How to Find the Max Number in an Array in Java
- Find Maximum Number in an Array Using the Iterative Way
-
Find Maximum Number in an Array Using
Stream
-
Find Maximum Number in an Array Using
Arrays.sort()
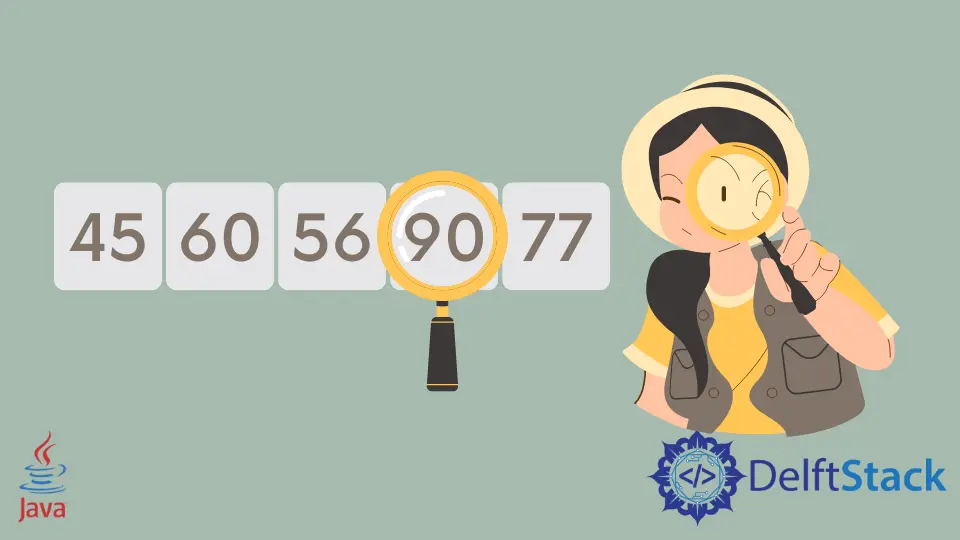
An array contains data of a similar type. While you can already read all the elements and perform several operations on them, this article will show you how to find the max value in an array in Java.
Find Maximum Number in an Array Using the Iterative Way
This method is the traditional way to find the maximum number from an array. It includes an iterator that is used to go through every element in the array. Below, we have an array of integers, intArray
; first, we create a variable maxNum
and initialize it with the first element of intArray
.
We create an enhanced for-loop that takes the array and returns every single element in each iteration. Then, we check each element with maxNum
that has 24, and once it finds a greater number than 24, it replaces 24 with that number in maxNum
. It will replace the number in maxNum
until it reaches the end of the array; otherwise, it didn’t find a bigger number than the existing value in maxNum
.
public class ArrayMax {
public static void main(String[] args) {
int[] intArray = {24, 2, 0, 34, 12, 110, 2};
int maxNum = intArray[0];
for (int j : intArray) {
if (j > maxNum)
maxNum = j;
}
System.out.println("Maximum number = " + maxNum);
}
}
Output:
Maximum number = 110
Find Maximum Number in an Array Using Stream
Java 8 introduced the Stream API
that provides several useful methods. One of them is the Arrays.stream()
method that takes an array and returns a sequential stream. In our case, we have an array of the int
type, and when we pass it in the stream, it returns an IntStream
.
The IntStream
function comes with a method max()
that helps to find the maximum value in the stream. It returns an OptionalInt
that describes that the stream might have empty int
values too.
At last, as we want the maximum number as an int
, we’ll use the optionalInt.getAsInt()
method that returns the result as an int
type.
import java.util.Arrays;
import java.util.OptionalInt;
import java.util.stream.IntStream;
public class ArrayMax {
public static void main(String[] args) {
int[] intArray = {24, 2, 0, 34, 12, 11, 2};
IntStream intStream = Arrays.stream(intArray);
OptionalInt optionalInt = intStream.max();
int maxAsInt = optionalInt.getAsInt();
System.out.println("Maximum number = " + maxAsInt);
}
}
Output:
Maximum number = 34
Find Maximum Number in an Array Using Arrays.sort()
The last technique in this list uses the sorting method that organizes the array in ascending order. To sort the array, we use the function Arrays.sort()
and pass intArray
as an argument.
To see how the array will look like after the sort operation, we print it. Now, as the array is sorted and the largest number of all is at the left-most position, we get its position using the intArray.length - 1
function, which is at the last position of the array.
import java.util.Arrays;
public class ArrayMax {
public static void main(String[] args) {
int[] intArray = {24, 340, 0, 34, 12, 10, 20};
Arrays.sort(intArray);
System.out.println("intArray after sorting: " + Arrays.toString(intArray));
int maxNum = intArray[intArray.length - 1];
System.out.println("Maximum number = " + maxNum);
}
}
Output:
intArray after sorting: [0, 10, 12, 20, 24, 34, 340]
Maximum number = 340
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn