How to Copy a 2D Array in Java
- Copy a 2D Array in Java
- Use Loop Iteration to Copy a 2D Array in Java
-
Use the
clone()
Method to Copy a 2D Array in Java -
Use the
System.arraycopy()
Method to Copy a 2D Array in Java -
Use the
Arrays.copyOf()
Method to Copy a 2D Array in Java - Use Stream API to Copy a 2D Array in Java
- Conclusion
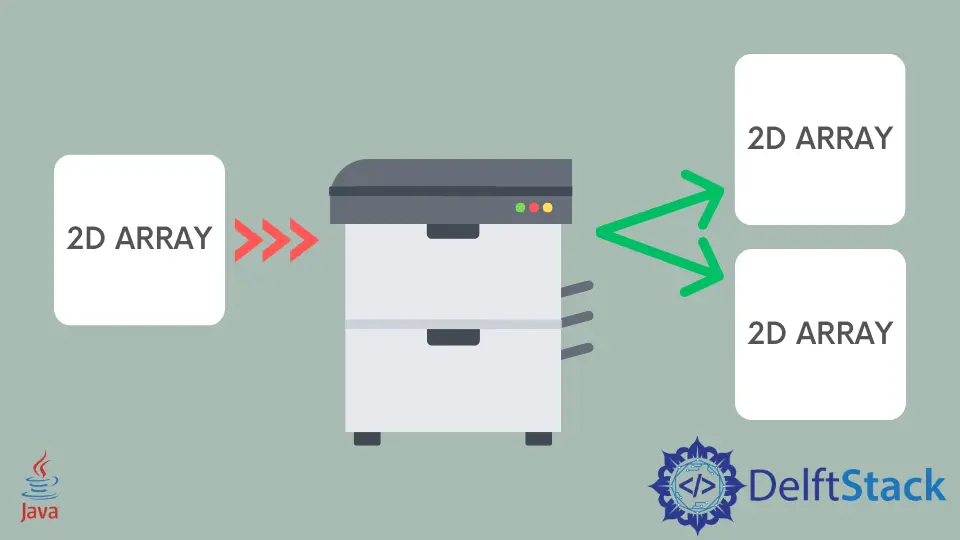
Copying 2D arrays in Java is essential for various programming tasks, from manipulating data to ensuring the integrity of original arrays. This comprehensive guide delves into multiple methods for copying 2D arrays in Java, providing detailed examples and explanations for each approach.
Copy a 2D Array in Java
Whenever we attempt to copy elements of the 2D array to another array, we often assign an original array to the destination array. Why this approach is logically wrong, we’ll discuss it first.
Even though the below-mentioned solution is logically wrong, we want to let you know why this solution doesn’t work.
// Java Program to copy 2-dimensional array
// create 2D array
int[][] arr1 = {{2, 4, 6}, {8, 10, 12}};
// creating the same array with the same size
int[][] arr2 = new int[arr1.length];
// this code does not copy elements of arr1[ ] to arr2[ ] because arr2[ ] sill refer to the same
// location
arr2 = arr1;
When we write arr2=arr1
, we are assigning arr2[ ]
a reference to the arr1[ ]
. Therefore, changing one array will be reflected in both the copied and the original array because both arrays are pointing to the same location.
To demonstrate this fact, have a look at the code depicted below.
// A Java program to demonstrate assigning an array reference doesn't make any sense
public class copy2DArray {
public static void main(String[] args) {
// Create an array arr1[]
int[][] arr1 = {{2, 4, 5}, {6, 8, 10}};
// Create an array arr2[] with the same size
int[][] arr2 = new int[arr1.length][];
// Doesn't copy elements of arr1 to arr2, but only arr2 starts referring arr1
arr2 = arr1;
// Any changing in the arr2 also reflects in the arr1 because
// Both are referring to the same location
arr2[0][0] = 88;
System.out.println("Elements of arr1[] are:");
for (int i = 0; i < arr1.length; i++) {
for (int j = 0; j < arr1.length; j++) {
System.out.println(arr1[i][j] + " ");
}
}
System.out.println("Elements of arr2[] are:");
for (int i = 0; i < arr2.length; i++) {
for (int j = 0; j < arr2.length; j++) {
System.out.println(arr2[i][j] + " ");
}
}
}
}
Output:
Elements of arr1[] are:
88
4
6
8
Elements of arr2[] are:
88
4
6
8
This behavior highlights the importance of understanding references in Java when working with arrays. Direct assignment (arr2 = arr1;
) only makes the reference point to the same memory location, and it doesn’t create a separate copy of the array’s content.
To create a true copy, a proper copying method like loop iteration, System.arraycopy()
, or Arrays.copyOf()
should be used.
In the above example, we have discussed the common mistakes developers often make while copying elements of the 2D array. Now, we’ll discuss the correct methods to accomplish the same task.
In Java, we can copy array elements using the following methods:
- Iterate all elements of an array and copy each element
- By using the
clone()
method - By using
System.arraycopy()
method - By using the
Arrays.copyOf()
method - By using Stream API
Use Loop Iteration to Copy a 2D Array in Java
Java, being an object-oriented programming language, allows developers to manipulate arrays efficiently. Copying a 2D array is a common task in Java programming, often achieved through loop iteration.
This method involves traversing through each element of the original array and duplicating its content into a new array.
Using this method, you’ll experience that any destination or original array modification will not affect an original array. Thus, the original array remains intact.
Let’s delve into an example illustrating the usage of loop iteration to copy a 2D array in Java:
// A Java program to demonstrate assigning an array reference doesn't make any sense
import java.util.Arrays;
public class copy2DArray {
public static void main(String[] args) {
// Create an array arr1[ ]
int[][] arr1 = {{2, 4, 5}, {6, 8, 10}};
// Create an array arr2[ ] with the same size
int[][] arr2 = new int[arr1.length][];
// Copying elements of arr1[ ] to arr2[ ]
for (int i = 0; i < arr1.length; i++) {
// allocation space to each row of arr2[]
arr2[i] = new int[arr1[i].length];
for (int j = 0; j < arr1[i].length; j++) {
arr2[i][j] = arr1[i][j];
}
}
// Any change in the elements of arr2[ ] will not be reflected in an original array
arr2[0][0] = 90;
System.out.println("Elements of arr1[] are:");
for (int i = 0; i < arr1.length; i++) {
for (int j = 0; j < arr1[0].length; j++) {
System.out.println(arr1[i][j] + " ");
}
}
System.out.println("Elements of arr2[] are:");
for (int i = 0; i < arr2.length; i++) {
for (int j = 0; j < arr2[0].length; j++) {
System.out.println(arr2[i][j] + " ");
}
}
}
}
Output:
Elements of arr1[] are:
2
4
5
6
8
10
Elements of arr2[] are:
90
4
5
6
8
10
This code showcases how to create a separate copy of a 2D array without retaining a shared reference between the original and copied arrays. By using nested loops and individual element assignments, it ensures that changes made to the copied array do not affect the original.
The code creates an initial 2D array, arr1
, and generates a new array, arr2
, with the same size as arr1
, allocating space for each row individually. It iterates through arr1
and copies its elements to arr2
using nested loops, ensuring distinct copies.
It demonstrates that modifying arr2
doesn’t impact arr1
, emphasizing the absence of shared references between the arrays. Finally, it outputs the elements of both arrays to validate the independence of modifications between them.
Use the clone()
Method to Copy a 2D Array in Java
We used the loop iteration technique to copy 2D array elements in the previous method. We can do the same task with the clone()
method.
Java provides the clone()
method for creating a shallow copy of objects, including arrays. This method is applicable to arrays, enabling developers to duplicate the contents of an array without retaining the reference to the original array.
When used correctly, the clone()
method can create an independent copy of the array elements.
The syntax for the clone()
method in Java is as follows:
protected Object clone() throws CloneNotSupportedException
The clone()
method in Java doesn’t take any parameters.
Return Type:
- The
clone()
method returns a cloned or copied object. The return type isObject
. - To use the cloned object as its intended type, explicit casting is often necessary.
Exceptions:
- The
clone()
method throws aCloneNotSupportedException
if the object’s class does not implement theCloneable
interface.
Let’s delve into an example illustrating the usage of the clone()
method to copy a 2D array in Java:
// A Java program to demonstrate assigning an array reference doesn't make any sense
import java.util.Arrays;
public class copy2DArray {
public static void main(String[] args) {
// Create an array arr1[]
int[][] arr1 = {{2, 4, 5}, {6, 8, 10}};
// Create an array arr2[] with the same size
int[][] arr2 = new int[arr1.length][];
// Copying elements of arr1[ ] to arr2[ ] using the clone() method
for (int i = 0; i < arr1.length; i++) arr2[i] = arr1[i].clone();
// Any change in the elements of arr2[] will not be reflected in an original array
arr2[0][0] = 90;
System.out.println("Elements of arr1[] are:");
for (int i = 0; i < arr1.length; i++) {
for (int j = 0; j < arr1[0].length; j++) {
System.out.println(arr1[i][j] + " ");
}
}
System.out.println("Elements of arr2[] are:");
for (int i = 0; i < arr2.length; i++) {
for (int j = 0; j < arr2[0].length; j++) {
System.out.println(arr2[i][j] + " ");
}
}
}
}
Output:
Elements of arr1[] are:
2
4
5
6
8
10
Elements of arr2[] are:
90
4
5
6
8
10
The output displays both arr1
and arr2
. Modifying an element in arr2
won’t affect arr1
.
The use of arr2[i] = arr1[i].clone()
ensures that a separate copy of each row from arr1
is made into arr2
. Therefore, modifications in one array won’t impact the other.
This code highlights the importance of using proper copying techniques like the clone()
method to ensure the independence of arrays when modifications are made, preventing unwanted side effects between original and copied arrays.
Use the System.arraycopy()
Method to Copy a 2D Array in Java
Likewise, we can copy 2D arrays using the arraycopy()
method. We can copy elements of any 2D array without iterating all the array elements with this method.
In Java, the System.arraycopy()
method offers a way to efficiently copy elements from one array to another. While it’s commonly used for copying elements within a 1D array, it can also be leveraged to copy elements between 2D arrays.
This method provides control over the copying process and allows developers to create independent copies of arrays.
Syntax:
System.arraycopy(Object src, int srcPos, Object dest, int destPos, int length);
To use this method, we need to provide the following parameters:
src
: The source array from which elements will be copied.srcPos
: The starting position in the source array from where elements will be copied.dest
: The destination array where elements will be copied.destPos
: The starting position in the destination array where elements will be copied.length
: The number of elements to be copied from the source to the destination.
Exception:
NullPointerException
: if either source or destination array is not defined or doesn’t exist.ArrayStoreException
: If a developer attempts to copy an integer array type into a string array, this situation automatically triggers this exception.
Notes:
- Both
src
anddest
must be arrays. - The types of
src
anddest
must match (both should be arrays of the same type). - The number of elements to copy (
length
) should not exceed the available elements in either the source or destination array.
Let’s explore an example illustrating the usage of System.arraycopy()
to copy a 2D array in Java:
package sampleProject;
import java.util.Arrays;
public class Codesample {
public static void main(String[] args) {
// Create an array arr1[]
int[][] arr1 = {{2, 4, 5}, {6, 8, 10}};
// Create an array arr2[] with the same size
int[][] arr2 = new int[arr1.length][];
// Copying elements of arr1[] to arr2[] using the clone() method
for (int i = 0; i < arr1.length; i++) {
arr2[i] = new int[arr1[i].length];
System.arraycopy(arr1[i], 0, arr2[i], 0, arr1[i].length);
}
// Any change in the elements of arr2[] will not be reflected in an original array
arr2[0][0] = 90;
System.out.println("Elements of arr1[] are:");
for (int i = 0; i < arr1.length; i++) {
for (int j = 0; j < arr1[0].length; j++) {
System.out.println(arr1[i][j] + " ");
}
}
System.out.println("Elements of arr2[] are:");
for (int i = 0; i < arr2.length; i++) {
for (int j = 0; j < arr2[0].length; j++) {
System.out.println(arr2[i][j] + " ");
}
}
}
}
Output:
Elements of arr1[] are:
2
4
5
6
8
10
Elements of arr2[] are:
90
4
5
6
8
10
The output displays both arr1
and arr2
. Modifying an element in arr2
won’t affect arr1
.
Each row of arr1
is copied individually to arr2
using System.arraycopy()
, ensuring that changes in one array do not affect the other.
This code demonstrates the importance of properly copying array elements, ensuring independence between original and copied arrays, and avoiding unwanted side effects when modifications are made to copied arrays.
Use the Arrays.copyOf()
Method to Copy a 2D Array in Java
In Java, the Arrays.copyOf()
method provides a straightforward way to copy an array, including 2D arrays. This method creates a new array with a specified length and copies elements from the original array into the new array. It allows for both shallow and deep copies, depending on the array type.
Here’s the syntax for the Arrays.copyOf()
method:
public static <T> T[] copyOf(T[] original, int newLength)
Parameters:
original
: The original array to be copied.newLength
: The desired length of the new array.
Return Type:
- The method returns a new array containing the copied elements.
Let’s explore an example illustrating the usage of Arrays.copyOf()
to copy a 2D array in Java:
import java.util.Arrays;
public class Copy2DArray {
public static void main(String[] args) {
// Create the original 2D array
int[][] originalArray = {{2, 4, 5}, {6, 8, 10}};
// Copy the original array to a new array using Arrays.copyOf()
int[][] copiedArray = Arrays.copyOf(originalArray, originalArray.length);
// Modify an element in the copied array
copiedArray[0][0] = 90;
// Display elements of the original and copied arrays
System.out.println("Original Array:");
print2DArray(originalArray);
System.out.println("Copied Array:");
print2DArray(copiedArray);
}
// Helper method to print a 2D array
public static void print2DArray(int[][] array) {
for (int[] row : array) {
for (int value : row) {
System.out.print(value + " ");
}
System.out.println();
}
}
}
Output:
Original Array:
90 4 5
6 8 10
Copied Array:
90 4 5
6 8 10
Explanation of the Code:
originalArray
Initialization: Creates the initial 2D array with values{ { 2, 4, 5 }, { 6, 8, 10 } }
.Arrays.copyOf()
Usage: Copies the entireoriginalArray
tocopiedArray
usingArrays.copyOf()
.- Modification of Copied Array: Changes an element in
copiedArray
to illustrate the independence of modifications between the arrays. - Displaying Arrays: Outputs both
originalArray
andcopiedArray
to demonstrate their respective elements.
The output will display both originalArray
and copiedArray
. Modifying an element in copiedArray
won’t affect originalArray
because Arrays.copyOf()
creates a shallow copy of the outer array. Therefore, modifications in one array won’t impact the other at the outer level.
Use Stream API to Copy a 2D Array in Java
The Stream API, introduced in Java 8, provides a powerful way to manipulate collections and arrays. Leveraging streams to copy a 2D array allows for concise and functional programming approaches.
- Streams can be used with arrays via
Arrays.stream()
for 1D arrays. However, for 2D arrays, a stream of arrays or a nested stream approach is needed. - Stream operations provide functional methods like
map()
,flatMap()
, andtoArray()
to facilitate transformations on arrays.
The approach involves using streams to traverse and copy elements of the original 2D array to a new array.
Let’s dive into an example demonstrating the usage of the Stream API to copy a 2D array in Java:
import java.util.Arrays;
public class Copy2DArrayWithStreams {
public static void main(String[] args) {
// Create the original 2D array
int[][] originalArray = {{2, 4, 5}, {6, 8, 10}};
// Copy the original array to a new array using Stream API
int[][] copiedArray = Arrays.stream(originalArray).map(int[] ::clone).toArray(int[][] ::new);
// Modify an element in the copied array
copiedArray[0][0] = 90;
// Display elements of the original and copied arrays
System.out.println("Original Array:");
print2DArray(originalArray);
System.out.println("Copied Array:");
print2DArray(copiedArray);
}
// Helper method to print a 2D array
public static void print2DArray(int[][] array) {
for (int[] row : array) {
for (int value : row) {
System.out.print(value + " ");
}
System.out.println();
}
}
}
Output:
Original Array:
2 4 5
6 8 10
Copied Array:
90 4 5
6 8 10
Explanation of the Code:
originalArray
Initialization: Creates the initial 2D array with values{ { 2, 4, 5 }, { 6, 8, 10 } }
.- Stream API Usage: Uses
Arrays.stream()
to create a stream of arrays,map()
to clone each inner array, andtoArray()
to collect the cloned arrays into a new 2D array (copiedArray
). - Modification of Copied Array: Changes an element in
copiedArray
to illustrate the independence of modifications between the arrays. - Displaying Arrays: Outputs both
originalArray
andcopiedArray
to demonstrate their respective elements.
The output displays both originalArray
and copiedArray
. Modifying an element in copiedArray
won’t affect originalArray
because the Stream API creates a new array with cloned inner arrays. Thus, modifications in one array won’t impact the other.
Conclusion
The article delves into various methods of copying 2D arrays in Java, emphasizing the importance of choosing the right approach to avoid shared references and ensure data integrity. It explores five distinct techniques:
- Loop Iteration: Manually copies elements to a new array, preserving the independence of the original and copied arrays.
- Using
clone()
: Creates a shallow copy, retaining shared references between nested arrays. System.arraycopy()
: Efficiently copies elements between arrays, ensuring independence between the original and copied arrays.Arrays.copyOf()
: Creates a new array with copied elements, allowing for shallow copies without affecting the original array.- Stream API: Provides a concise and functional approach, allowing transformations and cloning of elements while maintaining independence between arrays.
Understanding these methods enables developers to choose the most suitable approach for their specific needs, ensuring efficient and accurate manipulation of 2D arrays in Java programs.