How to Convert File to a Byte Array in Java
Hassan Saeed
Feb 02, 2024
-
Use
readAllBytes()
to Convert a File to Byte Array in Java -
Use
FileUtils.readFileToByteArray()
to Convert a File to Byte Array in Java
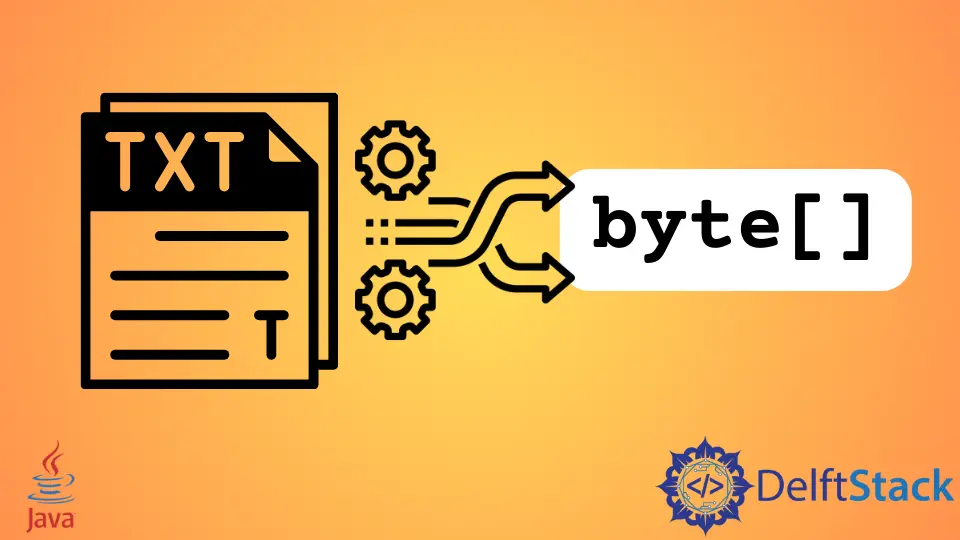
This tutorial discusses methods to convert a file to byte array in Java.
Use readAllBytes()
to Convert a File to Byte Array in Java
The simplest way to convert a file to byte array in Java is to use the readAllBytes()
method provided by the Files
class. In the example below, we will first read a file and then convert it to a byte array.
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Path;
public class MyClass {
public static void main(String args[]) throws IOException {
File file = new File('sample.txt') byte[] fileContent = Files.readAllBytes(file.toPath());
}
}
Use FileUtils.readFileToByteArray()
to Convert a File to Byte Array in Java
Another method to convert a file to byte array in Java is to use FileUtils.readFileToByteArray()
provided by Apache Commons File Utils. The below example illustrates this:
import org.apache.commons.io.FileUtils;
public class MyClass {
public static void main(String[] args) throws IOException {
File file = new File('sample.txt');
byte[] fileContent = FileUtils.readFileToByteArray(file);
}
}