How to Get the Current Working Directory in Java
-
Get Current Working Directory Using
System.getProperty()
Method in Java -
Get Current Working Directory Using
toAbsolutePath()
in Java -
Get Current Working Directory Using
normalize()
Method in Java -
Get Current Working Directory Using
FileSystems.getDefault()
Method in Java -
Get Current Working Directory Using
getAbsoluteFile()
Method in Java -
Get Current Working Directory Using
getClass()
Method in Java
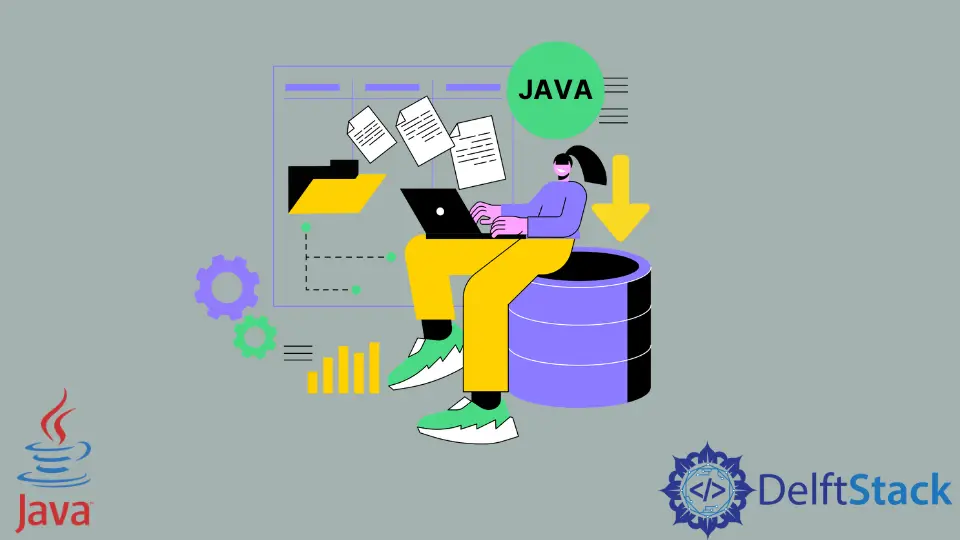
This tutorial introduces how to get the current working directory and lists some example codes to understand it.
There are several ways to get the current working directory, here we use System.getProperty()
, toAbsolutePath()
, and FileSystems.getDefault()
method, etc. Let’s see the examples.
Get Current Working Directory Using System.getProperty()
Method in Java
The System.getProperty()
method is that returns the system property indicated by the key (argument). For example, user.dir
returns the user directory and os.name
returns the name of the operating system. Here, we use this method to get the current user working directory. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
String directoryName = System.getProperty("user.dir");
System.out.println("Current Working Directory is = " + directoryName);
}
}
Output:
Current Working Directory is = D:\eclipse-workspace\corejavaexamples
Get Current Working Directory Using toAbsolutePath()
in Java
The toAbsolutePath()
method can get the absolute path of any location. Here, we use this method to get the absolute path of the current directory. See the example below.
import java.nio.file.Path;
import java.nio.file.Paths;
public class SimpleTesting {
public static void main(String[] args) {
Path path = Paths.get("");
String directoryName = path.toAbsolutePath().toString();
System.out.println("Current Working Directory is = " + directoryName);
}
}
Output:
Current Working Directory is = D:\eclipse-workspace\corejavaexamples
Get Current Working Directory Using normalize()
Method in Java
The normalize()
method returns a path from the current path in which all redundant name elements are eliminated. We use this method with the toAbsolute()
method to get the current working directory by eliminating the redundant names. See the example below.
import java.nio.file.Path;
import java.nio.file.Paths;
public class SimpleTesting {
public static void main(String[] args) {
Path path = Paths.get("");
String directoryName = path.toAbsolutePath().normalize().toString();
System.out.println("Current Working Directory is = " + directoryName);
}
}
Output:
Current Working Directory is = D:\eclipse-workspace\corejavaexamples
Get Current Working Directory Using FileSystems.getDefault()
Method in Java
We can use getDefault()
method of FileSystem
class to get default Filesystem
and then the toAbsolutePath()
method to get the absolute path of current working directory. See the example below.
import java.nio.file.FileSystems;
import java.nio.file.Path;
public class SimpleTesting {
public static void main(String[] args) {
Path path = FileSystems.getDefault().getPath("");
String directoryName = path.toAbsolutePath().toString();
System.out.println("Current Working Directory is = " + directoryName);
}
}
Output:
Current Working Directory is = D:\eclipse-workspace\corejavaexamples
Get Current Working Directory Using getAbsoluteFile()
Method in Java
We can use the getAbsoluteFile()
method to get the location of the current file that actually represents the location of the current directory. See the example below.
import java.io.File;
public class SimpleTesting {
public static void main(String[] args) {
File file = new File("");
String directoryName = file.getAbsoluteFile().toString();
System.out.println("Current Working Directory is = " + directoryName);
}
}
Output:
Current Working Directory is = D:\eclipse-workspace\corejavaexamples
Get Current Working Directory Using getClass()
Method in Java
We can use the getClass()
method of Object class in Java that returns the currently running class, which further can be used with the getPath()
method to get the path of the current working directory. See the example below.
public class SimpleTesting {
String getCurrentDirectory() {
return this.getClass().getClassLoader().getResource("").getPath();
}
public static void main(String[] args) {
String directoryName = new SimpleTesting().getCurrentDirectory();
System.out.println("Current Working Directory is = " + directoryName);
}
}
Output:
Current Working Directory is = D:\eclipse-workspace\corejavaexamples