How to Initialize List of String in Java
-
Use
ArrayList
,LinkedList
andVector
to Instantiate a List of String in Java -
Use
Arrays.asList
to Instantiate aList
of String in Java -
Use
Stream
in Java 8 to Instantiate aList
of String in Java -
Use
List.of
to Instantiate aList
of String in Java
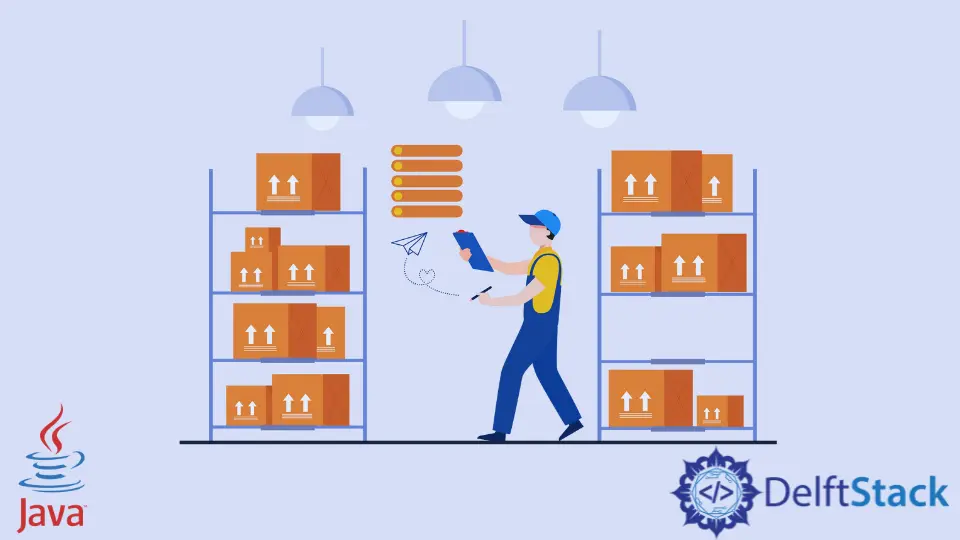
In this tutorial, we will see various ways in which we can Initialize a list of string in Java. Since the list is an interface, we can not directly instantiate it.
Use ArrayList
, LinkedList
and Vector
to Instantiate a List of String in Java
A List
is a child interface of Collections
in Java. It is an ordered collection of objects which can store duplicate values. The instance of List
can be created using classes that implement the List
Interface.
ArrayList
, Vector
, LinkedList
and Stack
are a few of these classes. We create an instance myList
of a List
using new ArraList<String>()
. Hence, we can declare and create an instance of List
using any of the following ways shown below and perform various operations on that List
.
import java.util.ArrayList;
import java.util.LinkedList;
import java.util.List;
import java.util.Vector;
public class ListExample {
public static void main(String[] args) {
List<String> myList = new ArrayList<String>();
List<Fruits> lList = new LinkedList<Fruits>();
List<Integer> vList = new Vector<Integer>();
myList.add("Happy");
myList.add("Coding");
lList.add(new Fruits("Grapes", "Green"));
lList.add(new Fruits("Apple", "Red"));
vList.add(1);
vList.add(4);
vList.add(9);
vList.add(7);
vList.remove(2);
for (String s : myList) {
System.out.println(s);
}
for (Fruits f : lList) {
System.out.println(f.name + " is " + f.color + " in color.");
}
for (int i : vList) {
System.out.println(i);
}
}
}
class Fruits {
String name;
String color;
Fruits(String name, String color) {
this.name = name;
this.color = color;
}
}
Output:
Happy
Coding
Grapes is Green in color.
Apple is Red in color.
1
4
7
Use Arrays.asList
to Instantiate a List
of String in Java
The Arrays.asList
method returns a fixed-size list that is backed by an array. This is just a wrapper that makes the array available as a list. We can not modify this list as it is immutable.
Here in the code, we get an instance of List
named myList
whose length can not be modified.
import java.util.Arrays;
import java.util.List;
public class ListExmp {
public static void main(String[] args) {
List<String> myList = Arrays.asList("John", "Ben", "Gregor", "Peter");
String name = myList.get(3);
System.out.println(name);
}
}
Output:
Peter
Use Stream
in Java 8 to Instantiate a List
of String in Java
Java 8 Stream
includes wrappers around a data source that makes a bulky process on the data easy and convenient.
The Stream.of()
method constructs a stream of data and collects them in a list. The Collector
interface provides the logic for this operation. The Collector.toList()
collects all the stream elements into an instance of List
.
import java.util.List;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class ListExmp {
public static void main(String[] args) {
List<String> list = Stream.of("john", "doe").collect(Collectors.toList());
if (list.contains("doe")) {
System.out.println("doe is there");
} else {
System.out.println("doe is not there");
}
}
}
Output:
doe is there
Use List.of
to Instantiate a List
of String in Java
The List.of
is the new method introduced in Java 9. In the code below, the List.of()
method takes any number of arguments and returns an immutable list. We have immutableList
as an unmodifiable instance of List
.
We have to instantiate ArrayList
with an immutable list as a parameter to create a mutable list. As shown in the code below, modifications can be made to the mutableList
instance of the List
.
import java.util.ArrayList;
import java.util.List;
public class ListExmp {
public static void main(String[] args) {
List<String> immutableList = List.of("One", "Two", "Three", "Four", "Five");
List<String> mutableList = new ArrayList<>(List.of("Six", "Seven", "Eight"));
for (String l : immutableList) {
System.out.println(l);
}
System.out.println("XXXXXX");
mutableList.add("Nine");
for (String l : mutableList) {
System.out.println(l);
}
}
}
Output:
One
Two
Three
Four
Five
XXXXXX
Six
Seven
Eight
Nine
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn