How to Create File if Not Exists in Java
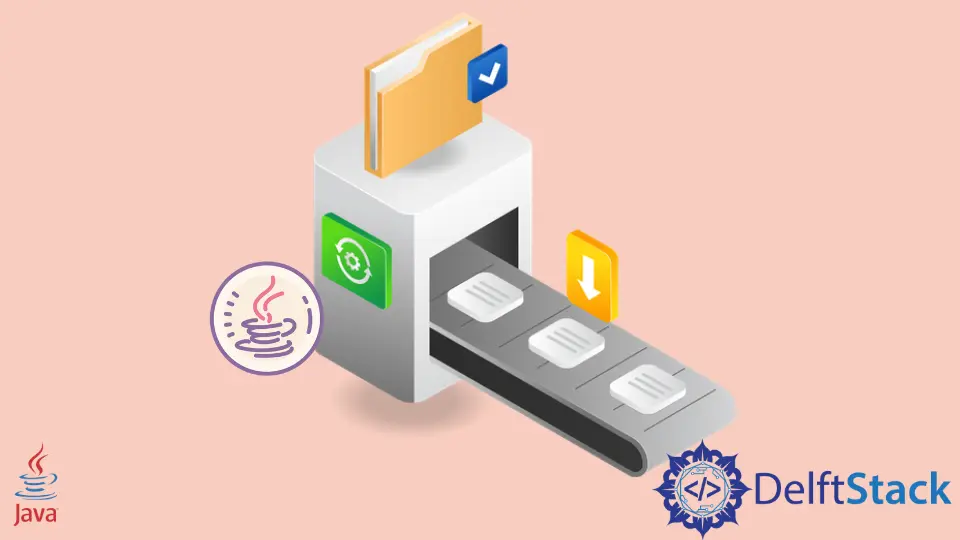
Creating files is a common task in programming, and Java provides a straightforward way to handle it. If you’re looking to create a file only when it doesn’t already exist, you’ve come to the right place.
In this tutorial, we’ll explore various methods to achieve this in Java. Whether you’re a beginner or an experienced developer, understanding how to manage files effectively is crucial for building robust applications. We’ll cover the use of File
, FileOutputStream
, and Files
classes, providing clear code examples and explanations along the way. Let’s dive into the world of file manipulation in Java!
Using the File Class
One of the simplest ways to create a file in Java is by using the File
class. This class offers methods to create a new file and check if it already exists. Below is an example demonstrating how to use the File
class to create a file only if it does not exist.
import java.io.File;
import java.io.IOException;
public class CreateFileExample {
public static void main(String[] args) {
File file = new File("example.txt");
try {
if (file.createNewFile()) {
System.out.println("File created: " + file.getName());
} else {
System.out.println("File already exists.");
}
} catch (IOException e) {
System.out.println("An error occurred.");
e.printStackTrace();
}
}
}
Output:
File created: example.txt
In this code snippet, we first import the necessary classes. We then create a File
object with the desired filename. The createNewFile()
method attempts to create the file. If the file already exists, it returns false
, and we print a message indicating that. If the file is created successfully, we print a confirmation message. The IOException
is caught to handle any errors that might occur during this process, ensuring that your program remains robust and informative.
Using FileOutputStream
Another approach to create a file if it does not exist is by using FileOutputStream
. This method is particularly useful when you want to write data to the file immediately upon creation. Below is a code example that illustrates this method.
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
public class CreateFileUsingOutputStream {
public static void main(String[] args) {
File file = new File("output.txt");
try (FileOutputStream fos = new FileOutputStream(file, false)) {
if (file.createNewFile()) {
String content = "This is a new file created using FileOutputStream.";
fos.write(content.getBytes());
System.out.println("File created and content written: " + file.getName());
} else {
System.out.println("File already exists.");
}
} catch (IOException e) {
System.out.println("An error occurred.");
e.printStackTrace();
}
}
}
Output:
File created and content written: output.txt
In this example, we create a FileOutputStream
to write data to the file. The false
parameter in the constructor indicates that we want to overwrite the file if it already exists. We check for the file’s existence and create it if necessary. Upon successful creation, we write a string to the file. The try-with-resources statement ensures that the FileOutputStream
is closed automatically, which is a good practice to prevent resource leaks.
Using the Files Class
Java 7 introduced the Files
class, which provides a more modern way to handle file operations. This method is recommended for its simplicity and efficiency. Here’s how you can create a file using the Files
class.
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class CreateFileWithFilesClass {
public static void main(String[] args) {
Path path = Paths.get("newfile.txt");
try {
if (!Files.exists(path)) {
Files.createFile(path);
System.out.println("File created: " + path.getFileName());
} else {
System.out.println("File already exists.");
}
} catch (IOException e) {
System.out.println("An error occurred.");
e.printStackTrace();
}
}
}
Output:
File created: newfile.txt
In this code, we use the Paths.get()
method to create a Path
object representing the file. The Files.exists()
method checks if the file already exists. If it doesn’t, we call Files.createFile()
to create it. This method is clean and efficient, making it a preferred choice for modern Java applications. The IOException
is again caught to handle any errors that might arise during file creation.
Conclusion
Creating files in Java when they do not exist is a fundamental skill that every developer should master. In this tutorial, we explored three different methods: using the File
class, FileOutputStream
, and the Files
class. Each method has its own advantages, depending on your specific needs, such as writing data immediately or simply creating a file. By understanding these techniques, you can enhance your file management skills in Java, leading to more robust applications. Happy coding!
FAQ
- how do I check if a file exists in Java?
You can use theFile.exists()
method orFiles.exists()
method to check if a file exists.
-
can I create a file in a specific directory?
Yes, you can specify the directory in the file path when creating aFile
orPath
object. -
what happens if I try to create a file that already exists?
The methods we discussed will not overwrite the existing file and will return a message indicating that the file already exists. -
is it necessary to handle exceptions when creating files?
Yes, handlingIOException
is important to manage potential errors during file operations. -
can I create directories as well as files in Java?
Yes, you can create directories using themkdir()
ormkdirs()
methods in theFile
class orFiles.createDirectory()
method.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook