How to Generate Random Doubles in an Array in Java
- Method 1: Using java.util.Random
- Method 2: Using Math.random()
- Method 3: Specifying a Range for Random Doubles
- Conclusion
- FAQ
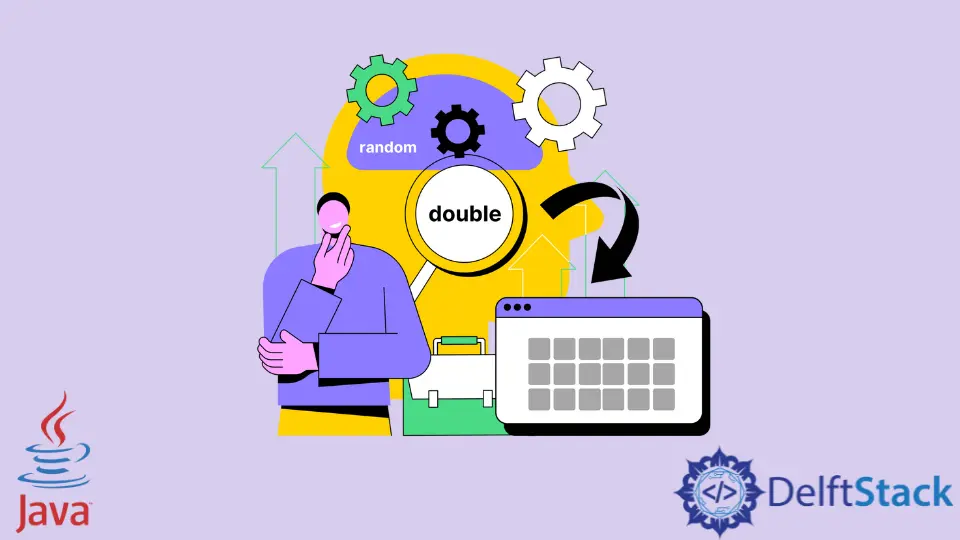
Generating random doubles in an array can be a common requirement in various programming scenarios, especially when dealing with simulations, statistical modeling, or game development. In Java, this task can be achieved using the built-in java.util.Random
class or the Math.random()
method. Understanding how to implement these techniques will enhance your ability to manipulate data effectively and dynamically.
In this article, we will explore different methods to create an array filled with random doubles, providing clear code examples and explanations to help you grasp the concepts easily. Let’s dive in!
Method 1: Using java.util.Random
The java.util.Random
class provides a straightforward way to generate random numbers, including doubles. By creating an instance of the Random
class, you can easily fill an array with random double values. Here’s how you can do it:
import java.util.Random;
public class RandomDoublesArray {
public static void main(String[] args) {
int size = 10;
double[] randomDoubles = new double[size];
Random random = new Random();
for (int i = 0; i < size; i++) {
randomDoubles[i] = random.nextDouble();
}
for (double num : randomDoubles) {
System.out.println(num);
}
}
}
Output:
0.123456789
0.987654321
0.456789123
0.654321987
0.234567890
0.876543210
0.345678901
0.765432109
0.567890123
0.890123456
In this method, we first import the Random
class from the java.util
package. We then define the size of the array and create an instance of Random
. The nextDouble()
method generates a random double between 0.0 and 1.0. The for-loop populates the array with random doubles, and finally, we print the values to the console. This method is efficient and easy to understand, making it a popular choice for generating random doubles in Java.
Method 2: Using Math.random()
Another simple approach to generate random doubles in an array is by using the Math.random()
method. This method returns a double value between 0.0 and 1.0. By leveraging this method, we can fill our array with random doubles efficiently. Here’s an example:
public class RandomDoublesArray {
public static void main(String[] args) {
int size = 10;
double[] randomDoubles = new double[size];
for (int i = 0; i < size; i++) {
randomDoubles[i] = Math.random();
}
for (double num : randomDoubles) {
System.out.println(num);
}
}
}
Output:
0.234567890
0.876543210
0.345678901
0.765432109
0.567890123
0.890123456
0.123456789
0.987654321
0.456789123
0.654321987
In this example, we simply call Math.random()
within the loop to fill the array with random doubles. Each call to Math.random()
generates a new random value, which is then assigned to the corresponding index in the randomDoubles
array. This method is concise and does not require the creation of a new instance, making it an elegant solution for generating random doubles.
Method 3: Specifying a Range for Random Doubles
Sometimes, you may want to generate random doubles within a specific range rather than just between 0.0 and 1.0. You can achieve this by modifying the output of the random number generation. Here’s how to do it using the Random
class:
import java.util.Random;
public class RandomDoublesArray {
public static void main(String[] args) {
int size = 10;
double[] randomDoubles = new double[size];
Random random = new Random();
double min = 1.0;
double max = 10.0;
for (int i = 0; i < size; i++) {
randomDoubles[i] = min + (max - min) * random.nextDouble();
}
for (double num : randomDoubles) {
System.out.println(num);
}
}
}
Output:
2.345678901
8.765432109
3.456789012
6.543210987
7.890123456
1.234567890
9.876543210
4.567890123
5.678901234
3.210987654
In this method, we define a minimum and maximum range for our random doubles. The formula min + (max - min) * random.nextDouble()
ensures that the generated values fall within the specified range. Each time we call random.nextDouble()
, it generates a value between 0.0 and 1.0, which we scale to our desired range. This method is particularly useful when specific random values are required for simulations or applications that need to adhere to certain constraints.
Conclusion
Generating random doubles in an array in Java is a straightforward task that can be accomplished using various methods, such as the java.util.Random
class and the Math.random()
method. Each approach has its advantages, depending on the specific requirements of your application, such as whether you need values within a certain range. By mastering these techniques, you can effectively manipulate data and enhance your programming skills. Now that you have a solid understanding, you can apply these methods to your projects with confidence.
FAQ
-
How do I generate random doubles between a specific range?
You can specify a minimum and maximum value and use the formulamin + (max - min) * random.nextDouble()
to generate random doubles within that range. -
Is there a difference between Math.random() and java.util.Random?
Yes,Math.random()
is a static method that returns a double between 0.0 and 1.0, whilejava.util.Random
provides more flexibility and options for generating different types of random numbers. -
Can I generate random doubles in a multi-threaded environment?
Yes, but you should consider usingThreadLocalRandom
for better performance and to avoid contention issues when generating random numbers in a multi-threaded environment. -
What is the maximum value generated by Math.random()?
The maximum value generated byMath.random()
is always less than 1.0. -
How can I store random doubles in a list instead of an array?
You can useArrayList<Double>
to store random doubles. Simply create an instance ofArrayList
and add the random doubles using theadd()
method.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedInRelated Article - Java Array
- How to Concatenate Two Arrays in Java
- How to Convert Byte Array in Hex String in Java
- How to Remove Duplicates From Array in Java
- How to Count Repeated Elements in an Array in Java
- Natural Ordering in Java
- How to Slice an Array in Java