Converti Hashmap in oggetto JSON in Java
-
new JSONObject(hashmap)
per convertire Hashmap in oggetto JSON - Jackson Library per convertire l’hashmap in un oggetto JSON
- Libreria GSON per convertire l’hashmap in oggetto JSON
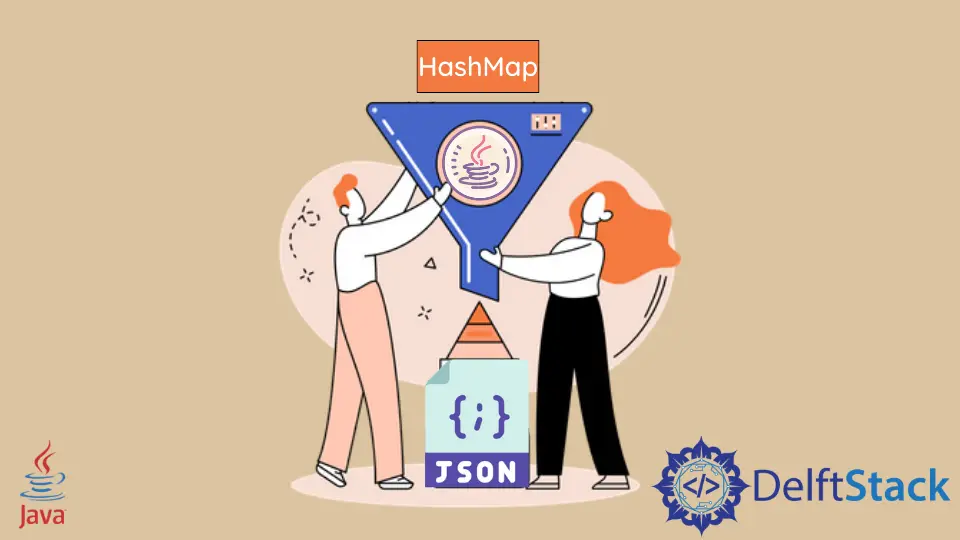
Questo articolo mostra i modi per convertire una hashmap in un oggetto JSON in Java. Vedremo in dettaglio gli esempi sulla creazione di una hashmap e quindi sulla sua conversione in un oggetto JSON.
Hashmap e JSON sono entrambi molto comunemente usati dagli sviluppatori in quanto ci aiutano a creare una struttura semplice che può essere utilizzata per archiviare e trasferire facilmente i dati.
new JSONObject(hashmap)
per convertire Hashmap in oggetto JSON
Il modo più tradizionale di convertire una hashmap in un oggetto JSON è chiamare JSONObject()
e quindi passare l’hashmap.
Diamo un’occhiata a un esempio che crea una hashmap e quindi la stampa in formato JSON.
import java.util.HashMap;
import java.util.Map;
import org.json.simple.JSONObject;
public class Main {
public static void main(String[] args) {
Map<String, Object> map = new HashMap();
map.put("key1", "value1");
map.put("key2", "value2");
JSONObject json = new JSONObject(map);
System.out.printf("JSON: %s", json);
}
Produzione:
JSON: {"key1":"value1","key2":"value2"}
Una cosa da notare qui è che Map<String, Object>
prende una stringa, che è la chiave
, e l’Oggetto, che è il valore
. Significa che possiamo passare qualsiasi oggetto valido come valore nella mappa e quindi convertirlo come oggetto JSON.
Di seguito è riportato un esempio che prende una stringa e un Arraylist
come valore.
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Map;
import org.json.simple.JSONObject;
public class Main {
public static void main(String[] args) {
ArrayList<String> stringArrayList = new ArrayList<>();
stringArrayList.add("firstString");
stringArrayList.add("secondString");
stringArrayList.add("thirdString");
Map<String, Object> map = new HashMap();
map.put("key1", "value1");
map.put("key2", "value2");
map.put("stringList", stringArrayList);
JSONObject json = new JSONObject(map);
System.out.printf("JSON: %s", json);
}
Produzione:
JSON: {"key1":"value1","key2":"value2","stringList":["firstString","secondString","thirdString"]}
Jackson Library per convertire l’hashmap in un oggetto JSON
Esistono librerie in Java che possono aiutarci a convertire la nostra hashmap in un oggetto JSON con molta flessibilità.
Jackson è una di quelle librerie che prende una map
Java e poi converte la mappa in formato JSON.
Non dobbiamo dimenticare di gestire JsonProcessingException
come ObjectMapper().writeValueAsString(map)
può lanciare un’eccezione quando trova un formato dati incompatibile.
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.util.HashMap;
import java.util.Map;
public class Main {
public static void main(String[] args) throws JsonProcessingException {
Map<String, Object> map = new HashMap();
map.put("key1", "value1");
map.put("key2", "value2");
String json = new ObjectMapper().writeValueAsString(map);
System.out.printf("JSON: %s", json);
}
Produzione:
JSON: {"key1":"value1","key2":"value2"}
Libreria GSON per convertire l’hashmap in oggetto JSON
La libreria Gson è una delle librerie più utilizzate per convertire una hashmap in un oggetto JSON. Fornisce metodi semplici per lavorare sulla nostra hashmap e JSON.
La classe Gson
ha un metodo toJsonTree
che prende la nostra mappa e la converte in un albero JSON. Ma poiché abbiamo bisogno di un oggetto JSON, possiamo usare toJSONObject()
per rendere l’albero JSON un oggetto JSON.
import com.google.gson.Gson;
import com.google.gson.JsonObject;
import java.util.HashMap;
import java.util.Map;
public class Main {
public static void main(String[] args) {
Map<String, Object> map = new HashMap();
map.put("key1", "value1");
map.put("key2", "value2");
Gson gson = new Gson();
JsonObject json = gson.toJsonTree(map).getAsJsonObject();
System.out.printf("JSON: %s", json);
}
Produzione:
JSON: {"key1":"value1","key2":"value2"}
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn