How to Fill a 2D Array in Java
- Understanding 2D Arrays in Java
- Method 1: Filling a 2D Array Using Nested Loops
- Method 2: Filling a 2D Array Using Arrays.fill()
- Method 3: Filling a 2D Array Using Stream API
- Conclusion
- FAQ
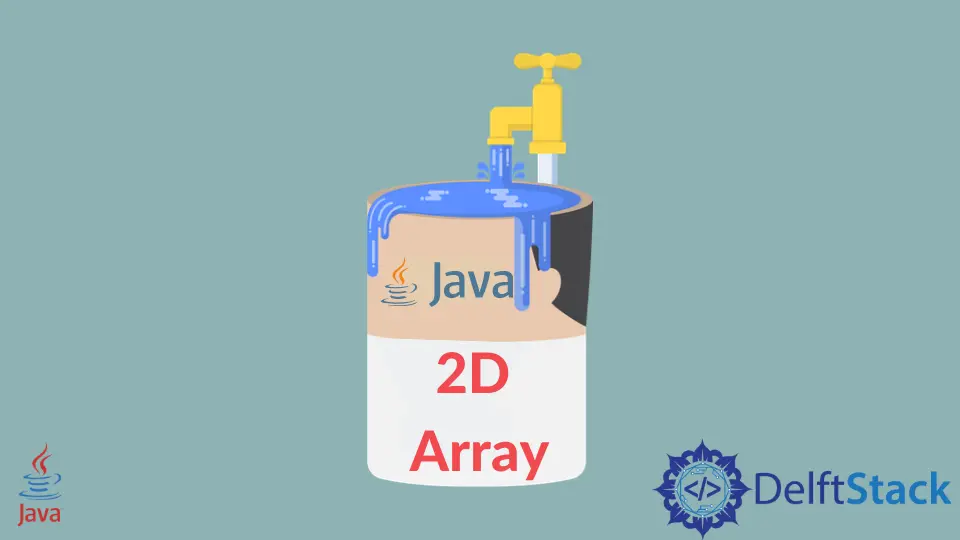
Filling a 2D array in Java can seem daunting at first, especially if you’re new to programming or coming from a different language. However, with a little guidance, you’ll find that it’s quite manageable. A 2D array is essentially an array of arrays, allowing you to store data in a tabular format.
This tutorial will walk you through the various methods to fill a 2D array in Java, covering everything from basic initialization to more complex scenarios. By the end of this article, you’ll have a solid understanding of how to create and manipulate 2D arrays effectively in Java.
Understanding 2D Arrays in Java
Before we dive into filling a 2D array, let’s clarify what a 2D array is. In Java, a 2D array is an array of arrays, which means each element of the main array is itself an array. This structure is useful for representing matrices, grids, or any data that can be organized in rows and columns. You can think of it as a table where each cell can hold a value.
To declare a 2D array in Java, you can use the following syntax:
dataType[][] arrayName = new dataType[rows][columns];
Here, dataType
can be any valid data type, such as int
, String
, or double
, and rows
and columns
specify the dimensions of the array.
Method 1: Filling a 2D Array Using Nested Loops
One of the most straightforward ways to fill a 2D array in Java is by using nested loops. This method allows you to iterate through each row and column, assigning values as you go. Below is an example of how to do this:
public class Fill2DArray {
public static void main(String[] args) {
int[][] array = new int[3][3];
for (int i = 0; i < array.length; i++) {
for (int j = 0; j < array[i].length; j++) {
array[i][j] = i + j; // Filling with the sum of indices
}
}
for (int[] row : array) {
for (int value : row) {
System.out.print(value + " ");
}
System.out.println();
}
}
}
Output:
0 1 2
1 2 3
2 3 4
In this example, we first declare a 3x3 integer array. Using nested loops, we fill each element with the sum of its row and column indices. After populating the array, we use another set of loops to print the values. This method is efficient and easy to understand, making it a popular choice among Java developers.
Method 2: Filling a 2D Array Using Arrays.fill()
Java provides a built-in method called Arrays.fill()
that can be used to fill an entire array or a specific range of an array. This method can also be applied to 2D arrays, but you will need to use it in conjunction with a loop. Here’s how you can do it:
import java.util.Arrays;
public class Fill2DArrayWithFill {
public static void main(String[] args) {
int[][] array = new int[3][3];
for (int i = 0; i < array.length; i++) {
Arrays.fill(array[i], i + 1); // Filling each row with the same value
}
for (int[] row : array) {
for (int value : row) {
System.out.print(value + " ");
}
System.out.println();
}
}
}
Output:
1 1 1
2 2 2
3 3 3
In this example, we use Arrays.fill()
to fill each row of the 2D array with the same value, which is determined by the row index plus one. This method is particularly useful when you want to initialize all elements of a row to the same value quickly. It simplifies the code and improves readability.
Method 3: Filling a 2D Array Using Stream API
If you’re using Java 8 or later, you can take advantage of the Stream API to fill a 2D array in a more functional programming style. This method can be particularly elegant and concise. Here’s how you can achieve that:
import java.util.stream.IntStream;
public class Fill2DArrayWithStreams {
public static void main(String[] args) {
int[][] array = IntStream.range(0, 3)
.mapToObj(i -> IntStream.range(0, 3).map(j -> i + j).toArray())
.toArray(int[][]::new);
for (int[] row : array) {
for (int value : row) {
System.out.print(value + " ");
}
System.out.println();
}
}
}
Output:
0 1 2
1 2 3
2 3 4
In this example, we use IntStream.range()
to create a stream of integers for both rows and columns. We then use map()
to fill the array based on the sum of the indices. Finally, we convert the stream back to a 2D array using toArray()
. This method not only looks clean but also leverages the power of Java’s functional programming capabilities.
Conclusion
Filling a 2D array in Java can be accomplished in various ways, each with its advantages. Whether you prefer the simplicity of nested loops, the efficiency of Arrays.fill()
, or the elegance of the Stream API, you now have a toolkit of methods at your disposal. Understanding how to manipulate 2D arrays is essential for any Java developer, as it opens the door to more complex data structures and algorithms. Take the time to experiment with these methods, and you’ll find that working with 2D arrays becomes second nature.
FAQ
-
What is a 2D array in Java?
A 2D array is an array of arrays, allowing you to store data in a grid-like structure with rows and columns. -
How do I declare a 2D array in Java?
You can declare a 2D array using the syntax:dataType[][] arrayName = new dataType[rows][columns];
. -
Can I fill a 2D array with random values in Java?
Yes, you can use theRandom
class to generate random values and fill the array using loops. -
What is the advantage of using the Stream API for filling arrays?
The Stream API allows for a more concise and functional approach to filling arrays, making the code cleaner and often easier to read. -
Are there any performance differences between these methods?
Performance can vary based on the method used and the size of the array, but for most practical purposes, the differences are negligible.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook