How to Remove the Last Character From the String in Java
-
Remove the Last Character From the String Using the
substring()
Method in Java -
Remove the Last Character From the String Using the
replaceFirst()
Method in Java -
Remove the Last Character From the String Using the
removeEnd()
Method in Java -
Remove the Last Character From the String Using the
chop()
Method in Java -
Remove the Last From the String Using the
substring()
Method in Java - Remove the Last Character From the String in Java 8 or Higher
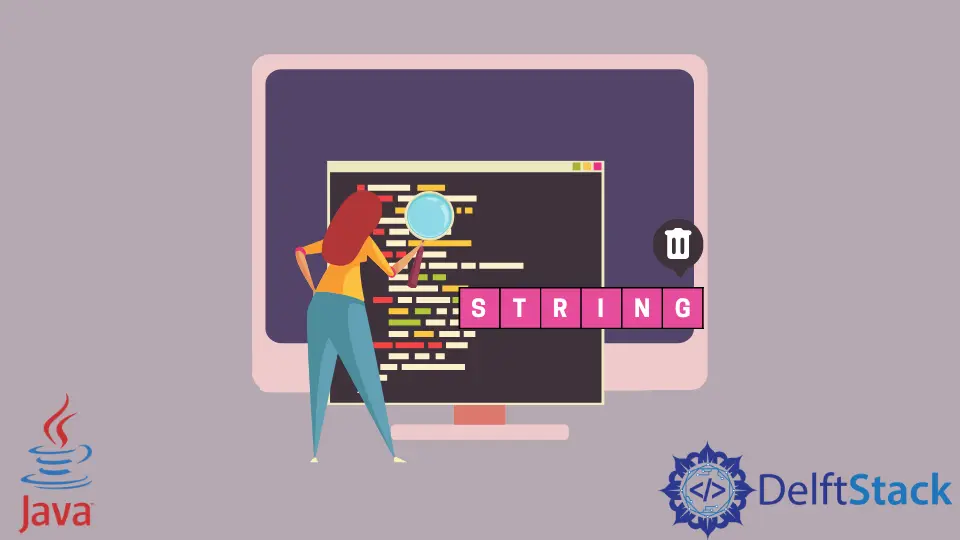
This tutorial introduces how to remove the last character from the string in Java. There are several ways to remove the last char, such as the substring()
method, the StringUtils
class, etc. Let’s take a close look at the examples.
Remove the Last Character From the String Using the substring()
Method in Java
The substring()
method of String
class is used to fetch substring from a string object. It takes two arguments: the start index and the last index. Here, we use the substring()
method to get a string after excluding the last char. See the example below.
public class SimpleTesting {
public static void main(String args[]) {
String str = "removea";
str = str.substring(0, str.length() - 1);
System.out.println(str);
}
}
Output:
remove
Remove the Last Character From the String Using the replaceFirst()
Method in Java
We can use the replaceFirst()
method to remove the last char from the string. It returns a string after replacing the char. See the example below.
public class SimpleTesting {
public static void main(String args[]) {
String str = "removea";
str = str.replaceFirst(".$", "");
System.out.println(str);
}
}
Output:
remove
Remove the Last Character From the String Using the removeEnd()
Method in Java
If you are working with the Apache commons lang
library, it is best to use the StringUtils
class that provides the removeEnd()
method to remove the string’s last char. See the example below.
import org.apache.commons.lang3.StringUtils;
public class SimpleTesting {
public static void main(String args[]) {
String str = "removea";
str = StringUtils.removeEnd(str, "a");
System.out.println(str);
}
}
Output:
remove
Remove the Last Character From the String Using the chop()
Method in Java
Another method, chop()
of the StringUtils
class, returns a string after removing the last char from the specified string.
import org.apache.commons.lang3.StringUtils;
public class SimpleTesting {
public static void main(String args[]) {
String str = "removea";
str = StringUtils.chop(str);
System.out.println(str);
}
}
Output:
remove
Remove the Last From the String Using the substring()
Method in Java
The StringUtils
class provides the substring()
method that takes three arguments. The first is a string, the second is a start index, and the third is an end index of the string. It returns a string, which is a substring of the specified string. See the example below.
import org.apache.commons.lang3.StringUtils;
public class SimpleTesting {
public static void main(String args[]) {
String str = "removea";
str = StringUtils.substring(str, 0, -1);
System.out.println(str);
}
}
Output:
remove
Remove the Last Character From the String in Java 8 or Higher
If you are using a higher version of Java, you can use the Optional
class to avoid the null exception and functional approach to remove the last char from the string.
import java.util.Optional;
public class SimpleTesting {
public static void main(String args[]) {
String str = "removea";
str = Optional.ofNullable(str)
.filter(s -> s.length() != 0)
.map(s -> s.substring(0, s.length() - 1))
.orElse(str);
System.out.println(str);
}
}
Output:
remove