How to Check if a File Exists in Java
-
Use
java.io.File
to Check if a File Exists in Java -
Use
isFile()
to Check if the File Exist in Java -
Use
Path.isFile()
WithisFile()
to Check if the File Exists or Not
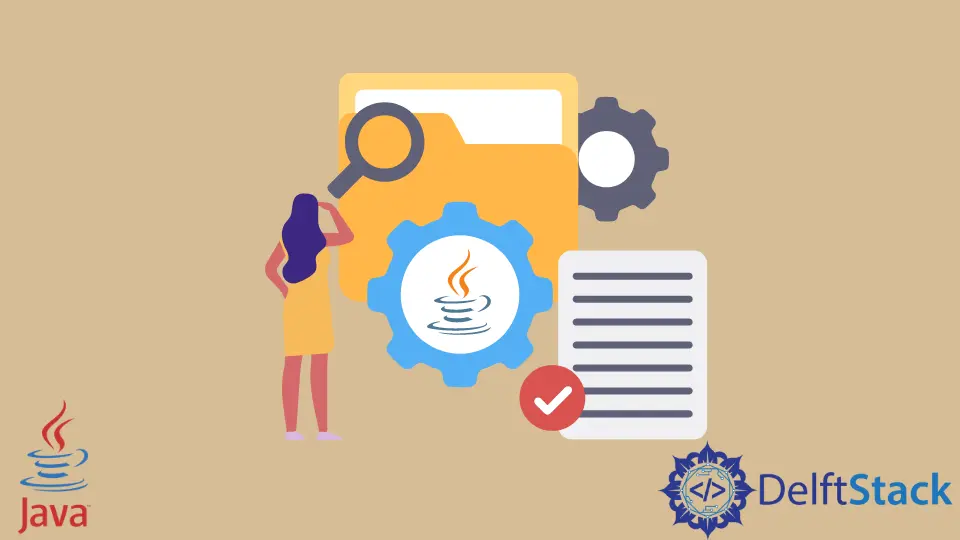
This article will look at a few simple ways in Java to check if a file exists. We will use different packages and classes when we want to know if the specified file exists.
Use java.io.File
to Check if a File Exists in Java
Java’s own Input/Output package java.io.File
has the exists()
method to check if the specified file exists. This function returns boolean
, which means that we can put it in a conditional statement.
But there is an issue with only using the exists()
method as it can also return true
if we accidentally specify a directory. It is why we will also use !file.isDirectory()
to ensure the given argument is a file but not a directory.
import java.io.File;
public class Main {
public static void main(String[] args) {
File file1 = new File("sampleFile.txt");
File file2 = new File("xyz.txt");
// Checks if file1 exists
if (file1.exists() && !file1.isDirectory()) {
System.out.println(file1 + " Exists");
} else {
System.out.println(file1 + " Does not exists");
}
// Checks if file2 exists
if (file2.exists()) {
System.out.println(file2 + " Exists");
} else {
System.out.println(file2 + " Does not exists");
}
}
}
Output:
sampleFile.txt Exists
xyz.txt Does not exists
Use isFile()
to Check if the File Exist in Java
The next method to check if the specified file exists is to use the isFile()
function of the same package java.io.File
that we used in the previous example.
The advantage of using isFile()
over exists()
is that we don’t have to check if the specified file is a directory or not. As the function name indicates, it only checks if it is a file or not.
import java.io.File;
public class Main {
public static void main(String[] args) {
File file = new File("sampleFile.txt");
File directory = new File("E:/Work/java");
if (file.isFile()) {
System.out.println(file + " Exists");
} else {
System.out.println(file + " Do not Exist or it is a directory");
}
if (directory.isFile()) {
System.out.println(directory + " Exists");
} else {
System.out.println(directory + " Do not Exist or it is a directory");
}
}
}
Output:
sampleFile.txt Exists
E:\Work\java Do not Exist or it is a directory
We can see that if an existing directory is given as the argument to the isFile()
function, it returns false
.
Use Path.isFile()
With isFile()
to Check if the File Exists or Not
Another Java package java.nio.file
provides us with useful methods like toFile()
and Paths
. We can get the path
using Paths.get()
and then convert it to file using toFile
.
Finally, we can bring back the method that we used in the last example, isFile()
, and combine it to check if the file exists.
import java.nio.file.Path;
import java.nio.file.Paths;
public class Main {
public static void main(String[] args) {
Path path = Paths.get("sampleFile.txt");
if (path.toFile().isFile()) {
System.out.println(path + " Exists");
} else {
System.out.println(path + " Do not Exists");
}
}
}
Output:
sampleFile.txt Exists
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn