Method to Calculate Factorial in Java
- Get Factorial Using the Iterative Method in Java
- Find Factorial Using the Recursive Method in Java
- Find Factorial Using the Dynamic Approach in Java
- Find Factorial Using Apache Commons in Java
- Find Factorial Using Java 8 Streams
-
Find Factorial Using
BigInteger
in Java -
Find Factorial Using
BigIntegerMath
Library
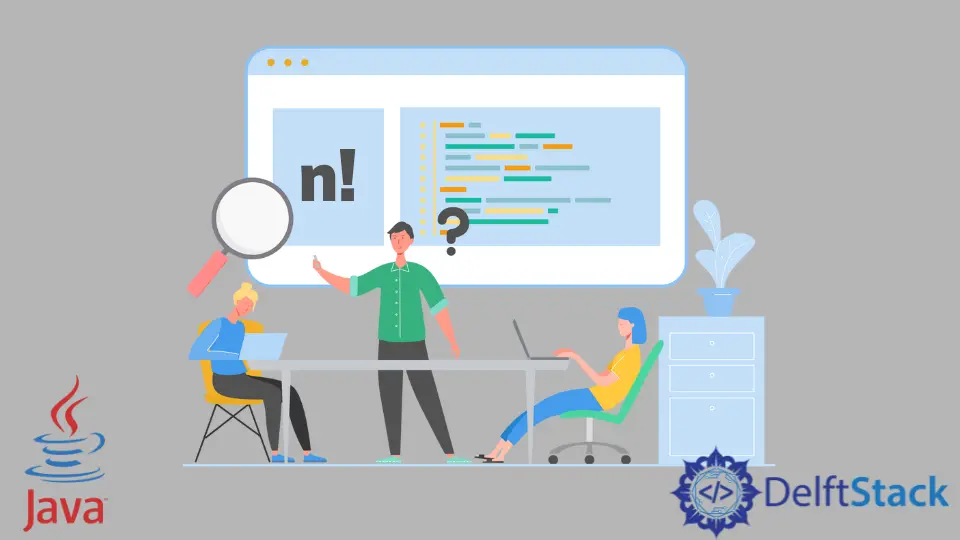
This tutorial introduces the methods and code examples to calculate factorial in Java.
The factorial of a number n
is the multiplication of all the natural numbers between 1
and n
. In this tutorial, we will see different ways of calculating the factorial of a number.
We will first look at how the factorial of numbers below and equal to 20
can be calculated. This segregation is because of Java’s limited range of long data types.
The factorials of numbers above 20
are too big to fit in the longs’ range.
Get Factorial Using the Iterative Method in Java
In this example, we created a variable, store_fact
, of a long type and initialized it with 1
.
We then loop through all the integers from 1
to the number whose factorial is calculated and multiply the loop variable value with the store_fact
value. We stored the calculated value into the store_fact
variable and updated the loop variable.
To make the above algorithm more clear, we can write it like this:
- initialize
n
- initialize
store_fact = 1
- do
for i = 1
ton
store_fact = store_fact*n
- increment
i
- return
store_fact
In the algorithm above, the store_fact
variable stores the factorial of the n
as follows:
- After the first iteration:
store_value = 1 = 1!
- After the second iteration:
store_value = 1 X 2 = 2!
- After the third iteration:
store_value = 1 X 2 X 3 = 3!
- After the nth iteration:
store_value = 1 X 2 X 3 X 4 ........ Xn = n!
Let us now look at the code example for the above algorithm.
import java.util.Scanner;
public class SimpleTesting {
static long factCalculator(int n) {
long store_fact = 1;
int i = 1;
while (i <= n) {
store_fact = store_fact * i;
i++;
}
return store_fact;
}
public static void main(String args[]) {
int number;
Scanner scan = new Scanner(System.in);
System.out.println("Enter a number: ");
number = scan.nextInt();
System.out.println(factCalculator(number));
}
}
Output:
Enter a number:
4
24
Find Factorial Using the Recursive Method in Java
The above iterative method can be transformed into a recursive method to find factorial of any number. In this method, we take the base case as:
if (n == 0 || n == 1) {
return 1;
}
If the base condition is not fulfilled, it returns:
n* factCalculator(n - 1);
Let’s see the code example below. We used a recursive method, factCalculator()
, to find factorial.
import java.util.*;
public class SimpleTesting {
static long factCalculator(int n) {
if (n == 0 || n == 1) {
return 1;
} else {
return n * factCalculator(n - 1);
}
}
public static void main(String args[]) {
int number;
Scanner scan = new Scanner(System.in);
System.out.println("Enter a number: ");
number = scan.nextInt();
System.out.println(factCalculator(number));
}
}
Output:
Enter a number:
4
24
Find Factorial Using the Dynamic Approach in Java
We can also calculate the factorial of a number by using the dynamic programming approach. This method is faster than other methods as it stores the factorial of smaller numbers and calculates the factorials of larger numbers using those factorials.
For example:
- 5! = 5 X 4!
- 4! = 4 X 3!
- 3! = 3 X 2!
- 2! = 2 X 1!
- 1! = 1 X 0!
- 0! = 1
In this method, we create a lookup table. This table stores the factorials of numbers from 0
to 20
.
We created the lookup table till 20
only because it is the largest number whose factorial long can store. We initialized 0!
as 1
.
We then used the value 0!
to calculate 1!
, the value of 1!
to calculate 2!
and so on. Look at the code below:
import java.util.*;
public class SimpleTesting {
static long[] factCalculator() {
long[] fact_table = new long[21];
fact_table[0] = 1;
for (int i = 1; i < fact_table.length; i++) {
fact_table[i] = fact_table[i - 1] * i;
}
return fact_table;
}
public static void main(String args[]) {
long[] table = factCalculator();
int number;
Scanner scan = new Scanner(System.in);
System.out.println("Enter a number: ");
number = scan.nextInt();
System.out.println(table[number]);
}
}
Output:
Enter a number:
5
120
Find Factorial Using Apache Commons in Java
If you work with the Apache Commons Math library, use the CombinatoricsUtils
class with a factorial()
method. It is a built-in method to calculate the factorial of any number.
The value returned by this method is of long type; hence, we cannot calculate factorial of numbers greater than 20
. See the example below.
import java.util.Scanner;
import org.apache.commons.math3.util.CombinatoricsUtils;
public class SimpleTesting {
static long factCalculator(int n) {
return CombinatoricsUtils.factorial(n);
}
public static void main(String args[]) {
int number;
Scanner scan = new Scanner(System.in);
System.out.println("Enter a number: ");
number = scan.nextInt();
System.out.println(factCalculator(number));
}
}
Output:
Enter a number:
5
120
Find Factorial Using Java 8 Streams
We can also use Java 8 stream API to calculate the factorial of a number. We will first create a stream of numbers from 1
to n
, where n
is the number whose factorial is calculated.
We then use the reduce method to perform the reduction operation on the elements. We passed 1
as the identity element and multiplication as the associative accumulation function.
Look at the code below:
import java.util.*;
import java.util.stream.LongStream;
public class SimpleTesting {
static long factCalculator(int n) {
return LongStream.rangeClosed(1, n).reduce(1, (long num1, long num2) -> num1 * num2);
}
public static void main(String args[]) {
int number;
Scanner scan = new Scanner(System.in);
System.out.println("Enter a number: ");
number = scan.nextInt();
System.out.println(factCalculator(number));
}
}
Output:
Enter a number:
5
120
There is a great advantage of using the reduce()
function over the iterative or recursive method. The reduce()
operation is parallelizable if the function used to process the elements is associative.
Now, we will calculate the factorial of numbers above 20
.
Find Factorial Using BigInteger
in Java
The BigInteger
class is used to handle very large numbers beyond the range of primitive data types. We can use BigInteger
to store the value of factorials of numbers above 20
.
See the example below.
import java.math.BigInteger;
import java.util.Scanner;
public class SimpleTesting {
static BigInteger factCalculator(int n) {
BigInteger store_fact = BigInteger.ONE;
for (int i1 = 2; i1 <= n; i1++) {
store_fact = store_fact.multiply(BigInteger.valueOf(i1));
}
return store_fact;
}
public static void main(String args[]) {
int number;
Scanner scan = new Scanner(System.in);
System.out.println("Enter a number: ");
number = scan.nextInt();
System.out.println(factCalculator(number));
scan.close();
}
}
Output:
Enter a number:
50
30414093201713378043612608166064768844377641568960512000000000000
Since we cannot multiply BigInteger
using the *
operator, we use the multiply()
function. This method is just like the iterative method, except that we use BigInteger
instead of long.
Find Factorial Using BigIntegerMath
Library
The BigIntegerMath
library has a built-in factorial()
method, which can be used to calculate the factorial of a number. It is a static method and returns a long-type value.
See the example below.
import com.google.common.math.BigIntegerMath;
import java.util.*;
public class SimpleTesting {
static long factCalculator(int n) {
return BigIntegerMath.factorial(n);
}
public static void main(String args[]) {
int number;
Scanner scan = new Scanner(System.in);
System.out.println("Enter a number: ");
number = scan.nextInt();
System.out.println(factCalculator(number));
}
}
Output:
Enter a number:
50
30414093201713378043612608166064768844377641568960512000000000000