How to Java Array Indexof
-
Get the Index of an Element in an
Integer
Type Array in Java - Get Index of an Array Element Using Java 8 Stream API in Java
-
Get Index of an Array Element Using
ArrayUtils.indexOf()
in Java -
Get Index of an Array Element Using
Arrays.binarySearch()
in Java -
Get Index of an Array Element Using a
for
Loop in Java - Conclusion
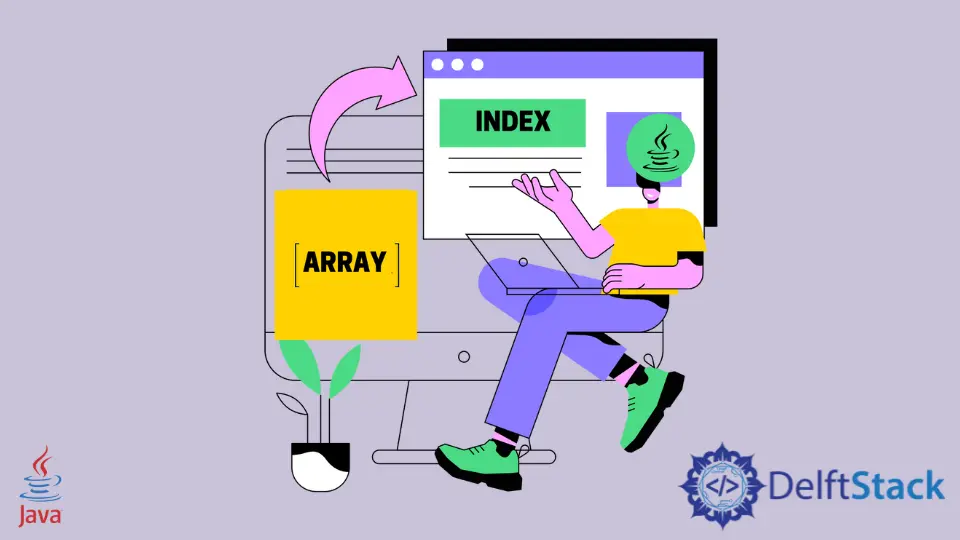
Arrays are a fundamental data structure in Java, and they allow you to store and manipulate collections of elements. Often, you’ll find yourself needing to find the index of a specific element within an array.
Whether you’re searching for a particular value or you need to perform some manipulation based on an element’s position, understanding how to get the index of an element in an array is an essential skill for Java developers.
In this article, we’ll explore various methods to achieve this task.
Get the Index of an Element in an Integer
Type Array in Java
In Java, when working with arrays, there is no built-in indexOf()
method, as you might find in other programming languages like JavaScript. However, you can achieve this functionality by converting your array to an ArrayList
and then using the indexOf()
method on the ArrayList
.
The ArrayList
class provides several utility methods to work with lists of objects, including finding the index of a specific element.
Here’s a breakdown of the steps involved:
-
First, create an array of objects. In the provided example, you will see that we have an array of
Integer
objects, which is an array of wrapper class types.It’s important to note that
Arrays.asList()
only accepts objects (not primitives likeint
), so using theInteger
wrapper class is necessary. However, the result will be of the primitive data typeint
. -
Use the
Arrays.asList()
method to convert the array to anArrayList
. This method is a convenient way to create a list from an array. -
With the array converted to an
ArrayList
, you can now use theindexOf()
method on theArrayList
to find the index of a specified element.
Here’s the code example:
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
public class ArrayIndexOf {
public static void main(String[] args) {
Integer[] array1 = {2, 4, 6, 8, 10};
List<Integer> arrayList = new ArrayList<>(Arrays.asList(array1));
int getIndex = arrayList.indexOf(8);
System.out.println("8 is located at index " + getIndex);
}
}
Output:
8 is located at index 3
In the code snippet above, we created an Integer
array named array1
, which contains a sequence of numbers.
To find the index of a specific element (in this case, 8
), we first converted the array1
into an ArrayList
using Arrays.asList(array1)
. This conversion is necessary because the indexOf()
method is not available for arrays directly; it’s a convenience method provided by ArrayList
.
We then called the indexOf(8)
method on the resulting ArrayList
. This method searches for the specified element (in this case, 8
) in the list and returns the index where the element is found.
In our example, the value of getIndex
will be 3
, as 8
is located at index 3
in the array.
Finally, we printed the result using System.out.println()
, displaying the index at which element 8
is located in the array.
It’s worth noting that this method works for reference types like Integer
, but for primitive types like int
, you’d need to use a loop to find the index. Additionally, if the element is not found in the list, the indexOf()
method will return -1
, indicating that the element is not present in the list.
Get Index of an Array Element Using Java 8 Stream API in Java
In Java, you can use the Stream API to efficiently filter out elements from an array and determine the position of a specified element. Specifically, IntStream
is an interface that allows you to perform Stream operations on primitive int data types, making it a valuable tool for processing arrays of integers.
Before we dive into the code, make sure you have Java 8 or a later version installed on your system. Java 8 introduced the Stream API, and it’s essential for this approach.
First, you need to have an array with the elements in which you want to find the index. Let’s assume we have an array of integers for this example:
int[] array = {10, 20, 30, 40, 50};
Next, specify the element for which you want to find the index. In this example, we’ll look for the index of the element with the value 30
:
int elementToFind = 30;
Now, we can use the Java 8 Stream API to find the index of the specified element in the array. Here’s how you can do it:
int index =
IntStream.range(0, array.length).filter(i -> array[i] == elementToFind).findFirst().orElse(-1);
Let’s break down this code to understand it better.
IntStream.range(0, array.length)
creates an IntStream
containing the indices from 0
to the length of the array minus one. It represents the indices of the elements in the array.
The .filter(i -> array[i] == elementToFind)
line of code contains the filter()
method that takes a predicate that checks whether the element at the current index (i
) is equal to the element we’re looking for (elementToFind
). It retains only the elements that satisfy this condition.
Next, the .findFirst()
method retrieves the first index of the element that matches the condition specified in the filter()
. Then, if no match is found, the orElse()
method returns -1
as a default value, indicating that the element is not in the array.
Finally, we can print the result to the console:
System.out.println("Index of " + elementToFind + " is " + index);
Here’s the complete Java program that finds the index of an element in an array using Java 8 Streams:
import java.util.stream.IntStream;
public class ArrayIndexOfWithStreams {
public static void main(String[] args) {
int[] array = {10, 20, 30, 40, 50};
int elementToFind = 30;
int index = IntStream.range(0, array.length)
.filter(i -> array[i] == elementToFind)
.findFirst()
.orElse(-1);
System.out.println("Index of " + elementToFind + " is " + index);
}
}
When you run the program, it will output:
Index of 30 is 2
In this example, the element with the value 30
is located at index 2
in the array.
This approach is particularly useful when working with large datasets or complex filtering conditions, as it provides a clear and functional way to search for elements in an array.
Get Index of an Array Element Using ArrayUtils.indexOf()
in Java
ArrayUtils
is a class from the Apache Commons Lang library, which is widely used for providing utility methods for common programming tasks. The ArrayUtils
class contains various methods for working with arrays, and one of the most helpful methods is indexOf()
.
This method enables us to find the index of a specified element within an array.
Before using ArrayUtils
, you need to include the Apache Commons Lang library in your project. You can download the library from the Apache Commons website or add it as a dependency using a build tool like Maven or Gradle.
For Maven, add the following dependency to your pom.xml
:
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.12.0</version> <!-- Replace with the latest version -->
</dependency>
Next, import the ArrayUtils
class and create an array in which you want to find the element. For this example, we’ll work with an array of integers:
import org.apache.commons.lang3.ArrayUtils;
public class ArrayIndexOfExample {
public static void main(String[] args) {
int[] array = {10, 20, 30, 40, 50};
}
}
Define the element for which you want to find the index. In this example, we’re searching for the index of the element with the value 30
:
int elementToFind = 30;
Now, you can use ArrayUtils.indexOf()
to find the index of the specified element:
int index = ArrayUtils.indexOf(array, elementToFind);
The ArrayUtils.indexOf()
method takes two arguments: the array to search in (in this case, array
) and the element to find (in this case, elementToFind
).
Finally, you can print the result to the console:
System.out.println("Index of " + elementToFind + " is " + index);
Here’s the complete Java program that finds the index of an element in an array using ArrayUtils.indexOf()
:
import org.apache.commons.lang3.ArrayUtils;
public class ArrayIndexOfExample {
public static void main(String[] args) {
int[] array = {10, 20, 30, 40, 50};
int elementToFind = 30;
int index = ArrayUtils.indexOf(array, elementToFind);
if (index != ArrayUtils.INDEX_NOT_FOUND) {
System.out.println("Index of " + elementToFind + " is " + index);
} else {
System.out.println("Element not found in the array.");
}
}
}
When you run the program, it will output:
Index of 30 is 2
In this example, the element with the value 30
is located at index 2
in the array.
It’s important to note that if the specified element is not found in the array, ArrayUtils.indexOf()
returns ArrayUtils.INDEX_NOT_FOUND
, which is a constant with a value of -1
. You can check this value to handle cases where the element is not present in the array.
Get Index of an Array Element Using Arrays.binarySearch()
in Java
The standard Java library provides a convenient method called Arrays.binarySearch()
for searching for an element in an array and determining its index. This method is designed for searching in sorted arrays and uses a binary search algorithm to efficiently locate elements.
It returns the index of the element if found or a negative value if not found.
To use Arrays.binarySearch()
, you must have a sorted array. This method will not work correctly on an unsorted array.
For this example, we’ll create a sorted array of integers:
import java.util.Arrays;
public class ArrayBinarySearchExample {
public static void main(String[] args) {
int[] sortedArray = {10, 20, 30, 40, 50};
}
}
Define the element you want to find the index for. In this example, we are searching for the index of the element with the value 30
:
int elementToFind = 30;
Now, you can use the Arrays.binarySearch()
method to find the index of the specified element:
int index = Arrays.binarySearch(sortedArray, elementToFind);
The Arrays.binarySearch()
method takes two arguments: the sorted array to search in (in this case, sortedArray
) and the element to find (in this case, elementToFind
).
Before displaying the result, you need to check the return value of Arrays.binarySearch()
. It will return the index of the element (if found), or it will return a negative value (if not found).
To handle both cases, you can use conditional statements:
if (index >= 0) {
System.out.println("Index of " + elementToFind + " is " + index);
} else {
System.out.println("Element not found in the array.");
}
Here’s the complete Java program that finds the index of an element in a sorted array using Arrays.binarySearch()
:
import java.util.Arrays;
public class ArrayBinarySearchExample {
public static void main(String[] args) {
int[] sortedArray = {10, 20, 30, 40, 50};
int elementToFind = 30;
int index = Arrays.binarySearch(sortedArray, elementToFind);
if (index >= 0) {
System.out.println("Index of " + elementToFind + " is " + index);
} else {
System.out.println("Element not found in the array.");
}
}
}
When you run the program, it will output:
Index of 30 is 2
In this example, the element with the value 30
is located at index 2
in the sorted array.
If the specified element is not present in the sorted array, Arrays.binarySearch()
will return a negative value. In such cases, you can use the conditional statement to handle the element not found
scenario gracefully.
Get Index of an Array Element Using a for
Loop in Java
While there are more advanced techniques available, using a simple for
loop can be a widely applicable method to determine the index of a specific element within an array.
First, you need an array in which you want to find the element’s index. Let’s create an array of integers for this example:
public class ArrayIndexOfExample {
public static void main(String[] args) {
int[] array = {10, 20, 30, 40, 50};
}
}
Next, specify the element for which you want to find the index. In this example, we’ll look for the index of the element with the value 30
:
int elementToFind = 30;
Now, you can use a for
loop to iterate through the elements in the array and check if each element matches the one you’re looking for. You’ll need a loop counter to keep track of the current index as you iterate through the array:
int index = -1; // Initialize the index to -1 (not found)
for (int i = 0; i < array.length; i++) {
if (array[i] == elementToFind) {
index = i;
break; // Exit the loop as soon as the element is found
}
}
In this code, the for
loop iterates through the elements in the array, and the if
statement checks if the current element matches the one you’re looking for.
If a match is found, the loop breaks and the index is set to the current value of i
. If no match is found, the index remains at -1
.
Finally, you can print the result to the console. The index will indicate the position of the element within the array, or it will remain -1
if the element is not found.
if (index != -1) {
System.out.println("Index of " + elementToFind + " is " + index);
} else {
System.out.println("Element not found in the array.");
}
Here’s the complete Java program that finds the index of an element in an array using a for
loop:
public class ArrayIndexOfExample {
public static void main(String[] args) {
int[] array = {10, 20, 30, 40, 50};
int elementToFind = 30;
int index = -1;
for (int i = 0; i < array.length; i++) {
if (array[i] == elementToFind) {
index = i;
break;
}
}
if (index != -1) {
System.out.println("Index of " + elementToFind + " is " + index);
} else {
System.out.println("Element not found in the array.");
}
}
}
Output:
Index of 30 is 2
In this example, the element with the value 30
is located at index 2
in the array.
Conclusion
When it comes to finding the index of an element in an array in Java, you have several options at your disposal, each with its advantages. The choice of method depends on your specific requirements and coding style.
When working with dynamic collections, such as ArrayList
, the indexOf
method is both efficient and easy to use.
For a more modern and functional programming approach, Java 8’s Stream API offers an elegant and concise way to find an element’s index in an array.
If you’re looking for a convenient and versatile solution that works well with different data types and offers additional array manipulation functions, Apache Commons Lang’s ArrayUtils.indexOf()
is a valuable tool to have in your toolbox.
If you prefer a traditional, straightforward approach and are working with basic arrays, using a for
loop is a reliable and easy-to-understand solution.
Finally, if you’re dealing with a sorted array and want to maximize efficiency, Java’s Arrays.binarySearch
method is the way to go, as it leverages the power of binary search.
All these methods are effective in finding the index of an element in an array in Java; choose the one that suits you best.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn