How to Copy Array in Java
-
Copy an Array to Another Using
Arrays.copyOf()
in Java -
Copy an Array to Another Using
System.arraycopy()
in Java -
Copy a Two Dimensional Array Into Another Using
clone()
in Java
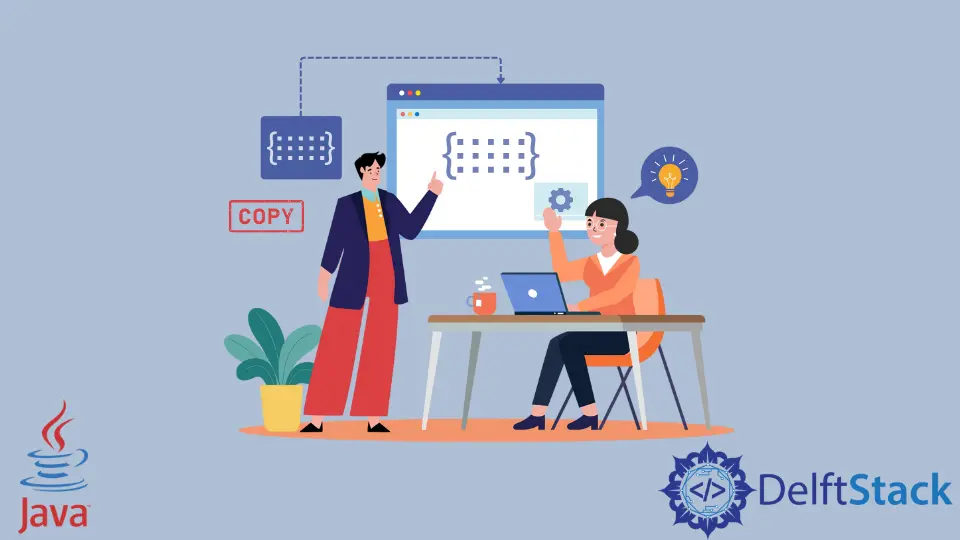
This tutorial introduces several methods to copy an array to another array in Java. We can use the manual approach with loops to achieve this, but we would like not to use that method for the sake of simplicity, and we do not want to reinvent the wheel.
Copy an Array to Another Using Arrays.copyOf()
in Java
We can use the copyOf()
method of the Arrays
class that copies the specified array to a new array. copyOf()
takes two arguments; the first is the array to copy, and the second is the length of the new array.
We copy array1
to array2
in the below example. The length of the new array is equal to the array1
. If the new array has a size greater than the old array, then every empty index will be filled with a zero. We used a loop to print the new array’s items and their indexes.
import java.util.Arrays;
public class CopyArray {
public static void main(String[] args) {
int[] array1 = new int[] {2, 4, 6, 8, 10};
int[] array2 = Arrays.copyOf(array1, array1.length);
for (int i = 0; i < array2.length; i++) {
System.out.println("array2 position " + i + ": " + array2[i]);
}
}
}
Output:
array2 position 0: 2
array2 position 1: 4
array2 position 2: 6
array2 position 3: 8
array2 position 4: 10
Copy an Array to Another Using System.arraycopy()
in Java
System.arraycopy()
can be useful when we want to create a new array with the sub-items of the old array, as it copies the array items from a specified position of the old array to the position of the new array.
System.arraycopy()
takes at least four arguments, that are, the array to copy array1
, starting position of array1
, new array array2
, the starting position of array2
, and the number of items to be copied to array2
. The output shows the items in array2
.
public class CopyArray {
public static void main(String[] args) {
String[] array1 = new String[] {"Adam", "Claire", "Dave", "Greg", "Halsey", "Jane", "Kylie"};
String[] array2 = new String[array1.length];
System.arraycopy(array1, 0, array2, 0, array1.length);
for (int i = 0; i < array2.length; i++) {
System.out.println("array2 position " + i + ": " + array2[i]);
}
}
}
Output:
array2 position 0: Adam
array2 position 1: Claire
array2 position 2: Dave
array2 position 3: Greg
array2 position 4: Halsey
array2 position 5: Jane
array2 position 6: Kylie
Copy a Two Dimensional Array Into Another Using clone()
in Java
The last method to copy an array in Java is clone()
that returns a new array with the copied array items. In this example, we use a two-dimensional array array1
that has eight elements. We use array1.clone()
to copy the array and two loops to print the new array array2
elements.
public class CopyArray {
public static void main(String[] args) {
int[][] array1 = {{10, 20}, {30, 40}, {50, 60}, {70, 80}};
int[][] array2 = array1.clone();
for (int i = 0; i < array2.length; i++) {
for (int j = 0; j < array2[i].length; j++) {
System.out.println("array2 position [" + i + "][" + j + "] : " + array2[i][j]);
}
}
}
}
Output:
array2 position [0][0] : 10
array2 position [0][1] : 20
array2 position [1][0] : 30
array2 position [1][1] : 40
array2 position [2][0] : 50
array2 position [2][1] : 60
array2 position [3][0] : 70
array2 position [3][1] : 80
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn