How to Zip Files in Java
-
Use
Java.Util.Zip
to Zip a Single File in Java -
Use
Java.Util.Zip
to Zip Multiple Files in Java -
Use
Java.Util.Zip
to Zip a Folder in Java
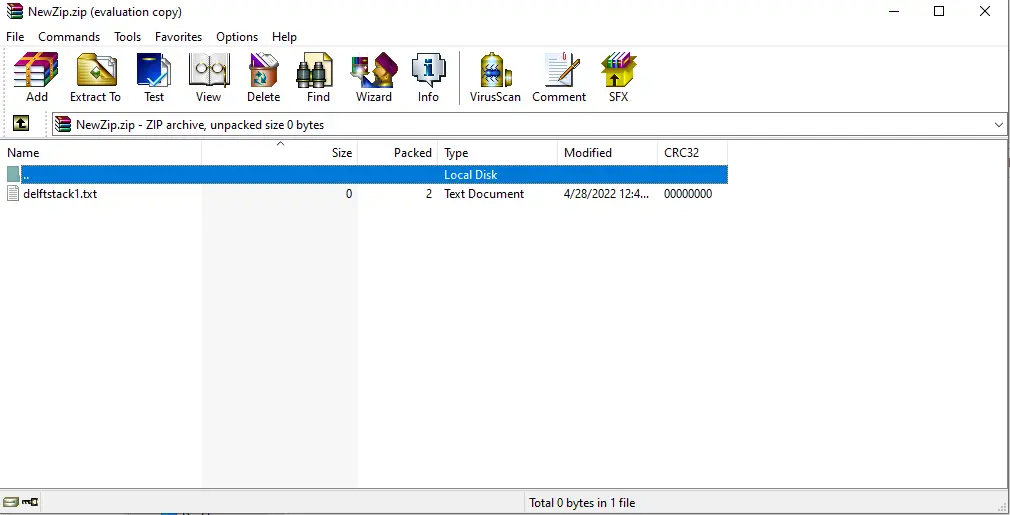
A zip file can consist of a single file, multiple files, or even a folder. There are different ways to create a zip file.
This tutorial demonstrates different examples of creating a zip file in Java.
Use Java.Util.Zip
to Zip a Single File in Java
We can use the java.util.zip.ZipOutputStream
class to zip a single file in Java. See the example below:
package delftstack;
import java.io.*;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.zip.ZipEntry;
import java.util.zip.ZipOutputStream;
public class Create_Zip {
public static void main(String[] args) {
Path Source_File =
Paths.get("C:\\Users\\Sheeraz\\OneDrive\\Desktop\\ZipFiles\\delftstack1.txt");
String Output_Zip = "C:\\Users\\Sheeraz\\OneDrive\\Desktop\\ZipFiles\\NewZip.zip";
try {
Create_Zip.Create_Zip_File(Source_File, Output_Zip);
System.out.println("Zip File Created");
} catch (IOException e) {
e.printStackTrace();
}
}
// method to zip a single file
public static void Create_Zip_File(Path Source_File, String Output_Zip) throws IOException {
if (!Files.isRegularFile(Source_File)) {
System.err.println("File Not Found.");
return;
}
try (ZipOutputStream Zip_Output_Stream = new ZipOutputStream(new FileOutputStream(Output_Zip));
FileInputStream File_Input_Stream = new FileInputStream(Source_File.toFile());) {
ZipEntry Zip_Entry = new ZipEntry(Source_File.getFileName().toString());
Zip_Output_Stream.putNextEntry(Zip_Entry);
byte[] Buffer = new byte[1024];
int Length;
while ((Length = File_Input_Stream.read(Buffer)) > 0) {
Zip_Output_Stream.write(Buffer, 0, Length);
}
Zip_Output_Stream.closeEntry();
}
}
}
The code above will create a zip file with the single file delftstack1
only.
Output:
Zip File Created
Use Java.Util.Zip
to Zip Multiple Files in Java
Similarly, we can use java.util.zip.ZipOutputStream
to add multiple files to a zip file. Multiple files are added one by one to the zip file.
See the following example:
package delftstack;
import java.io.BufferedInputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.zip.ZipEntry;
import java.util.zip.ZipOutputStream;
public class Create_Zip {
public static void main(String[] args) {
try {
// The Source file paths
String Source_File1 = "C:\\Users\\Sheeraz\\OneDrive\\Desktop\\ZipFiles\\delftstack1.txt";
String Source_File2 = "C:\\Users\\Sheeraz\\OneDrive\\Desktop\\ZipFiles\\\\delftstack2.txt";
// Output Zipped file path
String Output_Zip_Path = "C:\\Users\\Sheeraz\\OneDrive\\Desktop\\ZipFiles\\NewZip.zip";
File Output_Zip = new File(Output_Zip_Path);
FileOutputStream File_Output_Stream = new FileOutputStream(Output_Zip);
ZipOutputStream Zip_Output_Stream = new ZipOutputStream(File_Output_Stream);
Create_Zip_File(Source_File1, Zip_Output_Stream);
Create_Zip_File(Source_File2, Zip_Output_Stream);
Zip_Output_Stream.close();
System.out.println("Zip File Created");
} catch (IOException e) {
e.printStackTrace();
}
}
// Method to create the zip file
private static void Create_Zip_File(String Source_File, ZipOutputStream Zip_Output_Stream)
throws IOException {
final int BUFFER = 1024;
BufferedInputStream Buffered_Input_Stream = null;
try {
File file = new File(Source_File);
FileInputStream File_Input_Stream = new FileInputStream(file);
Buffered_Input_Stream = new BufferedInputStream(File_Input_Stream, BUFFER);
ZipEntry Zip_Entry = new ZipEntry(file.getName());
Zip_Output_Stream.putNextEntry(Zip_Entry);
byte Data[] = new byte[BUFFER];
int Length;
while ((Length = Buffered_Input_Stream.read(Data, 0, BUFFER)) != -1) {
Zip_Output_Stream.write(Data, 0, Length);
}
// close the entry
Zip_Output_Stream.closeEntry();
} finally {
try {
Buffered_Input_Stream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
The code above will add multiple files to a zip file.
Output:
Zip File Created
Use Java.Util.Zip
to Zip a Folder in Java
The Java.Util.Zip
can also zip a folder or directory, including all subfolders and files. See the example below:
package delftstack;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.nio.file.*;
import java.nio.file.attribute.BasicFileAttributes;
import java.util.zip.ZipEntry;
import java.util.zip.ZipOutputStream;
public class Create_Zip {
public static void main(String[] args) {
Path Source_Folder = Paths.get("C:\\Users\\Sheeraz\\OneDrive\\Desktop\\ZipFiles\\Folder");
if (!Files.isDirectory(Source_Folder)) {
System.out.println("Please provide the right folder.");
return;
}
try {
Create_Zip.Create_Zip_Folder(Source_Folder);
System.out.println("Folder is zipped");
} catch (IOException e) {
e.printStackTrace();
}
}
// zip a directory or folder
public static void Create_Zip_Folder(Path Source_Folder) throws IOException {
// get folder name as zip file name
String Output_Zip = Source_Folder.getFileName().toString() + ".zip";
try (
ZipOutputStream Zip_Output_Stream = new ZipOutputStream(new FileOutputStream(Output_Zip))) {
Files.walkFileTree(Source_Folder, new SimpleFileVisitor<>() {
@Override
public FileVisitResult visitFile(Path File, BasicFileAttributes File_Attributes) {
// only copy files
if (File_Attributes.isSymbolicLink()) {
return FileVisitResult.CONTINUE;
}
try (FileInputStream File_Input_Stream = new FileInputStream(File.toFile())) {
Path targetFile = Source_Folder.relativize(File);
Zip_Output_Stream.putNextEntry(new ZipEntry(targetFile.toString()));
byte[] BUFFER = new byte[1024];
int Length;
while ((Length = File_Input_Stream.read(BUFFER)) > 0) {
Zip_Output_Stream.write(BUFFER, 0, Length);
}
Zip_Output_Stream.closeEntry();
System.out.printf("Zipped file : %s%n", File);
} catch (IOException e) {
e.printStackTrace();
}
return FileVisitResult.CONTINUE;
}
@Override
public FileVisitResult visitFileFailed(Path File, IOException exception) {
System.err.printf("Unable to zip the file : %s%n%s%n", File, exception);
return FileVisitResult.CONTINUE;
}
});
}
}
}
The code above will zip the given folder.
Output:
Zipped file : C:\Users\Sheeraz\OneDrive\Desktop\ZipFiles\Folder\delftstack1.txt
Zipped file : C:\Users\Sheeraz\OneDrive\Desktop\ZipFiles\Folder\delftstack2.txt
Folder is zipped
There are also other ways like the file system or zip4j that can zip files or folders. But, the Java.Util.Zip
is considered the most efficient way to zip files and folders.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook