How to Get Key From Value in Java Hashmap
- Get a Single Key From a Value Using BidiMap in Java
-
Get a Single Key From a Value Using
map.entrySet()
in Java - Get Multiple Keys From Value Using the Stream API in Java
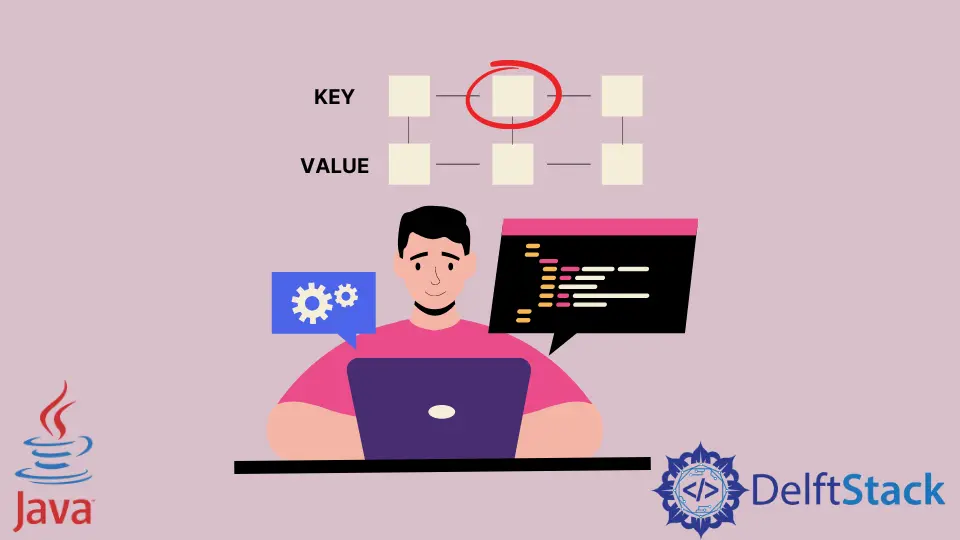
Hashmap is an essential part of Java and gives us the power of flexibly work on our data by using the key-value pair method. The value is attached to the key, and we can find the value using its key very easily. But what if we want to fetch the key from a value?
This is what we are going to discuss here. Our goal is to get the key that is attached to the value.
Get a Single Key From a Value Using BidiMap in Java
We can use a bidirectional map to get the key using its value. A bidirectional map ensures no duplicate values in the map and that the value can be used to get the key. BidiMap
is a bidirectional map that comes with the Apache Commons Collections.
The first and most important step is to include the dependency in our project.
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-collections4</artifactId>
<version>4.1</version>
</dependency>
The following example has a BidiMap
that contains four key-value pair elements. As a BidiMap
contains unique values, one key cannot have multiple values. It is why we can only get a single key from each value.
import org.apache.commons.collections4.BidiMap;
import org.apache.commons.collections4.bidimap.TreeBidiMap;
public class Main {
public static void main(String[] args) {
BidiMap<String, String> bidiMap = new TreeBidiMap<>();
bidiMap.put("key1", "value1");
bidiMap.put("key2", "value2");
bidiMap.put("key3", "value3");
bidiMap.put("key4", "value4");
System.out.println(bidiMap.getKey("value4"));
}
}
Output:
key4
Get a Single Key From a Value Using map.entrySet()
in Java
Map
in Java comes with a method entrySet()
that we can use to create a set of the map elements and then loop through it get our key with the value.
import java.util.*;
public class Main {
public static void main(String[] args) {
Map<String, Object> sampleMap = new HashMap<>();
sampleMap.put("key1", "value1");
sampleMap.put("key2", "value2");
sampleMap.put("key3", "value3");
sampleMap.put("key4", "value4");
String getKeyFromValue = getSingleKeyFromValue(sampleMap, "value2");
System.out.println(getKeyFromValue);
}
public static <K, V> K getSingleKeyFromValue(Map<K, V> map, V value) {
for (Map.Entry<K, V> entry : map.entrySet()) {
if (Objects.equals(value, entry.getValue())) {
return entry.getKey();
}
}
return null;
}
Output:
key2
Get Multiple Keys From Value Using the Stream API in Java
We saw how to get a single key from value, but a Map
can have multiple values attached to a single key. To get all the keys of a value, we will use the Stream API
of Java 8.
The below example uses the getMultipleKeysByValue()
method that takes the Map
and the value to find the keys. Then it returns the list of keys as a result.
import java.util.*;
import java.util.stream.Collectors;
public class Main {
public static void main(String[] args) {
Map<String, Object> sampleMap = new HashMap<>();
sampleMap.put("key1", "value1");
sampleMap.put("key2", "value1");
sampleMap.put("key3", "value2");
sampleMap.put("key4", "value1");
List<String> getKeysFromValue = getMultipleKeysByValue(sampleMap, "value1");
System.out.println(getKeysFromValue);
}
public static <String> List<String> getMultipleKeysByValue(
Map<String, Object> map, Object value) {
return map.entrySet()
.stream()
.filter(entry -> Objects.equals(entry.getValue(), value))
.map(Map.Entry::getKey)
.collect(Collectors.toList());
}
}
Output:
[key1, key2, key4]
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn