How to Check Whether a String Contains a Substring in Java
- Check if a String Contains a Substring in Java
-
Find Substring Using the
indexOf()
Mehtod in Java - Find Substring Using Regex in Java
-
Find Substring Using
Apache
in Java - Find Substring Using Java Regex
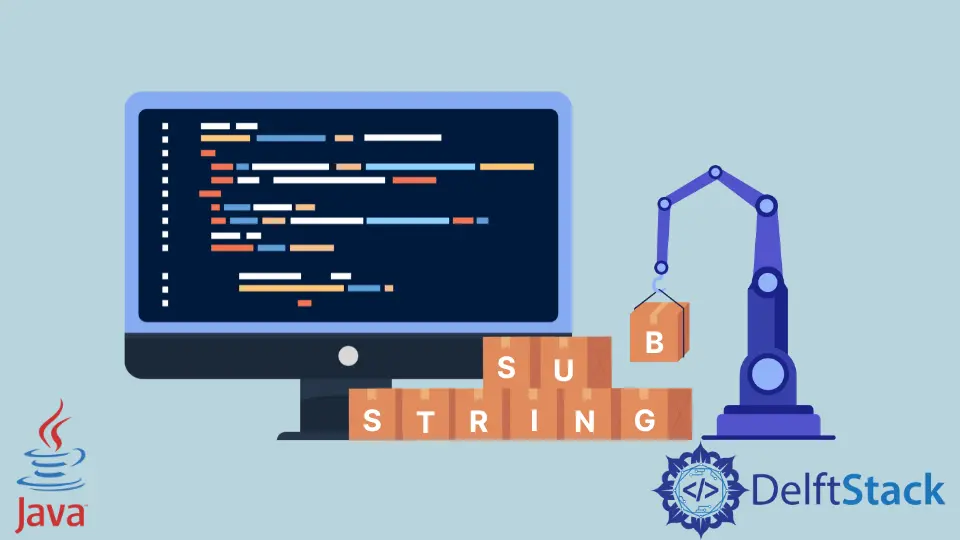
This tutorial introduces how to check whether a string contains a substring in Java and lists some example codes to understand it.
Check if a String Contains a Substring in Java
A String that is a part of another String is known as substring for that String
object. A string is a sequence of characters and an immutable object in Java. Let’s check if a substring exists in the given string.
In this example, we use the contains()
method of the String
class that returns true
if the string’s substring is found. We use the toLowerCase()
method to check for ignore case.
public class SimpleTesting {
public static void main(String[] args) {
String str = "abracadaabra";
String subStr = "daa";
boolean containsStr = str.contains(subStr);
System.out.println(containsStr);
// Check with string ignore case
subStr = "Daa";
containsStr = str.toLowerCase().contains(subStr.toLowerCase());
System.out.println(containsStr);
subStr = "baa";
containsStr = str.toLowerCase().contains(subStr.toLowerCase());
System.out.println(containsStr);
}
}
Output:
true
true
false
Find Substring Using the indexOf()
Mehtod in Java
The indexOf()
method of the String
class is used to get the index of the substring in a string but we can use it to find the substring.
Here, we check if the substring index is greater than or equal zero than it means substring is found. If the substring doesn’t exist in the string, it returns -1
.
See the example and output.
public class SimpleTesting {
public static void main(String[] args) {
String str = "abracadaabra";
String subStr = "daa";
boolean containsStr = str.indexOf(subStr)>=0;
System.out.println(containsStr);
// check with string ignore case
subStr = "DaA";
containsStr = str.toLowerCase().indexOf(subStr.toLowerCase())>=0;
System.out.println(containsStr);
subStr = "aaa";
containsStr = str.indexOf(subStr)>=0;
System.out.println(containsStr);
}
}
Output:
true
true
false
Find Substring Using Regex in Java
We can also use the matches()
method of the String
object that takes regex as the argument and returns either true
or false
.
public class SimpleTesting {
public static void main(String[] args) {
String str = "abracadaabra";
String subStr = "daa";
boolean containsStr = str.matches("(?i).*" + subStr + ".*");
System.out.println(containsStr);
subStr = "daa";
containsStr = str.matches("(?i).*" + subStr + ".*");
System.out.println(containsStr);
}
}
Output:
true
true
Find Substring Using Apache
in Java
If you use Apache
commons library, then you can use the containsIgnoreCase()
method of the StringUtils
class that returns true
if the substring is found, false
otherwise. See the example below.
import org.apache.commons.lang3.StringUtils;
public class SimpleTesting {
public static void main(String[] args) {
String str = "abracadaabra";
String subStr = "daa";
boolean containsStr = StringUtils.containsIgnoreCase(str, subStr);
System.out.println(containsStr);
subStr = "DAa";
containsStr = StringUtils.containsIgnoreCase(str, subStr);
System.out.println(containsStr);
subStr = "AcWF";
containsStr = StringUtils.containsIgnoreCase(str, subStr);
System.out.println(containsStr);
}
}
Output:
true
true
false
Find Substring Using Java Regex
Java provides a regex
package that contains several classes and methods. One of these class is a Pattern
class that provides couple of methods like compile()
, quote()
, matcher()
, etc. Here, we will use these methods to find substring.
import java.util.regex.Pattern;
public class SimpleTesting {
public static void main(String[] args) {
String str = "abracadaabra";
String subStr = "daa";
boolean containsStr =
Pattern.compile(Pattern.quote(subStr), Pattern.CASE_INSENSITIVE).matcher(str).find();
System.out.println(containsStr);
subStr = "RaCA";
containsStr =
Pattern.compile(Pattern.quote(subStr), Pattern.CASE_INSENSITIVE).matcher(str).find();
System.out.println(containsStr);
}
}
Output:
true
true