How to Convert Hashmap to JSON Object in Java
-
new JSONObject(hashmap)
to Convert Hashmap to JSON Object - Jackson Library to Convert Hashmap Into a JSON Object
- GSON Library to Convert the Hashmap Into JSON Object
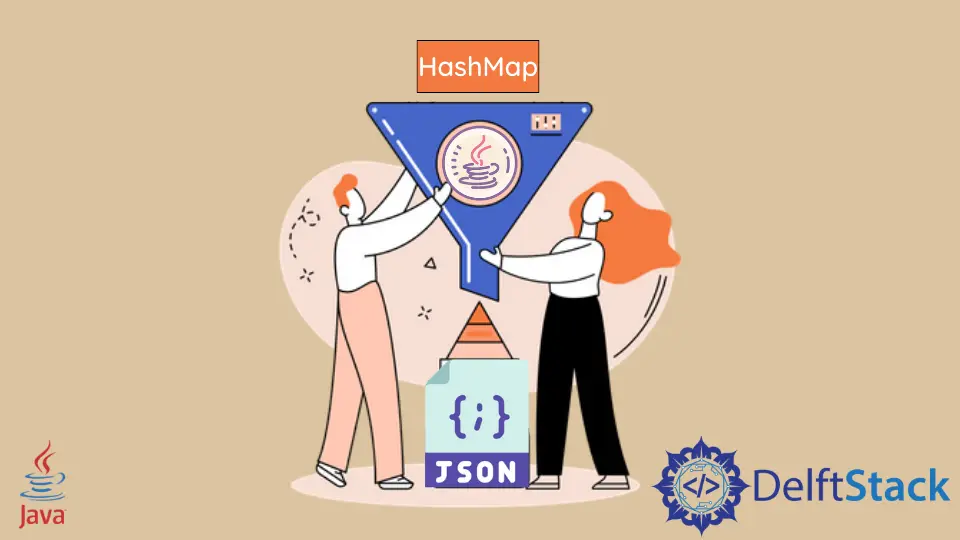
This article shows the ways to convert a hashmap to a JSON object in Java. We will see the examples in detail about creating a hashmap and then converting it into a JSON object.
Hashmap and JSON are both very commonly used by developers as they help us create a simple structure that can be used for storing as well as transferring data easily.
new JSONObject(hashmap)
to Convert Hashmap to JSON Object
The most traditional way of converting a hashmap to JSON object is by calling JSONObject()
and then passing the hashmap.
Let’s take a look at an example that creates a hashmap and then prints it in JSON format.
import java.util.HashMap;
import java.util.Map;
import org.json.simple.JSONObject;
public class Main {
public static void main(String[] args) {
Map<String, Object> map = new HashMap();
map.put("key1", "value1");
map.put("key2", "value2");
JSONObject json = new JSONObject(map);
System.out.printf("JSON: %s", json);
}
Output:
JSON: {"key1":"value1","key2":"value2"}
One thing to notice here is that Map<String, Object>
takes a string, which is the key
, and the Object, which is the value
. It means that we can pass any valid Object as a value into the map and then convert it as a JSON object.
Below is an example that takes a string and an Arraylist
as the value.
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Map;
import org.json.simple.JSONObject;
public class Main {
public static void main(String[] args) {
ArrayList<String> stringArrayList = new ArrayList<>();
stringArrayList.add("firstString");
stringArrayList.add("secondString");
stringArrayList.add("thirdString");
Map<String, Object> map = new HashMap();
map.put("key1", "value1");
map.put("key2", "value2");
map.put("stringList", stringArrayList);
JSONObject json = new JSONObject(map);
System.out.printf("JSON: %s", json);
}
Output:
JSON: {"key1":"value1","key2":"value2","stringList":["firstString","secondString","thirdString"]}
Jackson Library to Convert Hashmap Into a JSON Object
There are libraries in Java that can help us convert our hashmap into a JSON object with much flexibility.
Jackson is one of those libraries which takes a Java map
and then converts the map into JSON format.
We should not forget to handle the JsonProcessingException
as ObjectMapper().writeValueAsString(map)
can throw an exception when it finds an incompatible data format.
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.util.HashMap;
import java.util.Map;
public class Main {
public static void main(String[] args) throws JsonProcessingException {
Map<String, Object> map = new HashMap();
map.put("key1", "value1");
map.put("key2", "value2");
String json = new ObjectMapper().writeValueAsString(map);
System.out.printf("JSON: %s", json);
}
Output:
JSON: {"key1":"value1","key2":"value2"}
GSON Library to Convert the Hashmap Into JSON Object
Gson library is one of the most used libraries for converting a hashmap to a JSON object. It provides easy methods to work on our hashmap and JSON.
Gson
class has a method toJsonTree
which takes our map and converts it into a JSON tree. But as we need a JSON object, we can use toJSONObject()
to make the JSON tree a JSON Object.
import com.google.gson.Gson;
import com.google.gson.JsonObject;
import java.util.HashMap;
import java.util.Map;
public class Main {
public static void main(String[] args) {
Map<String, Object> map = new HashMap();
map.put("key1", "value1");
map.put("key2", "value2");
Gson gson = new Gson();
JsonObject json = gson.toJsonTree(map).getAsJsonObject();
System.out.printf("JSON: %s", json);
}
Output:
JSON: {"key1":"value1","key2":"value2"}
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedInRelated Article - Java JSON
- How to Pretty-Print JSON Data in Java
- How to Serialize Object to JSON in Java
- How to Convert JSON Data to String in Java
- How to Deserialize JSON in Java
- How to Handle JSON Arrays in Java
- How to Convert JSON to Java object