How to Add New Elements to an Array in Java
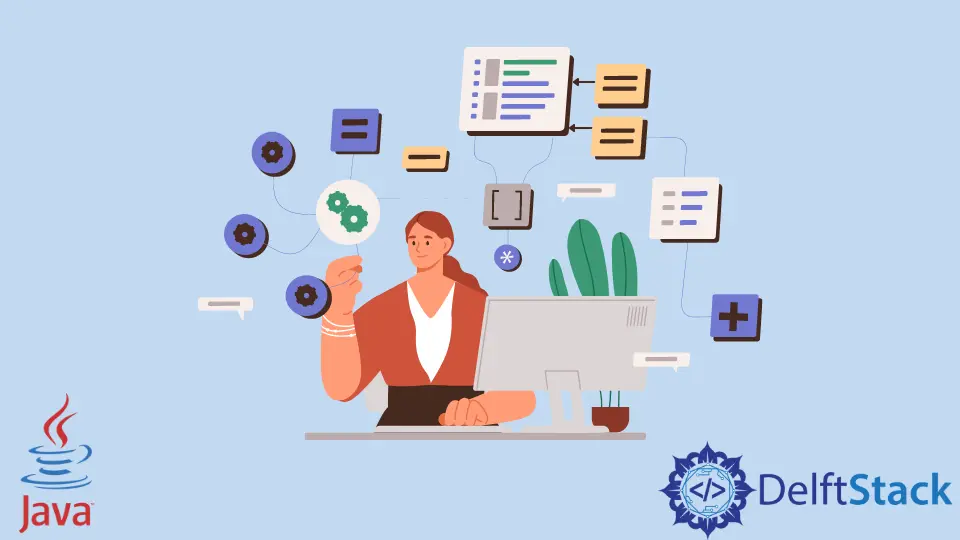
This tutorial discusses how to add new elements to an array in Java.
Array
in Java is a container object which holds a fixed number of elements of the same data type. The length of the array is defined while declaring the array object, and can not be changed later on.
Suppose we have an array of length 5 in Java instantiated with some values:
String[] arr = new String[5];
arr[0] = "1";
arr[1] = "2";
arr[2] = "3";
arr[3] = "4";
arr[4] = "5";
Now there is a requirement to add a 6th element to our array. Let’s try to add this 6th element to our array.
arr[5] = "6";
The above line of code gives the following error:
Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: Index 5 out of bounds for length 5
This is because we declared the array to be of size 5 initially and we tried to add a 6th element to it.
Not to worry, there are 2 possible solutions to get this done. We can use an ArrayList
instead of an array or create a new larger array to accommodate new elements.
Use an ArrayList
A better and recommended solution is to use an ArrayList
instead of an array since it is resizable. There is no fixed size of ArrayList
therefore whenever there is a need to add a new element you can simply add by executing testList.add(element)
.
import java.util.*;
public class Main {
public static void main(String args[]) {
List<String> testList = new ArrayList<String>();
testList.add("1");
testList.add("2");
testList.add("3");
testList.add("4");
testList.add("5");
// Print the original list
System.out.println("Initial ArrayList:\n" + testList);
// Add elements without running into any error
testList.add("6");
testList.add("7");
// Print the list after adding elements
System.out.println("Modified ArrayList:\n" + testList);
}
}
The above code outputs the following.
Initial ArrayList : [ 1, 2, 3, 4, 5 ] Modified ArrayList : [ 1, 2, 3, 4, 5, 6, 7 ]
Or if we already have an array, we can also create an ArrayList
directly.
import java.util.*;
public class Main {
public static void main(String args[]) {
// Create an array
String[] arr = new String[1];
arr[0] = "1";
// Convert to ArrayList
List<String> testList = new ArrayList<>(Arrays.asList(arr));
// Print the original list
System.out.println("Initial ArrayList:\n" + testList);
// Add elements to it
testList.add("2");
testList.add("3");
// Print the list after adding elements
System.out.println("Modified ArrayList:\n" + testList);
}
}
The above code outputs the following.
Initial ArrayList : [ 1 ] Modified ArrayList : [ 1, 2, 3 ]
We can easily convert an ArrayList
back to an array.
import java.util.*;
public class Main {
public static void main(String args[]) {
// Create an array
String[] arr = new String[1];
arr[0] = "1";
// Convert to ArrayList
List<String> testList = new ArrayList<>(Arrays.asList(arr));
// Add elements to it
testList.add("2");
testList.add("3");
// Convert the arraylist back to an array
arr = new String[testList.size()];
testList.toArray(arr);
}
}
Create a New Larger Array
If we are insistent on working with arrays only, we can use the java.util.Arrays.copyOf
method to create a bigger array and accommodate a new element. Let us use the array arr
we created above and add a new element to it in the example below.
import java.util.*;
public class Main {
public static void main(String args[]) {
// Create an array
String[] arr = new String[5];
arr[0] = "1";
arr[1] = "2";
arr[2] = "3";
arr[3] = "4";
arr[4] = "5";
// print the original array
System.out.println("Initial Array:\n" + Arrays.toString(arr));
// Steps to add a new element
// Get the current length of the array
int N = arr.length;
// Create a new array of length N+1 and copy all the previous elements to this new array
arr = Arrays.copyOf(arr, N + 1);
// Add a new element to the array
arr[N] = "6";
// print the updated array
System.out.println("Modified Array:\n" + Arrays.toString(arr));
}
}
The above code outputs the following.
Initial Array : [ 1, 2, 3, 4, 5 ] Modified Array : [ 1, 2, 3, 4, 5, 6 ]
If later we feel the need to add another element to arr
, we will have to repeat the above block of code again!
Therefore this solution is not recommended because the addition of every new element has a time complexity of O(n)
since it has to copy all the elements from the previous array to a new array. On the other hand, the addition of every new element using ArrayList
has O(1)
amortized cost per operation.