How to Create a Subarray in Java
- Using Arrays.copyOfRange Method
- Using a Loop to Create a Subarray
- Using Stream API for a Subarray
- Conclusion
- FAQ
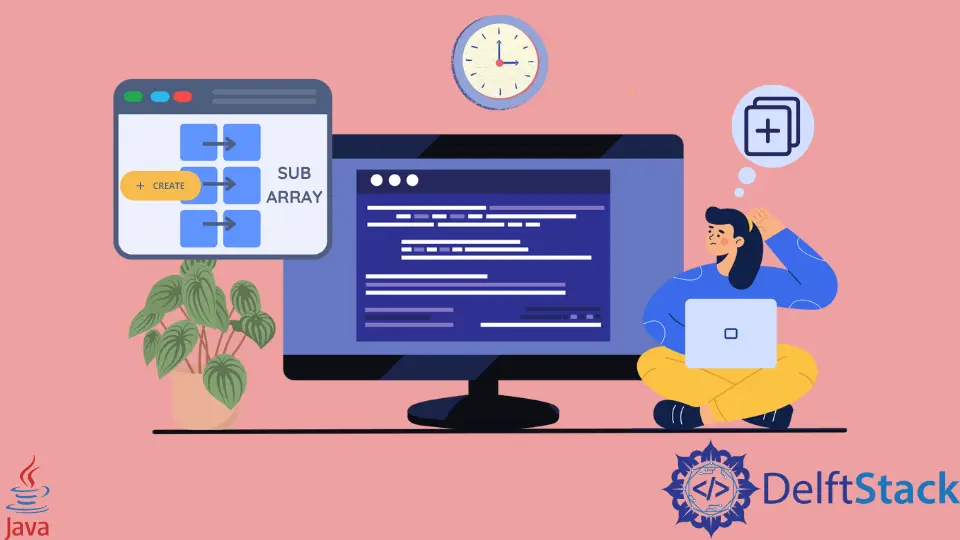
Creating a subarray in Java can be a crucial skill for developers who need to manipulate arrays efficiently. Whether you’re working on an algorithm that requires slicing an array or simply need to extract a portion of data, knowing how to create a subarray is essential.
In this tutorial, we will explore various methods to create a subarray from another array in Java. We will discuss the syntax, provide clear examples, and explain each method in detail. By the end of this article, you will have a solid understanding of how to create subarrays in Java, allowing you to implement this functionality in your own projects seamlessly.
Using Arrays.copyOfRange Method
One of the simplest and most effective ways to create a subarray in Java is by using the Arrays.copyOfRange
method. This method allows you to specify the original array, the starting index, and the ending index, making it easy to extract a specific portion of the array.
Here’s how you can use this method:
import java.util.Arrays;
public class SubarrayExample {
public static void main(String[] args) {
int[] originalArray = {1, 2, 3, 4, 5, 6, 7, 8, 9};
int start = 2;
int end = 5;
int[] subArray = Arrays.copyOfRange(originalArray, start, end);
System.out.println(Arrays.toString(subArray));
}
}
Output:
[3, 4, 5]
The Arrays.copyOfRange
method is straightforward and efficient. In this example, we start from index 2 and end at index 5 of the original array. The result is a new array containing elements from index 2 to index 4 (the end index is exclusive). This method is particularly useful when you need to create a subarray without writing additional loops or complex logic.
Using a Loop to Create a Subarray
Another method to create a subarray is by using a simple loop. This approach gives you more control over the elements you want to include in your subarray. It’s especially beneficial when you need to apply additional logic while copying elements.
Here’s how you can implement this method:
public class SubarrayWithLoop {
public static void main(String[] args) {
int[] originalArray = {1, 2, 3, 4, 5, 6, 7, 8, 9};
int start = 3;
int end = 7;
int[] subArray = new int[end - start];
for (int i = start; i < end; i++) {
subArray[i - start] = originalArray[i];
}
System.out.println(Arrays.toString(subArray));
}
}
Output:
[4, 5, 6, 7]
In this example, we define a new array subArray
with a size equal to the number of elements we want to copy. The loop iterates from the starting index to the ending index, copying each element from the original array to the new subarray. This method is flexible, allowing you to add conditions or transformations on the elements as you copy them.
Using Stream API for a Subarray
If you’re working with Java 8 or later, you can utilize the Stream API to create a subarray. This method is more concise and leverages functional programming features, making your code cleaner and more readable.
Here’s how you can achieve this:
import java.util.Arrays;
import java.util.stream.IntStream;
public class SubarrayWithStream {
public static void main(String[] args) {
int[] originalArray = {1, 2, 3, 4, 5, 6, 7, 8, 9};
int start = 1;
int end = 6;
int[] subArray = IntStream.range(start, end)
.map(i -> originalArray[i])
.toArray();
System.out.println(Arrays.toString(subArray));
}
}
Output:
[2, 3, 4, 5, 6]
Using the Stream API, we first create a range of indices from the start to the end. We then map each index to its corresponding value in the original array. Finally, we collect the results into a new array. This method is not only elegant but also allows for easy modifications, like filtering or transforming elements during the extraction process.
Conclusion
Creating a subarray in Java is a fundamental skill that can significantly enhance your array manipulation capabilities. Whether you choose to use Arrays.copyOfRange
, a loop, or the Stream API, each method has its own advantages. By understanding these techniques, you can choose the one that best fits your specific needs. With practice, you will find that creating subarrays becomes a seamless part of your programming toolkit, allowing you to write more efficient and effective Java code.
FAQ
-
What is a subarray in Java?
A subarray is a contiguous portion of an array, defined by a starting and ending index. -
Can I create a subarray from a multidimensional array?
Yes, you can create subarrays from multidimensional arrays, but the approach may vary based on the dimensions. -
Is there a performance difference between using Arrays.copyOfRange and a loop?
Generally,Arrays.copyOfRange
is optimized for performance, but using a loop allows for more customization. -
Can I create a subarray of an array of objects in Java?
Absolutely! The same methods apply to arrays of objects, just ensure you handle the object types correctly. -
Are there any limitations to creating subarrays in Java?
The primary limitation is the bounds of the original array; you cannot create a subarray that exceeds the original array’s size.