Integer Division in Java
Rashmi Patidar
Oct 12, 2023
Java
Java Integer
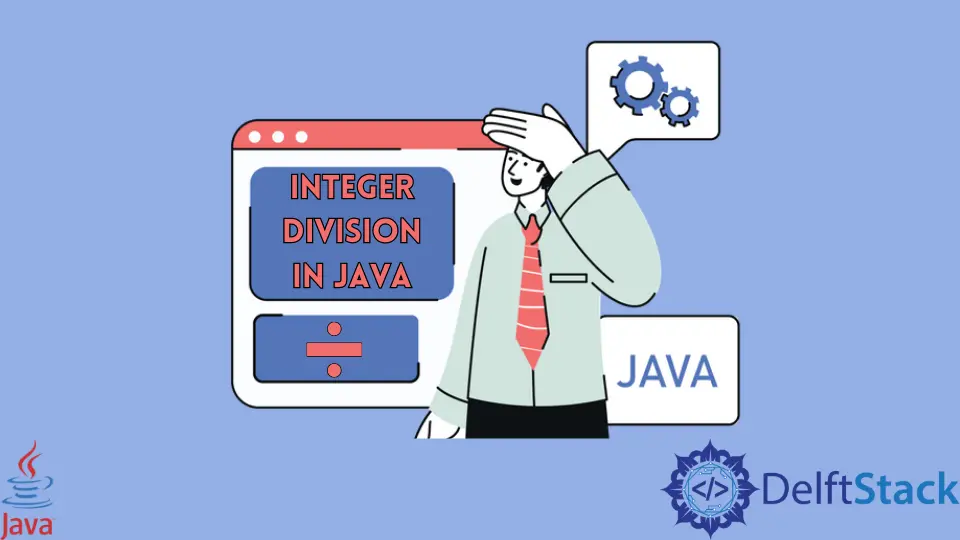
The article introduces a detailed explanation of what internally happens when we divide two integers.
In the below Java code, there are four java statements. Each line prints different output based on the numbers that divide from each other.
package integer_division;
public class IntegerDivision {
public static void main(String[] args) {
System.out.println(10 / 9);
System.out.println(-10 / 9);
System.out.println(10 / -9);
System.out.println(10 / 19);
System.out.println((float) 10 / 9);
System.out.println((double) 10 / 9);
}
}