How to Create A Generic Array in Java
- Use Object Arrays to Create Generic Arrays in Java
- Use the Reflection Class to Create Generic Arrays in Java
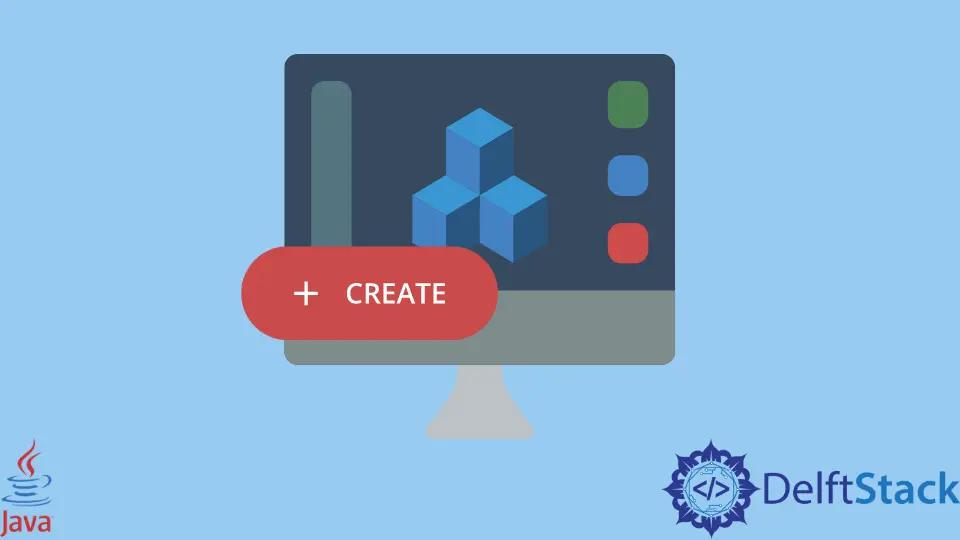
An array can be defined as a collection of items stored at contiguous memory locations. A generic array is independent of any data type and whose type of information is evaluated at runtime.
However, Java doesn’t allow the array to be generic because, in Java, arrays contain information associated with their components, and this information at the runtime is used to allocate memory.
We can simulate generic structures that are array-like using objects array and reflection class feature in Java. We will discuss these methods below.
Use Object Arrays to Create Generic Arrays in Java
An array of type objects as a member is used in this approach. We use the get()
and set()
functions to read and set the array elements.
The following program demonstrates the use of an object array to create a generic array.
import java.util.Arrays;
class Generic_Array<E> {
private final Object[] obj_array; // object array
public final int length;
// class constructor
public Generic_Array(int len) {
// new object array
obj_array = new Object[len];
this.len = len;
}
// get new object array(obj_array[i])
E get(int j) {
@SuppressWarnings("unchecked") final E e = (E) object_array[j];
return e;
}
// set e at new object array(obj_array[i])
void set(int j, E e) {
object_array[j] = e;
}
@Override
public String toString() {
return Arrays.toString(object_array);
}
}
class Main {
public static void main(String[] args) {
final int len = 5;
// creating an integer array
Generic_Array<Integer> int_Array = new Generic_Array(len);
System.out.print("Generic Array <Integer>:"
+ " ");
for (int i = 2; i < len; i++) int_Array.set(i, i * 2);
System.out.println(int_Array);
// creating a string array
Generic_Array<String> str_Array = new Generic_Array(len);
System.out.print("Generic Array <String>:"
+ " ");
for (int i = 0; i < len; i++) str_Array.set(i, String.valueOf((char) (i + 97)));
System.out.println(str_Array);
}
}
Output:
Generic Array <Integer>: [2, 4, 6, 8, 10]
Generic Array <String>: [a, b, c, d, e]
Use the Reflection Class to Create Generic Arrays in Java
In this type of approach, a reflection class is used to create a generic array whose type will be known at the runtime only.
The only difference between the previous approach and this approach is that the reflection class is used as the constructor itself. After that, the reflection class initiates an object array by explicitly passing the data to the constructor class.
The following program demonstrates the use of reflection to create a generic array.
import java.util.Arrays;
class Generic_Array<E> {
private final E[] objArray;
public final int length;
// constructor class
public Generic_Array(Class<E> dataType, int length) {
// creatting a new array with the specified data type and length at runtime using reflection
// method.
this.objArray = (E[]) java.lang.reflect.Array.newInstance(dataType, len);
this.len = len;
}
// get element at obj_Array[i]
E get(int i) {
return obj_Array[i];
}
// assign e to obj_Array[i]
void set(int i, E e) {
obj_Array[i] = e;
}
@Override
public String toString() {
return Arrays.toString(obj_Array);
}
}
class Main {
public static void main(String[] args) {
final int len = 5;
// create array with Integer as data type
Generic_Array<Integer> int_Array = new Generic_Array(Integer.class, len);
System.out.print("Generic Array<Int>:"
+ " ");
for (int i = 2; i < len; i++) int_Array.set(i, i + 10);
System.out.println(int_Array);
// create an array with String as data type
Generic_Array<String> str_Array = new Generic_Array(String.class, len);
System.out.print("Generic Array<Str>:"
+ " ");
for (int i = 0; i < len; i++) str_Array.set(i, String.valueOf((char) (i + 65)));
System.out.println(str_Array);
}
}
Output:
Generic Array<Int>: [12, 13, 14, 15, 16]
Generic Array<Str>: [A, B, C, D, E]
Generic array can’t provide type safety unless a type checking or explicit mechanism is implemented as generic classes are unknown to that type of argument they created at runtime.
If we want to have a precise array without any safety discussed above using generics, it can be done as shown below.
import java.lang.reflect.Array;
public class Gen_Set<E> {
private E[] x;
public Gen_Set(Class<E[]> cla, int len) {
x = cla.cast(Array.newInstance(cla.getComponentType(), len));
}
public static void main(String[] args) {
Gen_Set<String> foo = new Gen_Set<String>(String[].class, 1);
String[] bar = foo.x;
foo.x[0] = "xyzzy";
String baz = foo.a[0];
}
}
This code will give no warnings at the compile-time, and we can see that in the main class, the type of instance of Gen_Set
declared can be assigned the same to the x to an array of that type, meaning both array and the values of the array are incorrect types.