Java 中計算階乘的方法
- 使用 Java 中的迭代方法獲取階乘
- 在 Java 中使用遞迴方法查詢階乘
- 在 Java 中使用動態方法查詢階乘
- 在 Java 中使用 Apache Commons 查詢階乘
- 使用 Java 8 流查詢階乘
-
在 Java 中使用
BigInteger
查詢階乘 -
使用
BigIntegerMath
庫查詢階乘
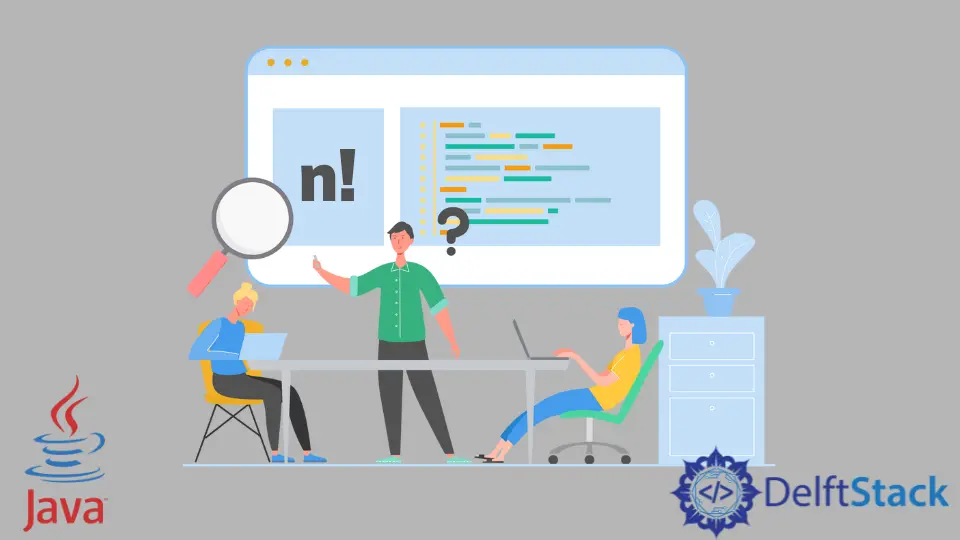
本教程介紹了在 Java 中計算階乘的方法和程式碼示例。
數 n
的階乘是 1
和 n
之間所有自然數的乘積。在本教程中,我們將看到計算數字階乘的不同方法。
我們將首先看看如何計算小於等於 20
的數字的階乘。這種隔離是因為 Java 的長資料型別範圍有限。
20
以上數字的階乘太大而無法放入多頭的範圍內。
使用 Java 中的迭代方法獲取階乘
在這個例子中,我們建立了一個 long 型別的變數 store_fact
,並使用 1
對其進行初始化。
然後我們遍歷從 1
到計算階乘的所有整數,並將迴圈變數值乘以 store_fact
值。我們將計算值儲存到 store_fact
變數中並更新迴圈變數。
為了讓上面的演算法更清晰,我們可以這樣寫:
- 初始化
n
- 初始化
store_fact = 1
- 執行
for i = 1
到n
store_fact = store_fact*n
- 增加
i
- 返回
store_fact
在上面的演算法中,store_fact
變數儲存 n
的階乘如下:
- 第一次迭代後:
store_value = 1 = 1!
- 第二次迭代後:
store_value = 1 X 2 = 2!
- 第三次迭代後:
store_value = 1 X 2 X 3 = 3!
- 第 n 次迭代後:
store_value = 1 X 2 X 3 X 4 ........ Xn = n!
現在讓我們看一下上述演算法的程式碼示例。
import java.util.Scanner;
public class SimpleTesting {
static long factCalculator(int n) {
long store_fact = 1;
int i = 1;
while (i <= n) {
store_fact = store_fact * i;
i++;
}
return store_fact;
}
public static void main(String args[]) {
int number;
Scanner scan = new Scanner(System.in);
System.out.println("Enter a number: ");
number = scan.nextInt();
System.out.println(factCalculator(number));
}
}
輸出:
Enter a number:
4
24
在 Java 中使用遞迴方法查詢階乘
上面的迭代方法可以轉化為遞迴方法來求任意數的階乘。在這種方法中,我們將基本情況視為:
if (n == 0 || n == 1) {
return 1;
}
如果不滿足基本條件,則返回:
n* factCalculator(n - 1);
讓我們看看下面的程式碼示例。我們使用遞迴方法 factCalculator()
來查詢階乘。
import java.util.*;
public class SimpleTesting {
static long factCalculator(int n) {
if (n == 0 || n == 1) {
return 1;
} else {
return n * factCalculator(n - 1);
}
}
public static void main(String args[]) {
int number;
Scanner scan = new Scanner(System.in);
System.out.println("Enter a number: ");
number = scan.nextInt();
System.out.println(factCalculator(number));
}
}
輸出:
Enter a number:
4
24
在 Java 中使用動態方法查詢階乘
我們還可以使用動態規劃方法計算數字的階乘。此方法比其他方法更快,因為它儲存較小數字的階乘並使用這些階乘計算較大數字的階乘。
例如:
- 5! = 5 X 4!
- 4! = 4 X 3!
- 3! = 3 X 2!
- 2! = 2 X 1!
- 1! = 1 X 0!
- 0! = 1
在這個方法中,我們建立了一個查詢表。該表儲存從 0
到 20
的數字的階乘。
我們建立直到 20
的查詢表只是因為它是階乘 long 可以儲存的最大數。我們初始化了 0!
作為 1
。
然後我們使用值 0!
計算 1!
,1!
的值計算 2!
等等。看下面的程式碼:
import java.util.*;
public class SimpleTesting {
static long[] factCalculator() {
long[] fact_table = new long[21];
fact_table[0] = 1;
for (int i = 1; i < fact_table.length; i++) {
fact_table[i] = fact_table[i - 1] * i;
}
return fact_table;
}
public static void main(String args[]) {
long[] table = factCalculator();
int number;
Scanner scan = new Scanner(System.in);
System.out.println("Enter a number: ");
number = scan.nextInt();
System.out.println(table[number]);
}
}
輸出:
Enter a number:
5
120
在 Java 中使用 Apache Commons 查詢階乘
如果你使用 Apache Commons Math 庫,請使用帶有 factorial()
方法的 CombinatoricsUtils
類。它是計算任何數字的階乘的內建方法。
該方法返回的值為 long 型別;因此,我們無法計算大於 20
的數字的階乘。請參見下面的示例。
import java.util.Scanner;
import org.apache.commons.math3.util.CombinatoricsUtils;
public class SimpleTesting {
static long factCalculator(int n) {
return CombinatoricsUtils.factorial(n);
}
public static void main(String args[]) {
int number;
Scanner scan = new Scanner(System.in);
System.out.println("Enter a number: ");
number = scan.nextInt();
System.out.println(factCalculator(number));
}
}
輸出:
Enter a number:
5
120
使用 Java 8 流查詢階乘
我們還可以使用 Java 8 流 API 來計算數字的階乘。我們將首先建立一個從 1
到 n
的數字流,其中 n
是計算其階乘的數字。
然後我們使用 reduce 方法對元素執行歸約操作。我們將 1
作為單位元素,將乘法作為關聯累加函式。
看下面的程式碼:
import java.util.*;
import java.util.stream.LongStream;
public class SimpleTesting {
static long factCalculator(int n) {
return LongStream.rangeClosed(1, n).reduce(1, (long num1, long num2) -> num1 * num2);
}
public static void main(String args[]) {
int number;
Scanner scan = new Scanner(System.in);
System.out.println("Enter a number: ");
number = scan.nextInt();
System.out.println(factCalculator(number));
}
}
輸出:
Enter a number:
5
120
與迭代或遞迴方法相比,使用 reduce()
函式有一個很大的優勢。如果用於處理元素的函式是關聯的,則 reduce()
操作是可並行化的。
現在,我們將計算 20
以上數字的階乘。
在 Java 中使用 BigInteger
查詢階乘
BigInteger
類用於處理超出原始資料型別範圍的非常大的數字。我們可以使用 BigInteger
來儲存 20
以上數字的階乘值。
請參見下面的示例。
import java.math.BigInteger;
import java.util.Scanner;
public class SimpleTesting {
static BigInteger factCalculator(int n) {
BigInteger store_fact = BigInteger.ONE;
for (int i1 = 2; i1 <= n; i1++) {
store_fact = store_fact.multiply(BigInteger.valueOf(i1));
}
return store_fact;
}
public static void main(String args[]) {
int number;
Scanner scan = new Scanner(System.in);
System.out.println("Enter a number: ");
number = scan.nextInt();
System.out.println(factCalculator(number));
scan.close();
}
}
輸出:
Enter a number:
50
30414093201713378043612608166064768844377641568960512000000000000
由於我們不能使用*
運算子來乘以 BigInteger
,所以我們使用 multiply()
函式。這個方法和迭代方法一樣,只是我們使用 BigInteger
而不是 long。
使用 BigIntegerMath
庫查詢階乘
BigIntegerMath
庫有一個內建的 factorial()
方法,可用於計算數字的階乘。它是一個靜態方法並返回一個 long 型別的值。
請參見下面的示例。
import com.google.common.math.BigIntegerMath;
import java.util.*;
public class SimpleTesting {
static long factCalculator(int n) {
return BigIntegerMath.factorial(n);
}
public static void main(String args[]) {
int number;
Scanner scan = new Scanner(System.in);
System.out.println("Enter a number: ");
number = scan.nextInt();
System.out.println(factCalculator(number));
}
}
輸出:
Enter a number:
50
30414093201713378043612608166064768844377641568960512000000000000