How to Create a File and Write Data to It in Java
-
PrintWriter
to Create File in Java -
Files
to Create File in Java -
BufferedWriter
to Create File in Java -
FileWriter
to Create File in Java
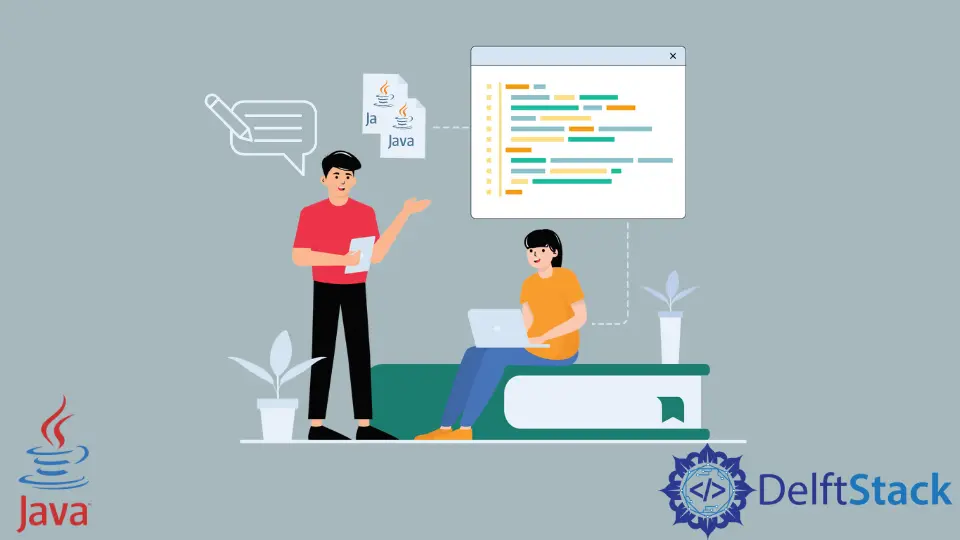
This tutorial discusses methods to create a text file and write data to it in Java.
Java has multiple methods to create a file. The one thing to remember is that the try-catch
block is necessary for almost all of the file creation methods so that any IO Exception is gracefully handled. Do not forget to use it.
PrintWriter
to Create File in Java
The PrintWriter
in Java allows you to create a new file with the specified encoding. Here is an example.
import java.io.*;
public class Main {
public static void main(String[] args) {
String fileName = "my-file.txt";
String encoding = "UTF-8";
try {
PrintWriter writer = new PrintWriter(fileName, encoding);
writer.println("The first line");
writer.println("The second line");
writer.close();
} catch (IOException e) {
System.out.println("An error occurred.");
e.printStackTrace();
}
}
}
Files
to Create File in Java
We can also use java.nio.file
to create a file and write data to it. The below example illustrates this approach.
import java.io.*;
import java.nio.charset.*;
import java.nio.file.*;
import java.util.*;
public class Main {
public static void main(String[] args) {
String fileName = "my-file.txt";
try {
List<String> lines = Arrays.asList("The first line", "The second line");
Path file = Paths.get(fileName);
Files.write(file, lines, StandardCharsets.UTF_8);
} catch (IOException e) {
System.out.println("An error occurred.");
e.printStackTrace();
}
}
}
In this example, we write the data from an ArrayList
to the file such that every element in ArrayList
is written to a new line in the output file. The output file looks like this:
> The first line
> The second line
BufferedWriter
to Create File in Java
The below example illustrates creating and writing to a file using BufferedWriter
.
import java.io.*;
import java.nio.charset.*;
import java.util.*;
public class Main {
public static void main(String[] args) {
Charset utf8 = StandardCharsets.UTF_8;
List<String> list = Arrays.asList("This is the first line", "This is the second line");
String content = "This is my content";
try {
// File name with path
String fileName = "my-file.txt";
File myFile = new File(fileName);
FileOutputStream fos = new FileOutputStream(myFile);
OutputStreamWriter osw = new OutputStreamWriter(fos);
Writer writer = new BufferedWriter(osw);
// Write data using a String variable
writer.write(content + "\n");
// Write data from an ArrayList
for (String s : list) {
writer.write(s + "\n");
}
} catch (IOException e) {
System.out.println("An error occurred.");
e.printStackTrace();
}
}
}
The output file looks like this:
> This is my content
> This is the first line
> This is the second line
FileWriter
to Create File in Java
Another common method to create a new file and write to it in Java is to use FileWriter
.
import java.io.*;
public class Main {
public static void main(String[] args) {
try {
FileWriter myWriter = new FileWriter("my-file.txt");
myWriter.write("We can also create a file and write to it using FileWriter");
myWriter.close();
} catch (IOException e) {
System.out.println("An error occurred.");
e.printStackTrace();
}
}
}
The output file looks like this:
> We can also create a file and write to it using FileWriter