How to Check Whether an Array Is Null/Empty in Java
- Null Array in Java
- Array Contains Null Values
- Empty Array in Java
- Check Array Null Using Apache Commons Library in Java
- Check Array Null Using Java 8
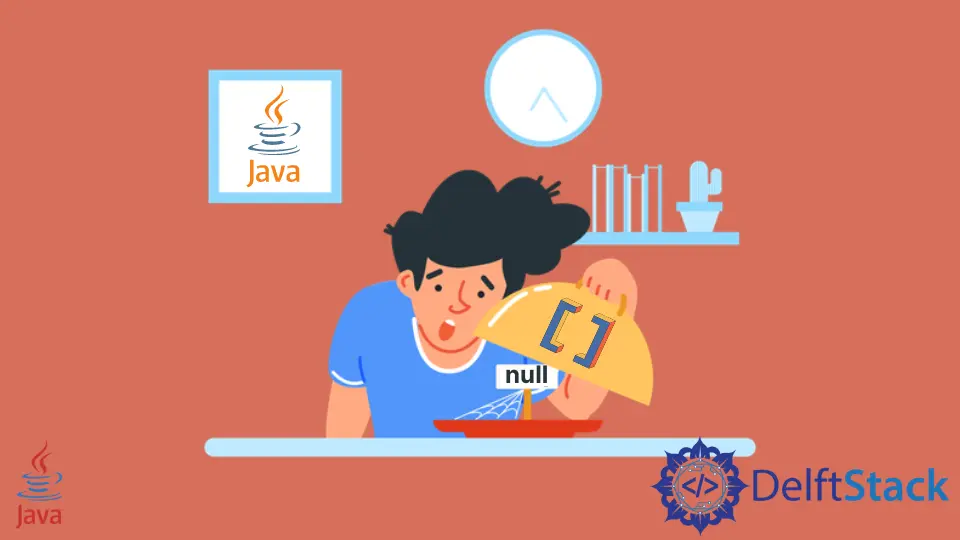
This tutorial introduces how to check whether an array is null or empty in Java and also lists some example codes to understand the null checking process.
Null Array in Java
In Java, an array is an object that holds similar types of data. It can be null only if it is not instantiated or points to a null reference.
In this example, we have created two arrays. The array arr
is declared but not instantiated. It does not hold any data and refers to a null reference (default value) assigned by the compiler. The array arr2
is declared and explicitly assigned to null to create a null array.
We can use this example to check whether the array is null or not.
public class SimpleTesting {
String[] arr;
String[] arr2 = null;
public static void main(String[] args) {
SimpleTesting obj = new SimpleTesting();
if (obj.arr == null) {
System.out.println("The array is null");
}
if (obj.arr2 == null) {
System.out.println("The array2 is null");
}
}
}
Output:
The array is null
The array2 is null
Array Contains Null Values
This is the second scenario where an array contains null values. In that case, we can consider an array to be null.
Suppose, we have an array of string that can contain 5 elements. Since the array is not initialized then it holds null (default value) assigned by the compiler.
public class SimpleTesting {
String[] arr = new String[5];
public static void main(String[] args) {
boolean containNull = true;
SimpleTesting obj = new SimpleTesting();
for (int i = 0; i < obj.arr.length; i++) {
if (obj.arr[i] != null) {
containNull = false;
break;
}
}
if (containNull) {
System.out.println("Array is null");
}
}
}
Output:
Array is null
Empty Array in Java
An array is empty only when it contains zero(0) elements and has zero length. We can test it by using the length property of the array object.
public class SimpleTesting {
String[] arr = new String[0];
public static void main(String[] args) {
SimpleTesting obj = new SimpleTesting();
if (obj.arr.length == 0) {
System.out.println("The array is Empty");
}
}
}
Output:
The array is Empty
Check Array Null Using Apache Commons Library in Java
If you are working with Apache
then use ArrayUtils
class to check whether an array is empty. The ArrayUtils
class provides a method isEmpty()
which returns a boolean value either true or false. For more info about apache library visit here.
import org.apache.commons.lang3.ArrayUtils;
public class SimpleTesting {
String[] arr = new String[0];
public static void main(String[] args) {
SimpleTesting obj = new SimpleTesting();
Boolean isEmpty = ArrayUtils.isEmpty(obj.arr);
if (isEmpty) {
System.out.println("Array is Empty");
}
}
}
Output:
Array is Empty
Check Array Null Using Java 8
If you are working with Java 8 or higher version then you can use the stream()
method of Arrays class to call the allMatch()
method to check whether array contains null values or not.
This is the case when array contains null values.
import java.util.Arrays;
import java.util.Objects;
public class SimpleTesting {
String[] arr = new String[10];
public static void main(String[] args) {
SimpleTesting obj = new SimpleTesting();
Boolean containNull = Arrays.stream(obj.arr).allMatch(Objects::nonNull);
if (!containNull) {
System.out.println("Array is null");
}
}
}
Output:
Array is null