How to Sort Array Elements in Java
-
Sort Array Elements Using the
sort()
Method in Java - Sort Array Elements in Java
-
Sort an Array Elements Using
parallelSort()
Method in Java -
Sort an Array Elements Using
parallelSort()
Method in Java -
Sort an Array Elements Using
parallelSort()
Method in Java
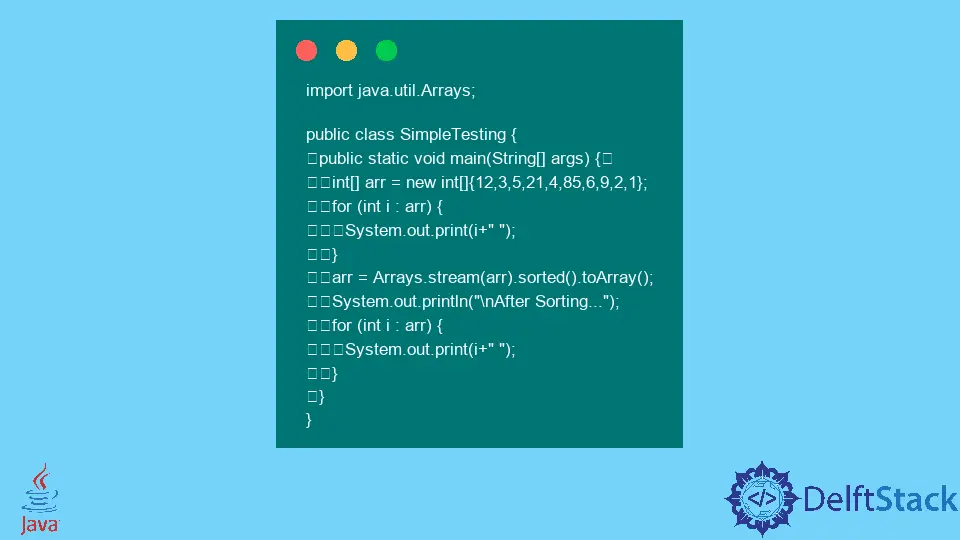
This tutorial introduces how to sort array elements in Java and lists some example codes to understand it.
There are several ways to sort an array elements, like Arrays.sort()
, sorted()
, and parallelSort()
methods, etc. Let’s see the examples.
Sort Array Elements Using the sort()
Method in Java
Here, we use the sort()
method of the Arrays
class to sort the elements. This method sorts the elements in ascending order. See the example below.
import java.util.Arrays;
public class SimpleTesting {
public static void main(String[] args) {
int[] arr = new int[] {12, 3, 5, 21, 4, 85, 6, 9, 2, 1};
for (int i : arr) {
System.out.print(i + " ");
}
Arrays.sort(arr);
System.out.println("\nAfter Sorting...");
for (int i : arr) {
System.out.print(i + " ");
}
}
}
Output:
12 3 5 21 4 85 6 9 2 1
After Sorting...
1 2 3 4 5 6 9 12 21 85
Sort Array Elements in Java
If we don’t want to use any built-in method of Java, then use this code that will sort array elements into ascending order.
public class SimpleTesting {
public static void main(String[] args) {
int[] arr = new int[] {12, 3, 5, 21, 4, 85, 6, 9, 2, 1};
for (int i : arr) {
System.out.print(i + " ");
}
for (int i = 0; i < arr.length; i++) {
for (int j = 0; j < arr.length; j++) {
if (arr[i] < arr[j]) {
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
}
System.out.println("\nAfter Sorting...");
for (int i : arr) {
System.out.print(i + " ");
}
}
}
Output:
12 3 5 21 4 85 6 9 2 1
After Sorting...
1 2 3 4 5 6 9 12 21 85
Sort an Array Elements Using parallelSort()
Method in Java
If you are working with Java 8 or higher version, you can use the parallelSort()
method of Arrays class. This method is useful for sorting in a multithreading environment. See the example below.
import java.util.Arrays;
public class SimpleTesting {
public static void main(String[] args) {
int[] arr = new int[] {12, 3, 5, 21, 4, 85, 6, 9, 2, 1};
for (int i : arr) {
System.out.print(i + " ");
}
Arrays.parallelSort(arr);
System.out.println("\nAfter Sorting...");
for (int i : arr) {
System.out.print(i + " ");
}
}
}
Output:
12 3 5 21 4 85 6 9 2 1
After Sorting...
1 2 3 4 5 6 9 12 21 85
Sort an Array Elements Using parallelSort()
Method in Java
Java provides one overloaded method of parallelSort()
to sort sub-array. It means we can sort some elements of an array from a specific index to another specific index (end index). This method takes three arguments; one is an array, second and third is start and end index of the array to sort. See the example below.
import java.util.Arrays;
public class SimpleTesting {
public static void main(String[] args) {
int[] arr = new int[] {12, 3, 5, 21, 4, 85, 6, 9, 2, 1};
for (int i : arr) {
System.out.print(i + " ");
}
Arrays.parallelSort(arr, 0, 5);
System.out.println("\nAfter Sorting...");
for (int i : arr) {
System.out.print(i + " ");
}
}
}
Output:
12 3 5 21 4 85 6 9 2 1
After Sorting...
3 4 5 12 21 85 6 9 2 1
Sort an Array Elements Using parallelSort()
Method in Java
If you want to use the stream feature of Java, then use the sorted()
method that will sort the elements, and by using toArray()
, we collect elements into an array.
import java.util.Arrays;
public class SimpleTesting {
public static void main(String[] args) {
int[] arr = new int[] {12, 3, 5, 21, 4, 85, 6, 9, 2, 1};
for (int i : arr) {
System.out.print(i + " ");
}
arr = Arrays.stream(arr).sorted().toArray();
System.out.println("\nAfter Sorting...");
for (int i : arr) {
System.out.print(i + " ");
}
}
}
Output:
12 3 5 21 4 85 6 9 2 1
After Sorting...
1 2 3 4 5 6 9 12 21 85