Java Jagged Array
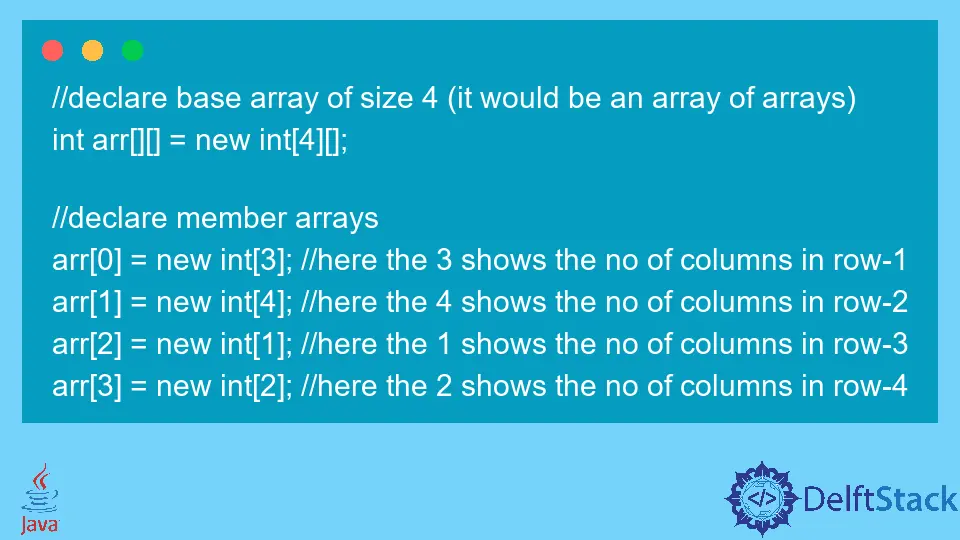
This tutorial educates about the Java jagged array. We will learn about its memory representation and implementation via different code examples.
Java Jagged Array
To understand the jagged array, you must have a good understanding of the arrays. A jagged array, also known as a ragged array, is an array of arrays where every member array is of a different size.
Here, an array of arrays means a two-dimensional array; it can be 2D or 3D or with more dimensions. See the following visual demonstration to understand the jagged array.
We can see that the size of each member array differs from one another. This is what we call a Jagged or Ragged array.
Declaration and Initialization of Jagged Array in Java
There are various ways to declare and initialize the jagged array; we will see each of them below using the int
type array extracted from the visual explanation.
Using the first approach, we declare the base array first by specifying the size. Then, we write different sizes for each member array.
Here, we are separating the declaration and initialization process. See the following snippet.
Declare Jagged Array:
// declare base array of size 4 (it would be an array of arrays)
int arr[][] = new int[4][];
// declare member arrays
arr[0] = new int[3]; // here the 3 shows the no of columns in row-1
arr[1] = new int[4]; // here the 4 shows the no of columns in row-2
arr[2] = new int[1]; // here the 1 shows the no of columns in row-3
arr[3] = new int[2]; // here the 2 shows the no of columns in row-4
Next, initialize the jagged array statically.
arr[0] = new int[] {1, 2, 3};
arr[1] = new int[] {1, 2, 3, 4};
arr[2] = new int[] {4};
arr[3] = new int[] {4, 5};
Alternatively, we can initialize the jagged array dynamically, which means we take the input from a user and initialize the arrays at run-time.
Scanner sc = new Scanner(System.in);
for (int base = 0; base < arr.length; base++) {
for (int member = 0; member < arr[base].length; member++) {
arr[base][member] = sc.nextInt();
}
}
In the second approach, we can declare and initialize the jagged array in one step. We can write this one step in various ways.
See the code snippet given below.
int arr[][] =
new int[][] {new int[] {1, 2, 3}, new int[] {1, 2, 3, 4}, new int[] {4}, new int[] {4, 5}};
OR
int[][] arr = {new int[] {1, 2, 3}, new int[] {1, 2, 3, 4}, new int[] {4}, new int[] {4, 5}};
OR
int[][] arr = {{1, 2, 3}, {1, 2, 3, 4}, {4}, {4, 5}};
Let’s dive into a more detailed implementation of the jagged array where we will assign values statically and dynamically.
Jagged Array Implementation Examples in Java
Example Code (Jagged Array is Initialized Statically):
public class jagggedArrayTest {
public static void main(String args[]) {
int arr[][] =
new int[][] {new int[] {1, 2, 3}, new int[] {1, 2, 3, 4}, new int[] {4}, new int[] {4, 5}};
for (int base = 0; base < arr.length; base++) {
System.out.print("arr[" + base + "] ======> {");
for (int member = 0; member < arr[base].length; member++) {
if (member < arr[base].length - 1)
System.out.print(arr[base][member] + ", ");
else
System.out.print(arr[base][member]);
}
System.out.print("}");
System.out.println();
}
}
}
Output:
arr[0] ======> {1, 2, 3}
arr[1] ======> {1, 2, 3, 4}
arr[2] ======> {4}
arr[3] ======> {4, 5}
We first declare and initialize the jagged array in the main
function. Then, we use a nested for
loop to print the jagged array where the outer loop is to iterate over the base array (rows), and the inner loop is used to iterate over the member arrays (columns).
Example Code (Jagged Array is Populated Dynamically):
import java.util.Scanner;
public class jaggedArrayTest {
/*
this function prints the populated jagged array
*/
static void printJaggedArray(int[][] arr) {
System.out.println("The populated array looks like as follows:");
for (int base = 0; base < arr.length; base++) {
System.out.print("arr[" + base + "] ======> {");
for (int member = 0; member < arr[base].length; member++) {
if (member < arr[base].length - 1)
System.out.print(arr[base][member] + ", ");
else
System.out.print(arr[base][member]);
}
System.out.print("}");
System.out.println();
}
}
/*
this function populates the jagged array by
taking input from the user
*/
static void populateJaggedArray(int[][] arr) {
Scanner sc = new Scanner(System.in);
for (int base = 0; base < arr.length; base++) {
System.out.println("Enter the member array at index " + base);
for (int member = 0; member < arr[base].length; member++) {
arr[base][member] = sc.nextInt();
}
}
// print jagged array
printJaggedArray(arr);
}
public static void main(String args[]) {
// declare base array of size 4 (it would be an array of arrays)
int arr[][] = new int[4][];
// declare member arrays
arr[0] = new int[3]; // here the 3 shows the no of columns in row-1
arr[1] = new int[4]; // here the 4 shows the no of columns in row-2
arr[2] = new int[1]; // here the 1 shows the no of columns in row-3
arr[3] = new int[2]; // here the 2 shows the no of columns in row-4
// populate jagged array
populateJaggedArray(arr);
}
}
Output:
Enter the member array at index 0
1 2 3
Enter the member array at index 1
1 2 3 4
Enter the member array at index 2
4
Enter the member array at index 3
4 5
The populated array looks like as follows:
arr[0] ======> {1, 2, 3}
arr[1] ======> {1, 2, 3, 4}
arr[2] ======> {4}
arr[3] ======> {4, 5}
Here, we have three methods named main()
, populateJaggedArray()
, and the printJaggedArray()
inside the jaggedArrayTest
class. The main()
method declares and initialize the jagged array which is passed to the populateJaggedArray()
function to get populated.
Further, it calls the printJaggedArray()
to print the populated jagged array. Remember, we are only populating the jagged array dynamically, but you can also take the size of the base and member arrays using the user’s input values.