How to Get the Separate Digits of an Int Number in Java
-
%
(mod
) to Get the Remainder of the Given Integer Number -
String.toCharArray()
to Get the Array of Characters -
number.split("(?<=.)")
Method to Get the String Array and Then Split Them - Separate Digits From an Int Using Recursion
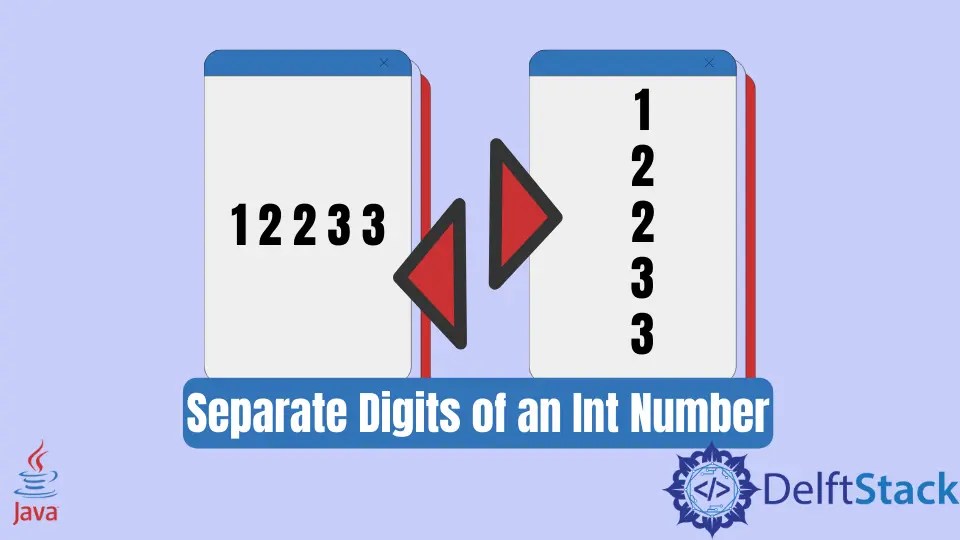
Java offers a lot of methods to work with integers. We will see how we can extract and separate every single digit from an int number.
%
(mod
) to Get the Remainder of the Given Integer Number
We can get every single digit of an integer number by using the remainder method. Java allows us to get the remainder of any integer number using the %
(mod) operator.
But merely getting the remainder will give us the result in the reverse order. It is why we will use a LinkedList
stack. In which we will push every single reminder and then pop one by one, providing us with the desired result.
import java.util.LinkedList;
public class Main {
public static void main(String[] args) {
int number = 12223;
LinkedList<Integer> stack = new LinkedList<Integer>();
while (number > 0) {
stack.push(number % 10);
number = number / 10;
}
while ((!stack.isEmpty())) {
System.out.println(stack.pop());
}
}
}
Output:
1
2
2
2
3
String.toCharArray()
to Get the Array of Characters
Another way of separating the digits from an int number is using the toCharArray()
method.
We will convert the integer number into a string and then use the string’s toCharArray()
to get the characters’ array. Now we can print out all the characters one by one. Later we can convert the characters back into the integer format.
public class Main {
public static void main(String[] args) {
int number1 = 12223;
String number = String.valueOf(number1);
char[] digits1 = number.toCharArray();
for (int i = 0; i < digits1.length; i++) {
System.out.println(digits1[i]);
}
}
}
Output:
1
2
2
2
3
number.split("(?<=.)")
Method to Get the String Array and Then Split Them
This method is similar to the above one, but here we are using split
, a function of String
.
public class Main {
public static void main(String[] args) {
int number1 = 12223;
String number = String.valueOf(number1);
String[] digits = number.split("(?<=.)");
for (int i = 0; i < digits.length; i++) {
System.out.println(digits[i]);
}
}
}
Output:
1
2
2
2
3
Separate Digits From an Int Using Recursion
We can use the recursion technique to get the digits out of an int in a more straightforward way.
The recursion()
method takes the number as an argument and then calls itself by dividing the number with 10. It is the number of times the method will be called.
public class Main {
public static void main(String[] args) {
recursion(12223);
}
public static void recursion(int number) {
if (number > 0) {
recursion(number / 10);
System.out.printf(number % 10);
}
}
}
Output:
1
2
2
2
3
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn