How to Compare Characters in Java
-
Compare Characters Using
Character.compare()
in Java -
Compare Characters Using
==
in Java -
Compare Characters Using
String.matches()
in Java
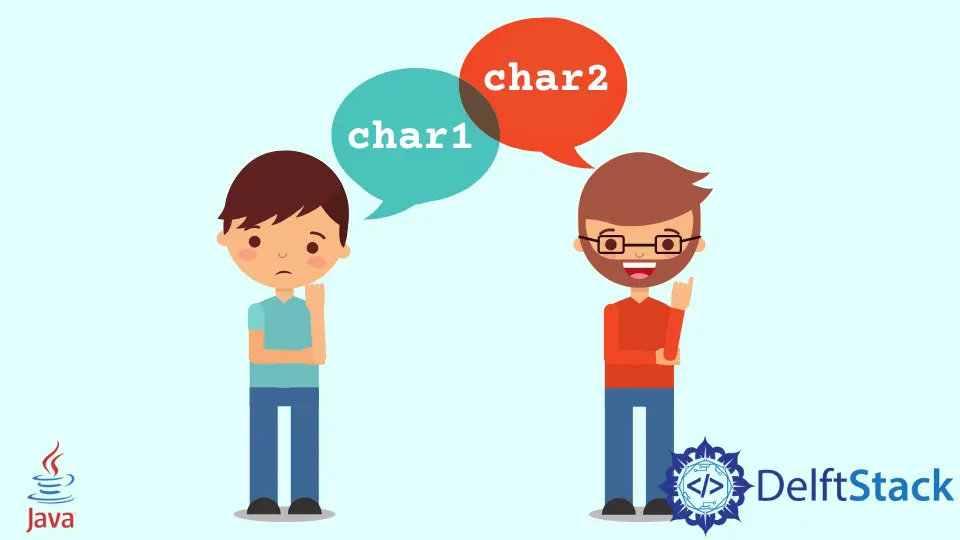
This article will introduce how to compare characters in Java.
Compare Characters Using Character.compare()
in Java
We can compare two characters using the compare()
method of the Character
class in Java. It takes two characters as the arguments and returns zero if both the characters are equal, a negative value if the first character is smaller than the second character, and a positive number if the first character is greater than the second one.
Below, two characters, char1
and char2
, are compared using Character.compare()
, and the return value is checked if it is less than, more than, or equal to zero. We can see that the output of the example shows that both the characters are identical.
public class CompareChar {
public static void main(String[] args) {
char char1 = 'z';
char char2 = 'z';
if (Character.compare(char1, char2) > 0) {
System.out.println(char1 + " is greater");
} else if (Character.compare(char1, char2) < 0) {
System.out.println(char1 + " is less than " + char2);
} else if (Character.compare(char1, char2) == 0) {
System.out.println(char1 + " and " + char2 + " are equal");
} else {
System.out.println(char1 + " and " + char2 + " are invalid characters");
}
}
}
Output:
z and z are equal
Compare Characters Using ==
in Java
We can use double equals to compare characters without using any long methods. But there is minimal flexibility as it only tells if the characters are the same or not.
public class CompareChar {
public static void main(String[] args) {
char char1 = 'a';
char char2 = 'b';
char char3 = 'a';
if (char1 == char2) {
System.out.println("Char1 and Char2 are equal");
} else {
System.out.println("Char1 and Char2 are not equal");
}
if (char1 == char3) {
System.out.println("Char1 and Char3 are equal");
} else {
System.out.println("Char1 and Char3 are not equal");
}
}
}
Output:
Char1 and Char2 are not equal
Char1 and Char3 are equal
Compare Characters Using String.matches()
in Java
In this method of comparing characters in Java, we use regular expressions to check if the character is a lower case alphabet or not. We will use the matched()
method that can be used with strings. We have a character that needs to be converted to a string using the matches()
method. Thus, in the example below, we use Character.toString(char1)
and then the regex method.
public class CompareChar {
public static void main(String[] args) {
char char1 = 'a';
if (Character.toString(char1).matches("[a-z?]")) {
System.out.println("The character matches");
} else {
System.out.println("The character does not match");
}
}
}
Output:
The character matches
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn