, especially in scenarios where you are dealing with dynamic data. Here’s how you can do it using a loop:
public class ArrayLengthWithLoop {
public static void main(String[] args) {
String[] fruits = {"Apple", "Banana", "Cherry", "Date"};
int count = 0;
for (String fruit : fruits) {
count++;
}
System.out.println("The length of the array is: " + count);
}
}
Output:
The length of the array is: 4
In this example, we have an array of strings called fruits
. We initialize a counter variable count
to zero. Using an enhanced for loop, we iterate through each element in the fruits
array and increment the count
variable for each element. At the end of the loop, we print out the total count, which gives us the length of the array. While this method is not as efficient as using the length
property, it can be useful in specific situations where you need to perform additional operations while counting.
Using Java Streams for Length
If you’re working with Java 8 or later, you can take advantage of the Stream API to determine the length of an array. This method is more functional in nature and can be very handy, especially when dealing with large datasets. Here’s how it works:
import java.util.Arrays;
public class ArrayLengthWithStreams {
public static void main(String[] args) {
double[] scores = {85.5, 90.0, 76.5, 88.0};
long length = Arrays.stream(scores).count();
System.out.println("The length of the array is: " + length);
}
}
Output:
The length of the array is: 4
In this code snippet, we use the Arrays.stream()
method to convert the scores
array into a stream. We then call the count()
method on the stream, which returns the number of elements in the array. This approach is particularly useful when working with more complex data manipulations, as the Stream API provides a wide range of methods for processing collections of data in a more functional style.
Conclusion
Understanding how to get the length of an array in Java is a fundamental skill for any programmer. In this tutorial, we explored three different methods: using the built-in length
property, counting elements with a loop, and utilizing Java Streams. Each method has its own advantages and use cases, so it’s beneficial to be familiar with all of them. Whether you’re developing applications or simply learning Java, mastering these techniques will enhance your coding capabilities. Happy coding!
FAQ
-
What is the difference between
length
andsize()
in Java?
Thelength
property is used to get the size of arrays, whilesize()
is a method used with collections like ArrayList. -
Can I change the length of an array in Java?
No, arrays in Java have a fixed length once they are created. To change the size, you would need to create a new array. -
Is it possible to get the length of a multidimensional array?
Yes, for a multidimensional array, you can use thelength
property on each dimension to get their respective lengths. -
What happens if I try to access an index outside the array length?
Attempting to access an index outside the bounds of an array will result in anArrayIndexOutOfBoundsException
. -
Are there any performance differences between these methods?
Yes, using thelength
property is the most efficient, while counting elements with a loop or using streams may introduce overhead.