How to Check if an Object Is Null in Java
-
Check for Null Objects in Java Using the Equality (
==
) Operator -
Check for Null Objects in Java Using
java.util.Objects
-
Check for Null Objects in Java Using the
equals
Method -
Check for Null Objects in Java Using the
Optional
Class - Check for Null Objects in Java Using the Ternary Operator
- Conclusion
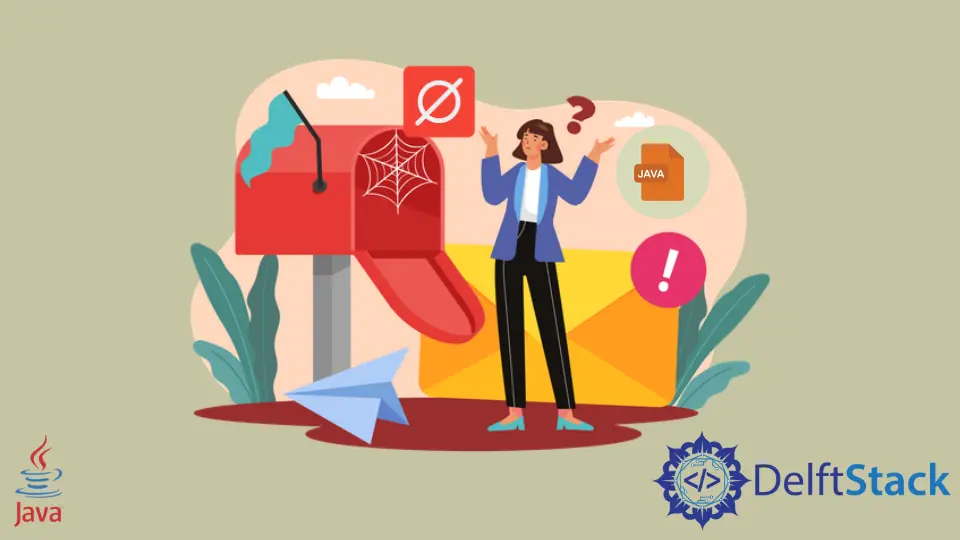
In Java programming, dealing with null values is a common task, and ensuring robust null-checking mechanisms is essential for writing reliable and error-free code. Whether you are handling user inputs, external data, or complex data structures, verifying if an object is null
before proceeding with operations is a fundamental practice.
Java provides several methods and techniques for performing null checks, each with its unique characteristics and use cases. In this article, we will explore various approaches, from classic methods like the equality operator and the equals
method to more modern solutions such as the java.util.Objects
class and the Optional
class introduced in Java 8.
Check for Null Objects in Java Using the Equality (==
) Operator
One straightforward approach to check whether an object is null
is using the equality (==
) operator. This operator compares the memory addresses of two objects, making it a suitable choice for testing nullity.
When applied to object references, the equality operator checks whether they point to the same memory location, indicating that the objects are the same. When used in the context of checking for null
, it evaluates to true
if the object reference is null
and false
otherwise.
Code Example: Checking for Null Using the Equality Operator
Let’s consider a practical example with two classes, User1
and User2
.
The User1
class has a single instance variable, name
, along with Getter and Setter methods. The User2
class contains a method, getUser1Object
, which returns an instance of User1
.
public class CheckNullWithEqualityOperator {
public static void main(String[] args) {
User2 user = new User2();
User1 getUserObject = user.getUser1Object();
if (getUserObject == null) {
System.out.println("Object is Null");
} else {
System.out.println("Not Null");
getUserObject.setName("John Doe");
System.out.println("Name: " + getUserObject.getName());
}
}
}
class User2 {
User1 user;
public User1 getUser1Object() {
return user;
}
}
class User1 {
String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
In this Java program, the first line within the main
method declares an object of class User2
named user
and initializes it.
Following that, we call the getUser1Object()
method on the user
object, which is a method of class User2
. This method returns an instance of User1
and is assigned to the variable getUserObject
.
Next, we encounter an if-else
statement that checks if the object referenced by getUserObject
is null
using the equality operator (==
). If the condition evaluates to true
, the program enters the if
block, and the message Object is Null
is printed to the console.
Otherwise, the program enters the else
block, where Not Null
is printed. Additionally, within the else
block, we set the name of the User1
object using the setName
method and print the updated name to the console.
Now, let’s explore the supporting classes. Class User2
contains an instance variable user
of type User1
.
It also includes a method named getUser1Object
that returns the user
object. The purpose of this method is to simulate a scenario where an object of User1
is retrieved.
class User2 {
User1 user;
public User1 getUser1Object() {
return user;
}
}
Class User1
is relatively simple, with a single instance variable name
representing a user’s name. It includes Getter and Setter methods (getName
and setName
, respectively) to retrieve and update the name
attribute.
class User1 {
String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
Code Output:
In this output, the message Object is Null
indicates that the object returned by getUser1Object()
is indeed null
. This approach is simple and widely used for checking null objects in Java.
Check for Null Objects in Java Using java.util.Objects
Another approach to checking if an object is null
involves using the java.util.Objects
class, specifically the isNull()
method. This method provides a convenient and robust way to handle null checks, offering improved readability and avoiding potential pitfalls associated with direct equality comparisons.
The java.util.Objects
class, introduced in Java 7, includes utility methods for object-related operations. Among these methods is isNull()
, which takes an object reference as an argument and returns true
if the reference is null
, and false
otherwise.
This method offers a concise and null-safe way to perform null checks.
Code Example: Checking for Null Using java.util.Objects
Let’s delve into a practical example to demonstrate the use of java.util.Objects.isNull()
. In this scenario, we have two classes, User1
and User2
, similar to the previous example.
import java.util.Objects;
public class CheckNullWithObjects {
public static void main(String[] args) {
User2 user = new User2();
User1 getUserObject = user.getUser1Object();
if (Objects.isNull(getUserObject)) {
System.out.println("Object is Null");
} else {
System.out.println("Not Null");
getUserObject.setName("John Doe");
System.out.println("Name: " + getUserObject.getName());
}
}
}
class User2 {
User1 user;
public User1 getUser1Object() {
return user;
}
}
class User1 {
String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
In this example, we import the java.util.Objects
class and utilize the isNull()
method within the main
method. The rest of the code remains similar to the previous example.
An object of class User2
is created, and the getUser1Object()
method is called to obtain an instance of User1
. The isNull()
method is then employed within an if-else
statement to check if the object is null
.
If the object is null
, the program prints Object is Null
. Conversely, if the object is not null
, it proceeds to the else
block, where Not Null
is printed.
Additionally, within the else
block, the name of the User1
object is set using the setName
method, and the updated name is printed to the console.
Code Output:
In this output, the message Object is Null
indicates that the java.util.Objects.isNull()
method correctly identified the null object. This method provides a concise and reliable way to perform null checks in Java, improving code readability and reducing the chances of errors associated with direct equality comparisons.
Check for Null Objects in Java Using the equals
Method
An alternative method for checking if an object is null
involves using the equals
method. While primarily designed for comparing objects for equality, the equals
method can also be employed to check if an object is null
.
The equals
method is part of the Object
class in Java and is used for comparing the contents of objects for equality. When applied to a non-null object, it returns true
if the compared objects are equal and false
otherwise.
However, when applied to a null object, it results in a NullPointerException
. To perform a null check using equals
, it is essential to ensure that the object reference is not null before calling the method.
Code Example: Checking for Null Using the equals
Method
Let’s explore a practical example that demonstrates how to use the equals
method for null checks. In this scenario, we have two classes, User1
and User2
, similar to the previous examples.
public class CheckNullWithEqualsMethod {
public static void main(String[] args) {
User2 user = new User2();
User1 getUserObject = user.getUser1Object();
if (getUserObject == null || getUserObject.equals(null)) {
System.out.println("Object is Null");
} else {
System.out.println("Not Null");
getUserObject.setName("John Doe");
System.out.println("Name: " + getUserObject.getName());
}
}
}
class User2 {
User1 user;
public User1 getUser1Object() {
return user;
}
}
class User1 {
String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
In this example, we perform a null check using the equals
method within the main
method. The code checks if the object reference getUserObject
is null or if calling equals(null)
on it results in true
.
If either condition is satisfied, the program prints Object is Null
. Otherwise, it proceeds to the else
block, printing Not Null
.
Similar to previous examples, within the else
block, the name of the User1
object is set using the setName
method, and the updated name is printed to the console.
Code Output:
In this output, the message Object is Null
indicates that the null check using the equals
method correctly identified the null object. However, it’s crucial to note that this approach introduces a risk of NullPointerException
and may not be as reliable as other methods specifically designed for null checks.
Careful consideration should be given to the potential pitfalls of using the equals
method in this context.
Check for Null Objects in Java Using the Optional
Class
In Java, the Optional
class, introduced in Java 8, provides an elegant and null-safe way to handle potentially null values. It offers methods to perform various operations on an object while avoiding the risk of NullPointerException
.
The Optional
class is part of the java.util
package and is designed to provide a more expressive and safer alternative to dealing with potentially null values. It encourages a functional programming style by allowing chained operations on values that may or may not be present.
For null checks, the Optional.ofNullable()
method can be used to wrap an object, and subsequent operations can be performed using methods like isPresent()
and ifPresent()
.
Code Example: Checking for Null Using the Optional
Class
Let’s dive into a practical example that demonstrates how to use the Optional
class for null checks. In this scenario, we have two classes, User1
and User2
, similar to the previous examples.
import java.util.Optional;
public class CheckNullWithOptional {
public static void main(String[] args) {
User2 user = new User2();
Optional<User1> optionalUser = Optional.ofNullable(user.getUser1Object());
if (optionalUser.isPresent()) {
System.out.println("Not Null");
User1 getUserObject = optionalUser.get();
getUserObject.setName("John Doe");
System.out.println("Name: " + getUserObject.getName());
} else {
System.out.println("Object is Null");
}
}
}
class User2 {
User1 user;
public User1 getUser1Object() {
return user;
}
}
class User1 {
String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
In this example, we import the Optional
class and use Optional.ofNullable()
to create an Optional
wrapper around the result of getUser1Object()
.
The isPresent()
method is then used within an if
statement to check if the object is not null. If the object is present, the program enters the if
block, prints Not Null
, and performs subsequent operations.
Within the if
block, we retrieve the actual object using optionalUser.get()
and then set the name using the setName
method. The updated name is printed on the console.
If the object is null, the program enters the else
block; printing Object is Null.
Code Output:
In this output, the message Object is Null
indicates that the Optional
class correctly identified the null object. Using the Optional
class promotes cleaner and safer code by encapsulating the null-checking logic within its methods, reducing the risk of NullPointerException
and improving the overall readability of the code.
Check for Null Objects in Java Using the Ternary Operator
The ternary operator (? :
) provides a concise and expressive way to perform conditional operations. Leveraging the ternary operator for null checks is a simple approach, offering a one-liner to determine whether an object is null
.
The ternary operator is a shorthand way of expressing an if-else
statement in a single line. Its syntax is:
condition ? expressionIfTrue : expressionIfFalse
In the context of null checks, the condition can be the object reference, and the expressions can handle the logic based on whether the object is null
or not.
Code Example: Checking for Null Using the Ternary Operator
Let’s delve into a practical example that demonstrates how to use the ternary operator for null checks. In this scenario, we have two classes, User1
and User2
, similar to the previous examples.
public class CheckNullWithTernaryOperator {
public static void main(String[] args) {
User2 user = new User2();
User1 getUserObject = user.getUser1Object();
String result = (getUserObject == null) ? "Object is Null" : "Not Null";
System.out.println(result);
if (getUserObject != null) {
getUserObject.setName("John Doe");
System.out.println("Name: " + getUserObject.getName());
}
}
}
class User2 {
User1 user;
public User1 getUser1Object() {
return user;
}
}
class User1 {
String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
In this code example, the ternary operator is used to perform a null check on the object reference getUserObject
. The expression (getUserObject == null) ? "Object is Null" : "Not Null"
is assigned to the variable result
.
If getUserObject
is null, the value Object is Null
is assigned to result
; otherwise, Not Null
is assigned.
Afterward, the result
is printed to the console, providing a clear indication of whether the object is null, additionally, within an if
block, further operations are performed if the object is not null.
In this case, the name of the User1
object is set using the setName
method, and the updated name is printed to the console.
Code Output:
In this output, the message Object is Null
indicates that the ternary operator successfully identified the null object. This method offers a concise and readable way to perform null checks, especially when the subsequent logic involves simple assignments or statements based on the nullity of the object.
Conclusion
In conclusion, Java provides several methods to check if an object is null
, each with its advantages and use cases.
The equality (==
) operator offers a straightforward and commonly used approach, comparing object references directly. The java.util.Objects.isNull()
method, introduced in Java 7, provides a null-safe alternative, enhancing readability and reducing the risk of errors associated with direct comparisons.
The equals
method, while primarily designed for equality checks, can be employed for null checks with caution, as it introduces the risk of NullPointerException
. The Optional
class, introduced in Java 8, offers an elegant and functional programming-style solution, encapsulating null-checking logic and promoting safer code.
Finally, the ternary operator (? :
) provides a concise one-liner for simple null checks. Choosing the appropriate method depends on the specific requirements and coding preferences.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn