Copier des tableaux 2D en Java
- Utilisation de l’itération de boucle pour copier un tableau 2D en Java
-
Utilisation de la méthode
clone()
pour copier un tableau 2D en Java -
Utilisation de la méthode
arraycopy()
pour copier un tableau 2D en Java
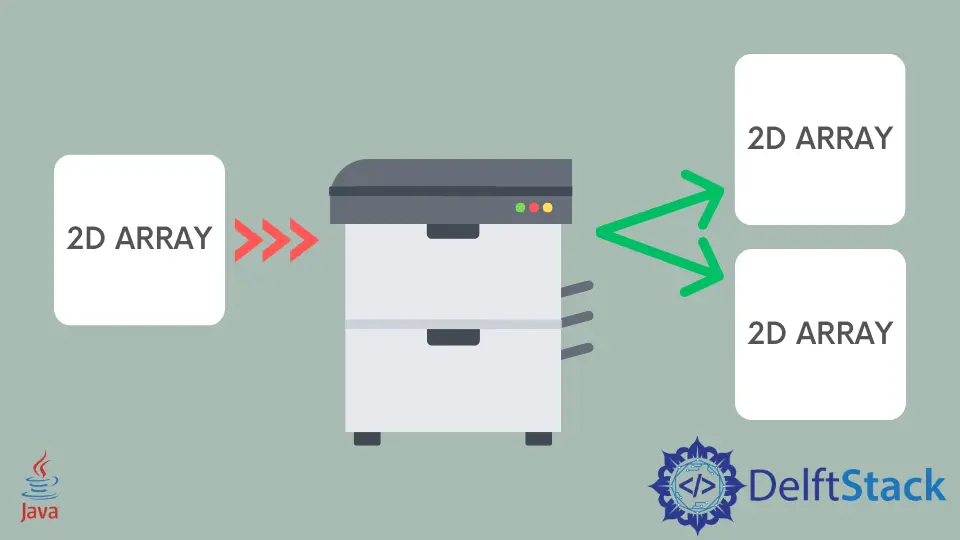
Chaque fois que nous essayons de copier des éléments du tableau 2D dans un autre tableau, nous affectons souvent un tableau d’origine au tableau de destination. Pourquoi cette approche est logiquement fausse, nous en discuterons d’abord.
Même si la solution mentionnée ci-dessous est logiquement fausse, nous voulons vous faire savoir pourquoi cette solution ne fonctionne pas.
// Java Program to copy 2-dimensional array
// create 2D array
int[][] arr1 = {{2, 4, 6}, {8, 10, 12}};
// creating the same array with the same size
int[][] arr2 = new int[arr1.length];
// this code does not copy elements of arr1[ ] to arr2[ ] because arr2[ ] sill refer to the same
// location
arr2 = arr1;
Lorsque nous écrivons arr2=arr1
, nous affectons à arr2[ ]
une référence au arr1[ ]
. Par conséquent, la modification d’un tableau sera reflétée à la fois dans le tableau copié et dans le tableau d’origine, car les deux tableaux pointent vers le même emplacement.
Pour démontrer ce fait, jetez un œil au code décrit ci-dessous.
// A Java program to demonstrate assigning an array reference doesn't make any sense
public class copy2DArray {
public static void main(String[] args) {
// Create an array arr1[]
int[][] arr1 = {{2, 4, 5}, {6, 8, 10}};
// Create an array arr2[] with the same size
int[][] arr2 = new int[arr1.length][];
// Doesn't copy elements of arr1 to arr2, but only arr2 starts refering arr1
arr2 = arr1;
// Any changing in the arr2 also reflects in the arr1 because
// both are referin to the same location
arr2[0][0] = 88;
System.out.println("Elements of arr1[] are:");
for (int i = 0; i < arr1.length; i++) {
for (int j = 0; j < arr1.length; j++) {
System.out.println(arr1[i][j] + " ");
}
}
System.out.println("\n\nElements of arr2[] are:");
for (int i = 0; i < arr2.length; i++) {
for (int j = 0; j < arr2.length; j++) {
System.out.println(arr2[i][j] + " ");
}
}
}
}
Production :
Elements of arr1[] are:
88
4
6
8
Elements of arr2[] are:
88
4
6
8
Dans l’exemple ci-dessus, nous avons discuté des erreurs courantes que les développeurs commettent souvent lors de la copie d’éléments du tableau 2D. Nous allons maintenant discuter de la méthode correcte pour accomplir la même tâche.
En Java, nous pouvons copier des éléments de tableau en utilisant les méthodes suivantes :
- Itérez tous les éléments d’un tableau et copiez chaque élément.
- En utilisant la méthode
clone()
. - En utilisant la méthode
arraycopy()
.
Utilisation de l’itération de boucle pour copier un tableau 2D en Java
Technique d’itération de boucle pour copier un tableau 2D. En utilisant cette méthode, vous découvrirez que toute modification de destination ou de tableau d’origine n’affectera pas un tableau d’origine. Ainsi, le tableau d’origine reste intact.
// A Java program to demonstrate assigning an array reference doesn't make any sense
import java.util.Arrays;
public class copy2DArray {
public static void main(String[] args) {
// Create an array arr1[ ]
int[][] arr1 = {{2, 4, 5}, {6, 8, 10}};
// Create an array arr2[ ] with the same size
int[][] arr2 = new int[arr1.length][];
// Copying elements of arr1[ ] to arr2[ ]
for (int i = 0; i < arr1.length; i++) {
// allocation space to each row of arr2[]
arr2[i] = new int[arr1[i].length];
for (int j = 0; j < arr1[i].length; j++) {
arr2[i][j] = arr1[i][j];
}
}
// Any change in the elements of arr2[ ] will not be reflected in an original array
arr2[0][0] = 90;
System.out.println("Elements of arr1[] are:");
for (int i = 0; i < arr1.length; i++) {
for (int j = 0; j < arr1[0].length; j++) {
System.out.println(arr1[i][j] + " ");
}
}
System.out.println("\n\nElements of arr2[] are:");
for (int i = 0; i < arr2.length; i++) {
for (int j = 0; j < arr2[0].length; j++) {
System.out.println(arr2[i][j] + " ");
}
}
}
}
Production :
Elements of arr1[] are:
2
4
5
6
8
10
Elements of arr2[] are:
90
4
5
6
8
10
Utilisation de la méthode clone()
pour copier un tableau 2D en Java
Nous avons utilisé la technique d’itération de boucle pour copier des éléments de tableau 2D dans la méthode précédente. Nous pouvons faire la même tâche avec la méthode clone()
.
// A Java program to demonstrate assigning an array reference doesn't make any sense
import java.util.Arrays;
public class copy2DArray {
public static void main(String[] args) {
// Create an array arr1[]
int[][] arr1 = {{2, 4, 5}, {6, 8, 10}};
// Create an array arr2[] with the same size
int[][] arr2 = new int[arr1.length][];
// Copying elements of arr1[ ] to arr2[ ] using the clone() method
for (int i = 0; i < arr1.length; i++) arr2[i] = arr1[i].clone();
// Any change in the elements of arr2[] will not be reflected in an original array
arr2[0][0] = 90;
System.out.println("Elements of arr1[] are:");
for (int i = 0; i < arr1.length; i++) {
for (int j = 0; j < arr1[0].length; j++) {
System.out.println(arr1[i][j] + " ");
}
}
System.out.println("\n\nElements of arr2[] are:");
for (int i = 0; i < arr2.length; i++) {
for (int j = 0; j < arr2[0].length; j++) {
System.out.println(arr2[i][j] + " ");
}
}
}
}
Production :
Elements of arr1[] are:
2
4
5
6
8
10
Elements of arr2[] are:
90
4
5
6
8
10
Utilisation de la méthode arraycopy()
pour copier un tableau 2D en Java
De même, nous pouvons copier des tableaux 2D en utilisant la méthode arraycopy()
. Nous pouvons copier des éléments de n’importe quel tableau 2D sans itérer tous les éléments du tableau avec cette méthode. Pour utiliser cette méthode, nous devons fournir les paramètres suivants :
src
: tableau source que vous devez copiersrcPos
: emplacement de départ d’un tableau d’origine.dest
: tableau destination.destPos
: indice de départ du tableau destination.length
: nombre total d’éléments du tableau 2D que l’on veut copier
Exception:
NullPointerException
: si le tableau source ou destination n’est pas défini ou n’existe pas.ArrayStoreException
: Si un développeur tente de copier un type tableau d’entiers dans un tableau de chaînes, cette situation déclenche automatiquement cette exception.
Exemple:
package sampleProject;
import java.util.Arrays;
public class Codesample {
public static void main(String[] args) {
// Create an array arr1[]
int[][] arr1 = {{2, 4, 5}, {6, 8, 10}};
// Create an array arr2[] with the same size
int[][] arr2 = new int[arr1.length][];
// Copying elements of arr1[] to arr2[] using the clone() method
for (int i = 0; i < arr1.length; i++) {
arr2[i] = new int[arr1[i].length];
System.arraycopy(arr1[i], 0, arr2[i], 0, arr1[i].length);
}
// Any change in the elements of arr2[] will not be reflected in an original array
arr2[0][0] = 90;
System.out.println("Elements of arr1[] are:");
for (int i = 0; i < arr1.length; i++) {
for (int j = 0; j < arr1[0].length; j++) {
System.out.println(arr1[i][j] + " ");
}
}
System.out.println("\n\nElements of arr2[] are:");
for (int i = 0; i < arr2.length; i++) {
for (int j = 0; j < arr2[0].length; j++) {
System.out.println(arr2[i][j] + " ");
}
}
}
}
Production :
Elements of arr1[] are:
2
4
5
6
8
10
Elements of arr2[] are:
90
4
5
6
8
10