How to Delete a File in Java
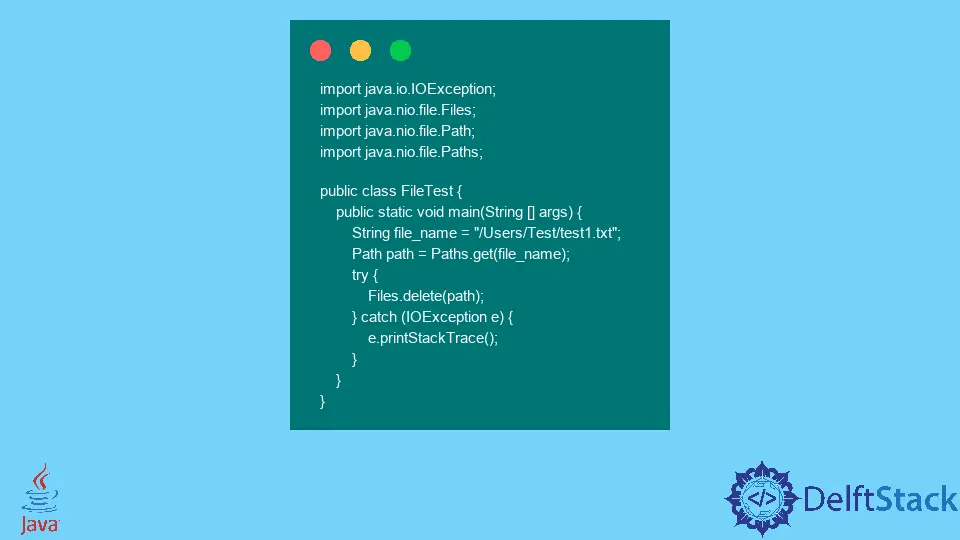
Java NIO
package was introduced in Java 7 version. It provides utility APIs named Files
to manipulate files and directions.
We will be using this package to delete files.
Delete a File Using Files.delete()
in Java
The java.nio.file
package includes the Java Files
class with many static methods to perform operations on files, directories, and other types.
We use the Path
interface java.nio.files.Path
to create a Path
instance. The Path
instance is created using the static method get()
inside the Paths
class (java.nio.file.Paths
).
Thus, calling Paths.get()
provides a Path
instance. This instance represents an object used to locate a file in the file system.
We pass the absolute path in a String
format as an argument to the static method get()
. The absolute path here in the code is /Users/Test/test1.txt
. The get() method converts a sequence of strings to form a path string.
The static method delete()
takes the Path
instance and deletes the specified path file. If the file does not exist at the specified path, this method will throw NoSuchFileException
.
If the file is a directory that is not empty and could not be deleted, it can also throw DirectoryNotEmptyException
. Thus, we placed the code inside a try-catch
block.
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class FileTest {
public static void main(String[] args) {
String file_name = "/Users/Test/test1.txt";
Path path = Paths.get(file_name);
try {
Files.delete(path);
} catch (IOException e) {
e.printStackTrace();
}
}
}
Delete a File Using Files.deleteIfExists()
in Java
The Files
class inside the java.nio.file
package also includes the method deleteIfExists()
, which deletes a file if that file exists at the specified path. This method returns a boolean value.
If the returned value is true
, this file is deleted as it existed at the path. If the files do not exist at that location, this method returns false
as it could not be deleted.
We store the boolean in the variable result and print outputs accordingly. As discussed in the above section, we placed the code inside a try-catch
block as this operation may throw IOException
.
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
class FileTest {
public static void main(String[] args) {
String file_name = "/Users/Test/test.txt";
Path path = Paths.get(file_name);
try {
boolean result = Files.deleteIfExists(path);
if (result) {
System.out.println("File is deleted!");
} else {
System.out.println("Sorry, could not delete the file.");
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output:
File is deleted!
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn