How to Copy a File in Java
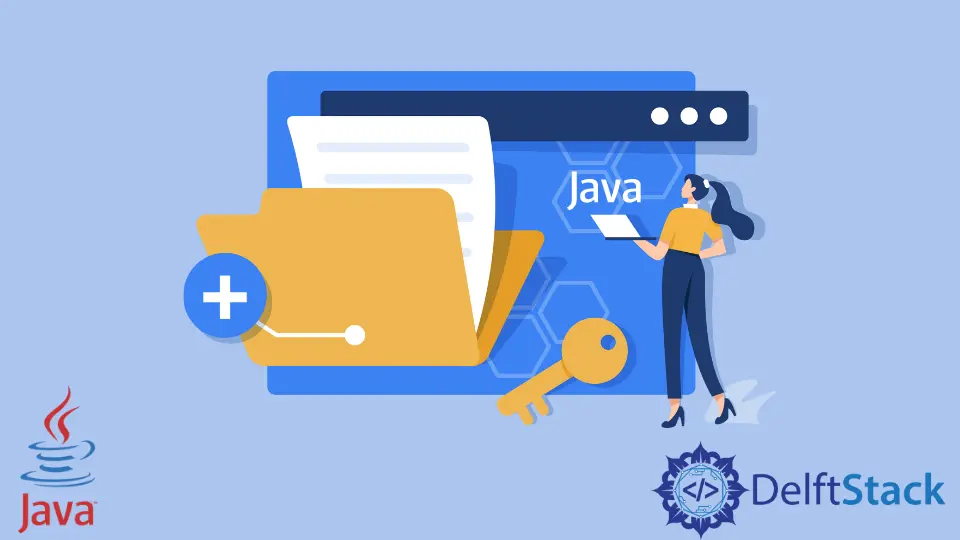
In this article, we’ll introduce the method you can copy a file from one location to another location. In the Java language, there are libraries that allow us to move one file to another directory. Let’s take a deeper look.
Copying files through java code involves storing a source and destination path in two different strings. Later, we capture our desired file through the source path and create its copy for the destination location. You need to add this library for the following code to work.
import static java.nio.file.StandardCopyOption.*;
Here are some exceptions that you need to handle.
Exception Handling When Copying Files in Java
Following are the three exception handling keywords that may come in handy for you.
- If you come across a situation where a file is already in the destination location (same name), you should use
REPLACE_EXISTING
. This will replace the already existing file. COPY_ATTRIBUTES
, this preserved keyword will copy the attributes linked with the source file.- If you don’t want the symbolic links to follow, meaning that you don’t want to copy the target link, you can use
NOFOLLOW_LINKS
.
Example Code of Copying a File in Java
import static java.nio.file.StandardCopyOption.*;
import java.io.*;
import java.nio.file.Files;
public class Main {
public static void main(String[] args) {
String sourcePath = "E:\\source location\\delftstack.txt"; // source file path
String destinationPath = "E:\\destination location\\"; // destination file path
File sourceFile = new File(sourcePath); // Creating A Source File
File destinationFile = new File(
destinationPath + sourceFile.getName()); // Creating A Destination File. Name stays the same
// this way, referring to getName()
try {
Files.copy(sourceFile.toPath(), destinationFile.toPath(), REPLACE_EXISTING);
// Static Methods To Copy Copy source path to destination path
} catch (Exception e) {
System.out.println(e); // printing in case of error.
}
}
}
In the above code example, as you can see, the libraries are added. We copied the source path, saved it inside a string sourcepath
, and did the same with the destination location.
Later, we created a source file (file object) and passed it to the string of the source path. Now, we know that while we usually copy and paste files, the name stays the same. To do that, we use getName()
with the source file.
By using the Files.copy(source, target, REPLACE_EXISTING);
command and passing the values, we have copied a text file in java in the above code example.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn